Module 1: Your first applet
A simple "helloworld" applet
Follow these steps in getting a simple "helloworld" applet to work:
- Step 1: Install Java on your PC and learn how to
compile a Java program. For this purpose, you can either use
a sophisticated environment such as Forte or simply compile at the
command-line (DOS prompt).
See the Getting Started page for instructions.
- Step 2: Create a directory (folder) for the Java
program you will be writing in Step 3.
- Step 3: Write the "helloworld" Java program in
a file called HelloWorld.java. Here is the source:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
You can create this file using
any editor or environment. For example, this is what it would
look like in WordPad:
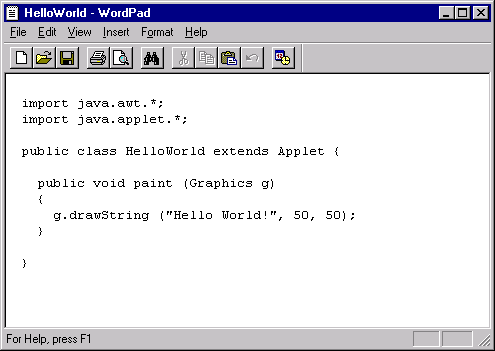
- Step 4: Compile the program using any of the options
outlined in the
Getting Started page.
- Step 5: Create an HTML file in the same directory
that is to be uploaded
into the web browser and that refers to the applet. Here's what
the HTML file should contain:
<HTML>
<BODY>
<applet code="HelloWorld.class" width=300 height=200>
</applet>
</body>
</html>
This file can be created in any editor.
For example, this is what it would look like in WordPad:
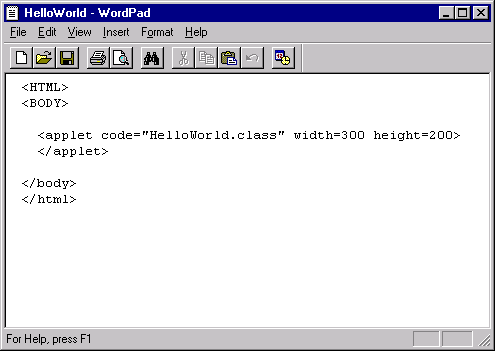
- Step 6: Load the HTML file in a web browser.
If the browser is enabled for Java, you should see the applet
running. For example, here's what it looks like in Internet
Explorer:
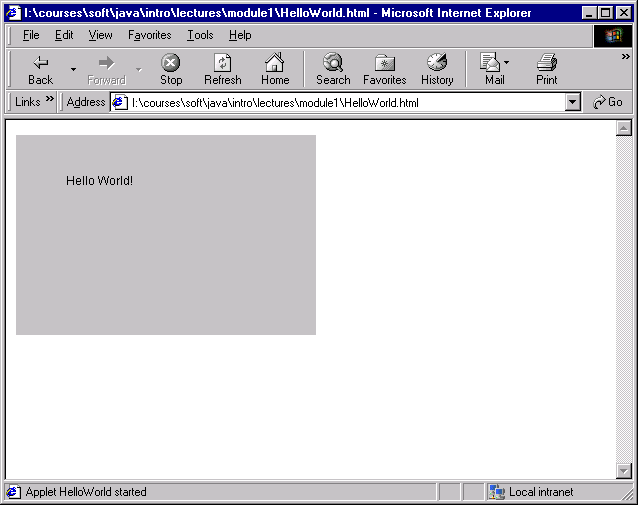
Troubleshooting (It didn't work):
- Is your browser enabled for Java? By default, both Netscape
and Internet Explorer enable Java applets.
For Internet Explorer, click on the "Tools" menu and go down
to "Internet Options". This brings up a new window with tabs
like "General", "Security" and so on. Go to the tab called
"Advanced". You should see a long list of checkboxes with
labels next to them. One of these will be "Java". Make sure
that the box is checked.
- Did you read a local file or did you access a file you placed
on a website?
- If you are reading a local file, you need to go to the
"File" menu of your browser, then to "open" and then open
a local file. The HTML file and the Java file
(HelloWorld.class) need to be in the same directory.
- If you uploaded your HTML to a website, check to make sure
that they are downloadable (i.e., that permissions have been set right).
- Note: a link to the applet itself
Other ways of running applets:
- You can run applets from most development environments.
For example, JGrasp lets you run applets from within JGrasp
(from the "Run" menu, go to "Run as Applet"). For this purpose
you will not need the HTML file.
