Environment variables
What is it?.
An environment variable is like a global
variable that's accessible to both the operating system and applications.
Some are already defined, such as the variable called PATH. Others
need to be defined by users.
The one that's needed for Java is
called CLASSPATH, which often needs to be defined or modified in both
Mac-OS and Windows.
When do you deal with environment variables?
You often need to either define some yourself or modify
existing ones by adding something to them.
Usually, environment variables contain strings
that identify directories (folders), and often
an environment variable will contain a group of such directories.
The PATH environment variable.
This is typically what the operating system uses to find executables.
It is especially useful when working at the command-line
(Mac or Windows), because the command-line tool (Terminal on Mac,
Command-prompt on Windows) will examine the PATH variable to
discover where an executable can be found.
The CLASSPATH environment variable.
This is used by Java (by both javac and java)
to find other Java code or libraries that you invoke from your code.
Note to Windows users: You are either using one of the Linux
options or using Windows-native (via Command-prompt or Powershell):
- Linux: If you are using Gitbash or WSL, the instructions
below will work provided:
- You perform editing from within Linux.
- You will be editing .bashrc instead of .profile.
- Use the nano editor at the command-line.
- You'll need to include the /c/ drive in the full path.
- Windows native: Read through the Mac/Linux instructions below for the sake
of understanding and then follow alternate instructions for Windows.
