- A simple webserver is nothing but a program that listens for requests
on a port and serves up requested files.
- Servlet-capable webservers also run Java servlets on request.
- A simple file-serving webserver can be written in less than 100
lines of code.
- A servlet-capable webserver needs more because it needs to upload
servlets, maintain servlet contexts and provide some additional API's.
- The Miniature Java
Webserver, based on the ACME webserver,
is an attempt to create a small webserver capable of
handling Java servlets.
- The webserver below is a modification of TJWS, further simplified
for CS-2113 students.
Getting your first webpage served
- Step 1: Login. Use your username/password combination to
login to the Remote Unix Server. If you've forgotten,
the instructions are here
- Step 2: Make a directory for this part of the course.
From your home directory, make a directory called
cs2113:
cd
mkdir cs2113
- Step 3: Download two jar-files into
cs2113:
Download SWS.jar
and servlet.jar.
Ultimately, the files SWS.jar
and servlet.jar
need to be in the
cs2113
directory off of your home directory on the Unix machine.
- Step 4:
Enter the
cs2113
directory and
unpack the SWS.jar file:
cd cs2113
jar -xvf SWS.jar
This should create a subdirectory called SimpleWebServerJar.
There should be another jar file called simplewebserver.jar
inside.
- Do NOT unpack servlet.jar.
- Type pwd
to know the full path into this directory.
pwd
- Step 4: modify your .bashrc. Go back to your
home directory and create (or edit) your
.bashrc file
(you will end up creating one if there isn't one)
to add these jar files to your
CLASSPATH
variable.
To get to the home directory and create (or edit), do this
at the Terminal:
cd
pico .bashrc
For example, if your username is
batman,
add the following lines inside the
pico editor:
export CLASSPATH=$CLASSPATH:/home/seas/batman/cs2113/servlet.jar
export CLASSPATH=$CLASSPATH:/home/seas/batman/cs2113/SimpleWebServerJar/simplewebserver.jar
export CLASSPATH=$CLASSPATH:.
Now save the file.
NOTE: the path to
cs2113
may change from year to year, depending on how the Unix system
is configured. For example, in the past, student home directories
have had paths like
/home/seas/students/batman/cs2113.
You'll know by using
pwd
to "print the working directory":
cd
pwd
- Step 5: "Source" the .bashrc file:
cd
source .bashrc
And then check that this worked by typing this in the Terminal:
echo $CLASSPATH
You should see the two jar files in your classpath (among other things, possibly).
- Important:
You will need to edit your
.bashrc
just once. Future logins will execute this and your CLASSPATH
should be set. You can always check this by echo-ing CLASSPATH
to verify.
IF, however, it does not work, follow these steps:
- Create or edit in your home directory a file called
.profile
- Put the same EXPORT lines into this file as you did with
.bashrc
- Every time you open a Terminal, "source" the file as
cd
source .profile
The CLASSPATH needs to be properly set for the webserver to launch.
- Step 6: Edit the webserver's port number. Get into the
SimpleWebServerJar directory and edit the cmdparams
file:
cd
cs cs2113
cd SimpleWebServerJar
pico cmdparams
Important: Change the port number (next to
the -p option) to
your assigned port number.
For example, if your assigned port number
is 40013, then change the line to:
-a aliases.properties -p 40013 -l -c cgi-bin -s servlet.properties
- Step 7: Create a test HTML file. Go to the
webroot sub-directory and edit the index.html
file to include your name.
- Step 8: Run the webserver. Go to the
SimpleWebServerJar directory and run the webserver:
cd
cd cs2113
cd SimpleWebServerJar
java Serve
You should see something like (if 40013 is your assigned port):
STARTING webserver with logfile=SWS.log
Current directory: /home/batman/SimpleWebServerJar
Commandline arguments:
-a aliases.properties
-p 40013
-l -c
-c cgi-bin
-s servlet.properties
Attempting a read of className=HelloWorld from directory /home/batman/SimpleWebServerJar/servlets/
=> Instance of className=/home/batman/SimpleWebServerJar/servlets/HelloWorld created with urlPat=/servlets/
Press "q" , for gracefully stopping the server WebServer at port 9013 is ready.
As indicated, you can (later) enter "q" to quit.
- Trouble-shooting Step 8:
- Occasionally you may find that the
Serve
program runs but quits on its own, returning to the commandline.
- What means is that Java is able to find the program
(so steps 1-7 worked) but that the program cannot capture
your assigned port number.
- This means that your assigned port number is being used,
mostly likely by your previous attempt.
- To get rid of this:
- Start by discovering whether a previous attempt of yours
at running
java Serve
is still active by typing the following at the commandline:
ps -u
This will list all active processes (as rows) where the
first column is the username and second is the PID (process ID).
- One of the columns towards the right is the COMMAND column.
This is where you will see
java Serve
if that's active.
- The second column is the PID, which is typically a 6-digit number.