- You can run applets using the standalone appletviewer tool
from SUN. This tool comes with the JDK and can be run from the commandline.
Explaining the "helloworld" applet
So far, we have written, compiled and run a Java program without
necessarily understanding what we are doing. That's OK for now - it's
important to get going with something.
Let's take a look at the program itself:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
What do we notice?
- There appear to be words such as import and paint.
- There are other symbols such as { and ;
(semicolon).
- There are two kinds of parentheses, curly ones (braces) and round ones
(the ones we usually call parentheses or brackets).
- There are numbers such as 50.
- Some lines are indented, others are not.
First, let's understand reserved words and identifiers:
- These roughly correspond to "words".
- Reserved "words" are words that have special meaning in the
Java language. They can be used for only one purpose, although some
can be used many times in a single program.
- Let's identify the reserved words in the HelloWorld program (in
boldface below):
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
- The reserved words in this particular program are:
import, public, class, extends, public and void.
- These are not the only reserved words in the Java language.
Here's a complete list:
abstract,
boolean, break, byte,
case, catch, char, class, const, continue,
default, do, double,
else, extends,
false, final, finally, float, for,
goto,
if, implements, import, instanceof, int, interface,
long,
native, new, null,
package, private, protected, public,
return,
short, static, super, switch, synchronized,
this, throw, throws, transient, true, try,
void, volatile, while
- While there's no need to memorize this list, it's worth reading
through a couple of times or coming back to it.
- Identifiers are also "words" but they are not
part of the language and are instead "invented" by ther
programmer to name items in the program.
- Example: the identifer HelloWorld is used to name the
program above.
- Another identifier above is the one-letter identifier
g that's next to drawString.
- Now, there are two kinds of identifiers: names that you
invent completely (such as HelloWorld above)
and names that have been invented by Java but aren't reserved words.
Let's explain the latter:
- There are "names" above such as Applet, paint, Graphics
and drawString that are names of things in the
Java library.
- The Java library is not part of the Java language
per se, but is often considered inseparable because they come
with the Java toolkit. It's sometimes called "The API"
(for Application Programmer Interface).
- When you create class'es of your own
and methods of your own, you'll give them names.
Thus, because the Java library contains classes, those
must have names, which in the above program are:
Applet, paint, Graphics
and drawString.
- The import statements at the top, in fact,
tell you which parts of the library are being "imported"
for use: the java.awt package and the
java.applet package.
- There are some differences between reserved words and
identifiers worth noting:
- You may not use reserved words as your own identifiers.
For example, if you renamed your program instanceof (a
reserved word), you would get a compilation error:
import java.awt.*;
import java.applet.*;
public class instanceof extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
- You may use Java library identifiers, but at some risk:
- For example, let's use paint as follows:
import java.awt.*;
import java.applet.*;
public class paint extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
Here, as long as you name the file paint.java (and
change the applet reference in the HTML file accordingly),
it'll work.
- In the above case, we used a Java library identifier
(paint) in place of our own one (HelloWorld).
- However, replacing a Java library identifier with one
of our own won't work. Consider this example:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends BlahBlah {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
Here, we replaced Applet with BlahBLah, a name
we just invented. The program won't compile because the compiler
looks around for a BlahBlah class and can't find it.
- For identifiers that we invent, we can use any combination
of letters and digits and the underscore character as long
as the identifier starts with a letter.
- For example, let's replace the identifier g above
with gee_whiz_123X
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics gee_whiz_123X)
{
gee_whiz_123X.drawString ("Hello World!", 50, 50);
}
}
This works fine because (as we'll learn) the parameter g
is really a name given by us.
- It's not recommended that you re-name variables as above;
that was only for illustration.
Next, let's go over whitespace:
- As you might guess, whitespace refers to blanks, tabs
and "new lines" between characters in the program.
- You must have at least some whitespace (at least one blank)
between successive "words", that is, between two reserved words,
or a reserved word and identifier, or between two identifiers.
- For example, we couldn't remove the blank between extends
and Applet above:
import java.awt.*;
import java.applet.*;
public class HelloWorld extendsApplet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
This will cause a compilation error.
- There is an exception: if a Java symbol
is between reserved words, there's no need for whitespace.
For example, there is a period between the identifiers
g and drawString.
- Where whitespace is allowed, you can use any amount of
whitespace.
For example, let's place a whole bunch of whitespace between
void and paint above:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void
paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
This works fine, but looks strange.
- There are conventions to using whitespace, which
are best learned by looking at example programs.