When you see Terminal (for Mac),you substitute Command-Prompt (or DOS-prompt) in Windows.
And your environment variables are set in a special tool via
Settings in Windows.
Topic 1: the PATH variable (Mac, Linux, Linux-on-Windows)
When does one need to set the PATH variable?
Java typically comes installed with a Mac and so there's
usually no need to set up this variable. Even when you install
Java, the installation procedure will correctly set this up.
However, you will need set this up if you install
an older verson of Java.
Important: z-shell vs. bash on Mac OS-X, and Linux
- Newer Mac's may have the z-shell as the default shell
in the Terminal.
- Older Mac's using bash require editing .profile
- Newer Mac's using z-shell require editing .zshrc
(How do you know which shell you have? Type echo $SHELL to find out.)
- On Linux, edit .bashrc
In the instructions below, we'll assume .profile but
you should substitute the appropriate initialization file.
How do you set the PATH variable?
You do this by editing the file .profile
(.zshrc if using z-shell) in the home directory.
- Type cd in the Terminal to get to the home directory.
- Start by bringing the .profile file into an editor.
Note: the file name starts with a period.
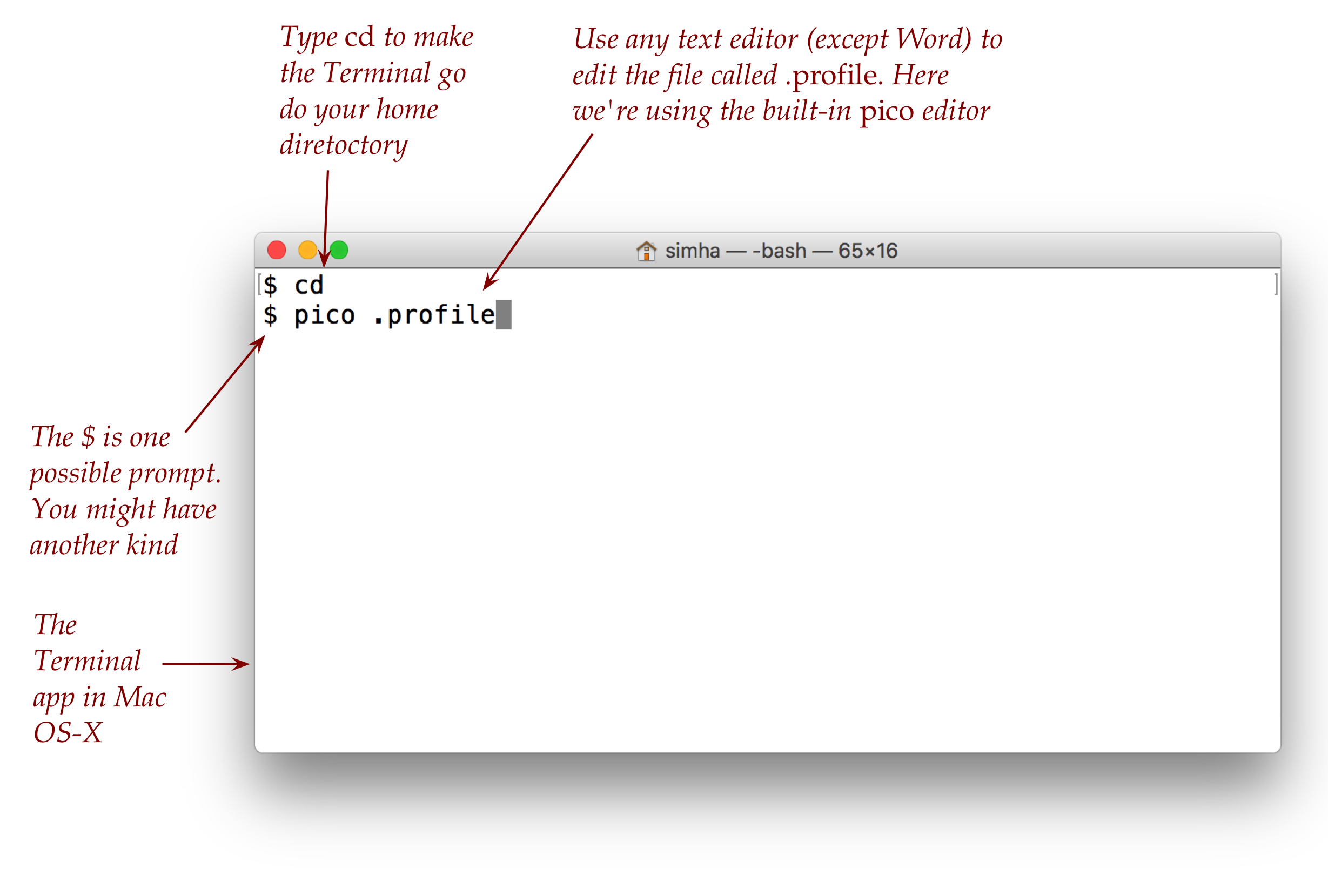
- For example, when using the pico editor, this is
what it will look like:
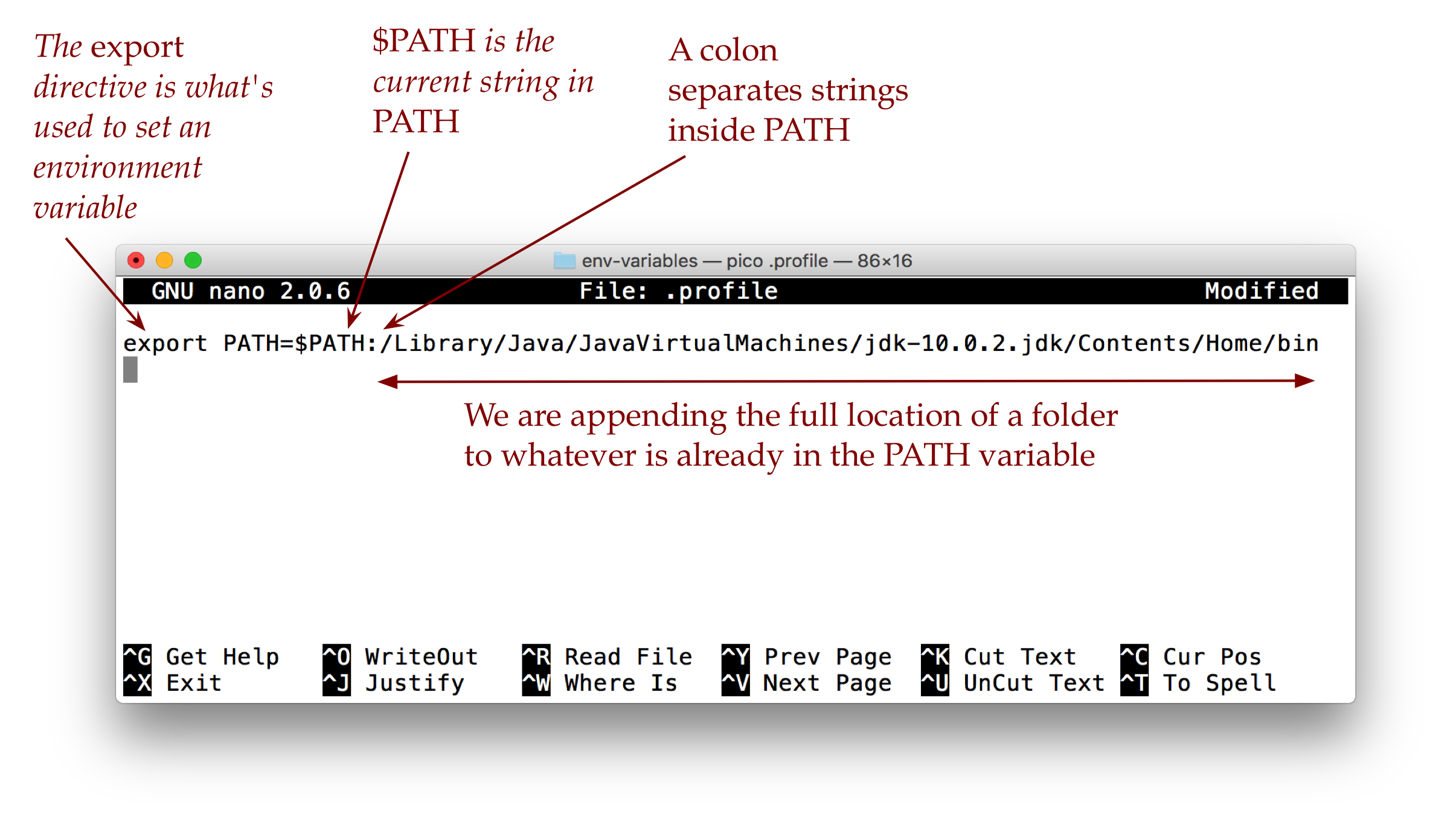
- We'll describe the above more compactly as:
export PATH=$PATH:/Library/Java/JavaVirtualMachines/jdk-10.0.2.jdk/Contents/Home/bin
- Just for demonstration, let's create our own new environment
variable, after our PATH variable.