- If you see that it's active, you can get rid of it by typing
the following at the Terminal
kill <PID>
For example, if the PID for
java Serve
row is 164753, you would type
kill 164753
See this picture for example:
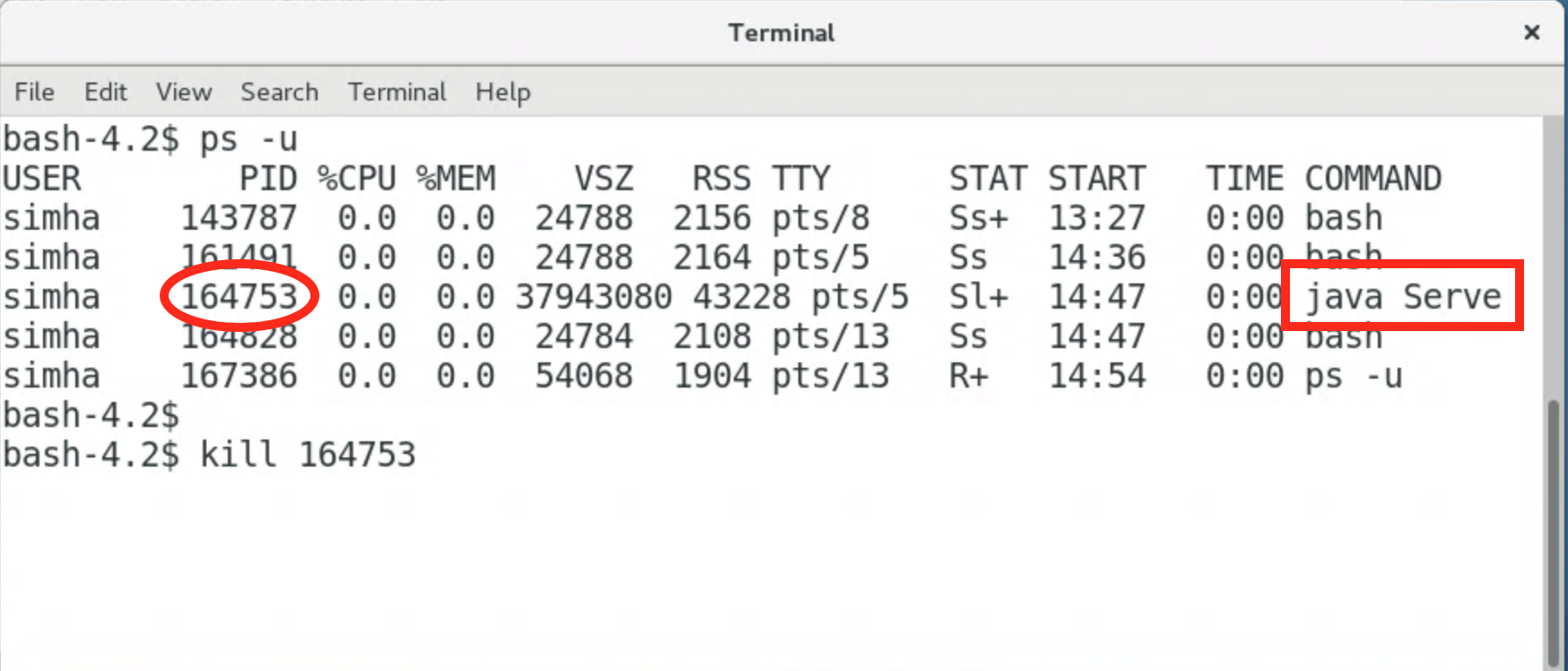
- Then, run
java Serve
again.
- Step 9: Use it. Access the index.html file
from any browser. For example, if your assigned port is 40013,
then type the URL:
http://unix.seas.gwu.edu:40013
This should bring up your index.html file.
- Step 10: Do something more complicated.
For example, create a subdirectory with some files and link to them.
Put some form elements in the files.
- Step 11: Examine the rest of the folder.
- The webroot directory is the home for all documents.
Thus the index.html file in there is what is uploaded
when you enter the URL of the webserver (e.g.,
http://unix.seas.gwu.edu:40013) without a file name.
- The servlets directory contains servlet code (source
and class files). For example, the HelloWorld servlet
is in this directory.
- To execute HelloWorld, you would enter the
URL
http://unix.seas.gwu.edu:port/servlets/HelloWorld
in a browser. The browser can run anywhere.
- The locations of servlets are specified in the file
SimpleWebServerJar/servlet.properties, which the
webserver reads on startup.
- Important: when installing a new servlet, you must
add the servlet's path in the servlet.properties file and
re-start the webserver (else it won't be loaded).
- The directory servlets/examples contains the
code for the servlet examples in Module 15.
- The webroot/examples directory contains webpages that
are used for the servlet examples in Module 15.
Getting your first servlet to run
Parameters
This section shows you how to run the TestForm servlet
that prints out form parameters:
- Step 1: Locate the file TestForm.java in
the servlets/examples directory.
Does the code have port numbers buried in it? If so, you'll
have to change the port number to your assigned port number.
- Step 2: Compile the servlet.
- Step 3: Make sure the servlet.properties file
contains the correct path to this servlet. The path should be:
servlet.TestForm.code=/servlets/examples/TestForm
- Step 4: Locate the HTML file testform.html
in the webroot/examples directory. This is the file to
access.
- Step 5: Important: Edit
testform.html
to change the form URL in testform.html
so that it uses your assigned port number.
- Step 6: Run the webserver. Check that the servlet
got loaded by the webserver and that the webserver is listening
at your assigned port.
- Step 7: Access the file testform.html from
a browser
http://unix.seas.gwu.edu:40013/examples/testform.html
- Step 8: Modify the HTML to contain a hidden parameter
and run the servlet again to make sure that hidden parameters are
printed out.
- Important: You will eventually need to edit all the
HTML files in the
webroot/examples directory.
to use your assigned port number.
Session tracking
This section shows you how to run the PageCount servlet
that prints out form parameters:
- Step 1: Modify the servlet source to use your assigned
port number. This is in the line that writes out the
"form" action command in HTML. Then compile the servlet.
- Step 2: Modify the
webroot/examples/pagecount.html
file to use your port number.
- Step 3: Run the webserver and access
pagecount.html.