Finally, let's point out a few more things:
- Most of your initial programming will be done inside
the innermost set of braces:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
- It is not necessary to understand the other stuff to get
started with useful and interesting programming.
- The phrase "Hello World!" encased in double-quotes.
is called a string.
- The numbers 50 and 50 are coordinates using the top-left
corner as the origin. Thus, the command drawString
draws the string "Hello World!" starting at a point
50 units below the top-left and 50 units to the right of it.
Modifying the "helloworld" applet
Let's make a couple of changes to the helloworld applet
and put the changes into a new file called
HelloWorldBlah.java:
import java.awt.*;
import java.applet.*;
public class HelloWorldBlah extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
g.drawString ("Blah, blah, blah", 50, 80);
g.drawString (".... and more blah, blah", 90, 150);
}
}
Note:
- We are writing out three strings in the program:
- The string "Hello World!" starting at (50, 50), that we had earlier.
- The string "Blah, blah, blah" starting at (50, 80). Thus,
this is at the same X position, but deeper down.
- The string ".... and more blah, blah" at (90, 150) which
is more to the right and further down.
- Remember to compile the program.
- Important: the class name
(HelloWorldBlah) has to be the same as the first
part of the file name (HelloWorldBlah.java).
- We need to create a new HTML file or change the reference in
the earlier file:
<HTML>
<BODY bgcolor="#FFFFFF">
<applet code="HelloWorldBlah.class" width=300 height=200>
</applet>
</body>
</html>
(Note: we've explicitly set the background color of the page to white)
- Load the new HTML file into a browser.
- The resulting applet looks like:
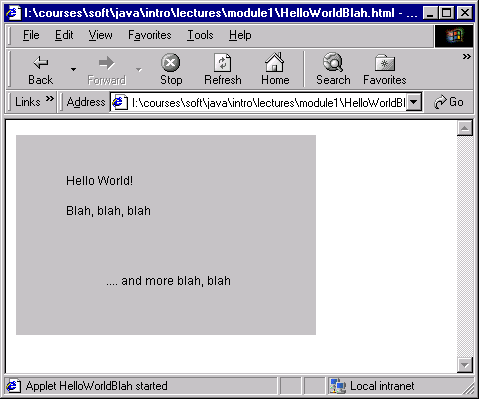
- Note: a link to the applet itself
Thus, we've learned how to do some programming and, more importantly,
the process of getting the modified applet to execute.
In-Class Exercise:
Write an applet that displays two intersecting words, one
vertical and one horizontal, as in:
J
C L A S S
V
A
Comments
Comments are text that we can place in a program
that don't affect the program at all. For example:
import java.awt.*;
import java.applet.*;
// This program is a modification of the HelloWorld applet
public class HelloWorldBlah extends Applet {
public void paint (Graphics g)
{
// Our original helloworld string:
g.drawString ("Hello World!", 50, 50);
// A new string that's below it:
g.drawString ("Blah, blah, blah", 50, 80);
// A third string that's more to the left and much below:
g.drawString (".... and more blah, blah", 90, 150);
}
}
Note:
- The comments above start with a
double-forwardslash "//"
- Such a comment causes the compiler to ignore everything
from the "//" all the way up to the end of the line.
- You can start a comment after some valid Java commands, as in:
import java.awt.*;
import java.applet.*;
// This program is a modification of the HelloWorld applet.
public class HelloWorldBlah extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50); // Our original helloworld string.
g.drawString ("Blah, blah, blah", 50, 80); // A new string that's below it.
// A third string that's more to the left and much below:
g.drawString (".... and more blah, blah", 90, 150);
}
}
- You can place any number of comments in a file, as in:
import java.awt.*;
import java.applet.*;
// This program is a modification of the HelloWorld applet
public class HelloWorldBlah extends Applet {
public void paint (Graphics g)
{
// Our original helloworld string:
g.drawString ("Hello World!", 50, 50);
// A new string that's below it:
g.drawString ("Blah, blah, blah", 50, 80);
// A third string that's more to the left and much below:
g.drawString (".... and more blah, blah", 90, 150);
// Another comment.
// Yet another comment.
// And one more.
// ... yada, yada, yada.
}
}
// And for good measure, one last comment here.
- Comments serve to explain programs to readers.
- A good question to ask is: if I'm writing my programs, why
do I need to explain them to me?
- First, you most often write programs that are read by others.
- Even if you are the only one reading your programs, you might
forget why you did something. A comment helps explain.