export PATH=$PATH:/Library/Java/JavaVirtualMachines/jdk-10.0.2.jdk/Contents/Home/bin
export MY_ENV_VAR=Hello
- Now, exit the editor and save the file
called .profile.
If you do this in pico, you type control-x and
it will ask you whether you want to save (you type y).
- Next, get rid of the Terminal and open a new Terminal.
- At the command line, type echo $MY_ENV_VAR, for
example:
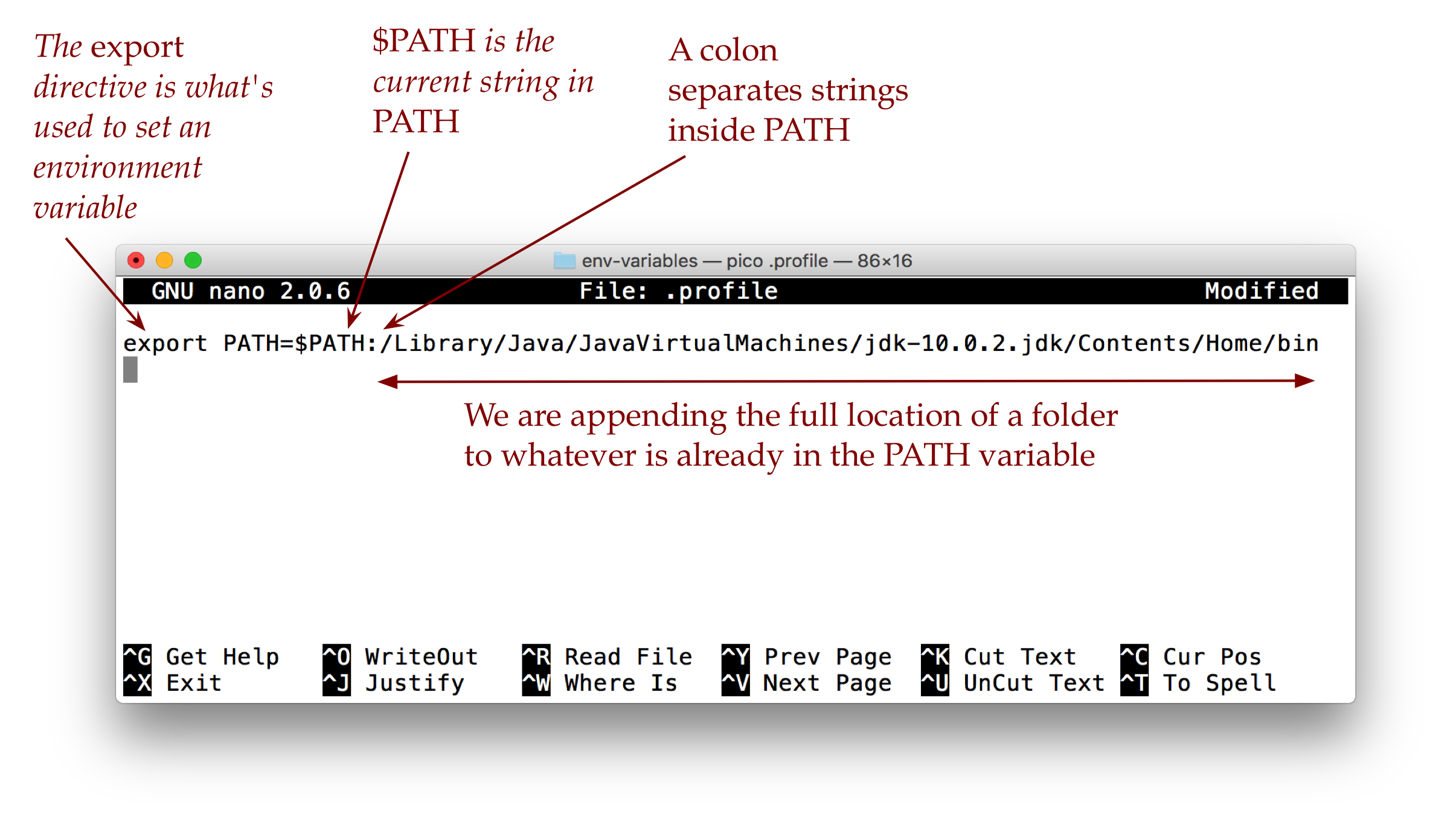
Thus we see that the contents of the environment variable called
MY_ENV_VAR is Hello and the Terminal was able
to access it.
- Type echo $PATH to confirm that your PATH
variable contains what you placed in it.
Multiple strings inside PATH.
Typically, a PATH variable contains many directories, each as a
string. The strings are separated by a colon, as in:
export PATH=$PATH:/Users/simha/Desktop/sillyapp:/Users/simha/Desktop/anotherapp/version3/bin/
Here, we've added the full directory as a string to each of two
programs (perhaps programs we've written):
- One is located in the /Users/simha/Desktop/sillyapp/ folder.
- Another is
located in /Users/simha/Desktop/anotherapp/version3/bin/.
So, when you type a command (program name) in Terminal, then Terminal
will examine the different directories in PATH in order,
and pick the first executable it finds to match the name.
This becomes an issue when there are two executables with
the same name, as in:
export PATH=$PATH:/Users/simha/Desktop/sillyapp1.0:/Users/simha/Desktop/sillyapp1.0:/Users/simha/Desktop/sillyapp2.0
Suppose both of these folders contain the
program sillyprogram, as in:
- /Users/simha/Desktop/sillyapp1.0/sillyprogram
- /Users/simha/Desktop/sillyapp2.0/sillyprogram
Suppose, each of these contain an executable called sillyprogram.
Then, when you type sillyprogram at the commandline,
the Terminal will execute the first one above (version 1.0).
Thus, if you have TWO versions of Java, put the version you want
executed first. You can do this as follows:
export PATH=/Library/Java/JavaVirtualMachines/jdk-10.0.2.jdk/Contents/Home/bin:$PATH
Here, we're placing Java-10 ahead of the installed Java by appending
the default (installed) contents of PATH to the folder for Java-10.
Use a period for the current directory.
Typically, we write programs in a directory and we want
the Terminal or Java to find these. The current directory is
represented by a period (.) and this must be included.
For example:
export PATH=$PATH:/Users/simha/Desktop/sillyapp1.0:/Users/simha/Desktop/sillyapp1.0:/Users/simha/Desktop/sillyapp2.0:.
Notice the period all by itself, after a colon, which says "current directory".
Topic 2: the CLASSPATH variable (Mac, Linux, Linux-on-Windows)
Let's start by explaining what an executable jar file is.
A plain jar file is just a zip file: a single file that rolls up a
whole folder (including sub-folders). An executable jar file is
one of two things: (1)
a zip file that contains Java code and that can be executed directly
without unpacking; or (2) a zip file that contains
Java code that can be imported into your code. We'll try out both
variations below, but will only typically need the second one.
Next, what is CLASSPATH? As mentioned before, an operating
system maintains a few global variables that both the operating
system and applications can access. CLASSPATH is a variable
used by the Java compiler (which is called javac)
and the Java interpreter (which is called java).