- Commenting is particularly important when programming in
groups, which is how most software is written.
- A program without comments is like a phonebook that has
only phone-numbers (the useful part) and no names next to the
phone numbers. You might remember phone-numbers initially
("Hmm, I know Joe's number is right at the bottom"), but
you'll eventually forget.
- There are other kinds of comments in Java:
import java.awt.*;
import java.applet.*;
/**
* This program is a modification of the HelloWorld applet.
* This is a Javadoc comment.
*/
public class HelloWorldBlah extends Applet {
public void paint (Graphics g)
{
/* Our original helloworld string.*/
g.drawString ("Hello World!", 50, 50);
// A new string that's below it.
g.drawString ("Blah, blah, blah", 50, 80);
/* A third string that's more to the
left and much below: */
g.drawString (".... and more blah, blah", 90, 150);
}
}
- There are C-style comments that begin with /*
and end with */ and can span multiple lines.
These are rarely used in Java programs.
- There are javadoc comments that begin with
/**, end with */ and have each line
beginning with *. These are used with the
javadoc standalone utility to generate
documentation for large programs.
- For the most part, we'll use the regular "//"
comments.
In-Class Exercise:
The following program will not compile. Why? And what kind of
errors are identified by the compiler?
public class HelloWorld extends Applet {
//
Comment1
public void paint (Graphics g)
{
// Comment 2 // Comment 3
g.drawString ("Hello World!", 50, 50);
}
} // Comment 4
Troubleshooting: compiler errors
First, let's distinguish between three kinds of errors:
- Compiler errors:
- These are the result of not typing in the program correctly.
- Example: suppose we forgot the semi-colon at the end of
a statement as in:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
// Deliberately removed the semicolon below:
g.drawString ("Hello World!", 50, 50)
}
}
- This would result in compiler error, something like:
HelloWorld.java:9: ';' expected
g.drawString ("Hello World!", 50, 50)
^
1 error
- Compiler errors arise when a program's structure is
not correct according to Java's syntactic rules.
- Runtime errors:
- These errors occur when a program compiles but won't
run properly because of something that went wrong during execution.
- For example, let's deliberately create one:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g = null;
g.drawString ("Hello World!", 50, 50);
}
}
- This will compile, but will create a runtime error.
- Unfortunately, it's hard to know when a runtime error
occurs when using applets because the browser will not display
anything special.
- Runtime errors are easily seen when running regular
Java programs (not applets).
- For example, consider
public class BadHelloWorld {
public static void main (String[] argv)
{
argv = null;
System.out.println ("Argument length: " + argv.length);
}
}
- This produces the run time error:
Exception in thread "main" java.lang.NullPointerException
at BadHelloWorld.main(BadHelloWorld.java:6)
- Logical errors:
- These errors are those found in programs that compile
perfectly but don't produce the desired effect.
- For example, the program below won't print anything
because the coordinates are too big:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 5000, 5000);
}
}
- The program compiles and executes fine but nothing
is displayed because the coordinates (5000, 5000) are way
off screen.
- Logical errors usually result from sloppiness in thinking
or from not knowing the effects of some actions.
It is initially difficult to tell exactly what the compiler
is complaining about when you get a compiler error.
Let's get accustomed to some compiler errors when the
syntax is incorrect:
In-Class Exercise:
What errors are reported by the compiler for this program?
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet // Left out this brace
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
In-Class Exercise:
What errors are reported by the compiler for this program?
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString (Hello World!", 50, 50); // Forgot the first double quote "
}
}
In-Class Exercise:
What errors are reported by the compiler for this program?
import java.awt.*;
// Removed the applet package import.
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
In-Class Exercise:
What errors are reported by the compiler for this program?
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
// Removed the ending brace
In-Class Exercise:
What errors are reported by the compiler for this program?
import java.awt.*;
import java.applet.*;
// Left out the "class" reserved word
public HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
Try these programs to see what happens.
In-Class Exercise:
import java.awt.*;
import java.applet.*;
// Left out the "public" reserved word
class HelloWorld extends Applet {
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
In-Class Exercise:
import java.awt.*;
import java.applet.*;
public class HelloWorld { // Did not extend Applet
public void paint (Graphics g)
{
g.drawString ("Hello World!", 50, 50);
}
}
In-Class Exercise:
import java.awt.*;
import java.applet.*;
public class HelloWorld extends Applet {
public void pain (Graphics g) // Misspelled "paint"
{
g.drawString ("Hello World!", 50, 50);
}
}