The CLASSPATH variable tells the compiler where to find stuff.
The variable holds a string that itself is a concatention of
directory (folder) locations.
There are two types of locations that one puts into the CLASSPATH
string: (1) folders that contain Java code; (2) executable jar files.
This is how the compiler knows where to get libraries, for example.
Let's start by adding a jar file to CLASSPATH.
Topic 3: CLASSPATH in Windows (Windows native)
There are two different complications with Windows:
- Locating the environment variables can be challenging,
depending on which version of Windows you are using.
- The conventions on Windows are slightly different:
- You use Command-Prompt instead of Terminal.
- You use backslash instead of forwardslash. That is, \
instead of /
- Use semicolon instead of colon to separate strings in PATH
or CLASSPATH.
- Use dir instead of ls to list files in
a directory (at the command prompt).
Let's follow the above directions with CLASSPATH for Windows:
- First, let's see what the default CLASSPATH has. Open a
command-prompt and type echo %CLASSPATH%, which
will print out the current value that's in the variable.
Very likely, there will be nothing.
- Make a new folder off of your Desktop called
testdir.
- Now
cd to the testdir folder you created earlier.
- Download testclasspath.jar
into that directory using this browser.
- Open a command prompt and get inside this directory:
cd Desktop
cd testdir
- Type cd at the commandline.
This will give you the full pathname to the directory.
- I'll assume that your username is "Alice" in the example below.
- Suppose the full pathname to testclasspath.jar
is
C:\Users\alice\Desktop\testdir\testclasspath.jar.
- Now, locate the place where you can change environment variables.
- Go to the Control Panel via Settings
and from there to the System menu,
and from there to "Advanced".
Note: in Windows-8 and later, you may need to switch to the
"classic" view just to find the Systems menu. There are youtube
videos that show you how to find the environment variables.
- Now click on setting the "environment variables", after which
you'll see a box that's divided into two parts: (1) the top part
has user-defined environment variables; and (2) the bottom part
has system-defined ones.
- Scroll down the system variables until you see the PATH (or Path)
variable. Then click to "edit" its "value" field. Do NOT
change the name of the variable.
- Most likely, there will not be any variable called
CLASSPATH, so create one, and add the string
C:\Users\alice\Desktop\testdir\testclasspath.jar;.
to the value field.
NOTE: we added the current directory at the end, separated by a semicolon.
Click OK on all the boxes until done.
- Now get rid of the command-prompt and open a new
command prompt (which re-checks the environment variables).
- Type echo %CLASSPATH%. You should see the jar file
in the CLASSPATH. This means you are ready to test.
- Type java testjar.TestClasspath, at the command line,
which will tell you if it all worked.
- Assuming it did, here's what happened. There is a Java
file called TestClasspath.java that was compiled into
TestClasspath.class. This file was in the jar file
testclasspath.jar. You can execute it from any folder
because the CLASSPATH variable has its location.
Just for reference, here's the code.
- Lastly, we will write our own code that uses the jar file
as a library.
- Download TestImport.java
into the testdir directory you created earlier.
- Open a Command-Prompt and cd to the testdir
directory.
- Compile and later execute TestImport.java. If your
CLASSPATH was set up correctly, it should work. Now examine
the code in TestImport.java
to see how it imported the code in the jar file.
- You know know (for the rest of your life) how to
Topic 4: PATH in Windows and new installs of Java
Sometimes, when installing an older or newer version of Java, or installing
a new program that you want to invoke in the Command-Prompt, you
need to put the full directory path in the PATH variable.
For example, let's explain how to install a different version of
Java, for example, Java-8.
- First, google "JDK download", which takes you to the Oracle
page where you can download JDK. Get the basic JDK SE.
Here's the link you should have found:
http://www.oracle.com/technetwork/java/javase/downloads/index.html
- Follow the JDK install instructions to complete installation
(which will take at least a few minutes).
- You now have to tell Windows where the compiler is by
editing the PATH environment variable. This is a bit of a pain
but is done just once.
- First, locate the compiler.
- Open Windows Explorer. Then, click on "C:" or
Local-Disk. Then, enter "Program Files". Most likely, after the
JDK install, there should be a directory called "Java", inside
of which is a directory called something like "jdk1.8.0_65"
(depending on which version of Java you installed), inside of
which is a directory called "bin". If you can't find it, type
"javac.exe" in the search box of Explorer.
- Once you've found the directory (folder), check to see
that the file javac.exe is in the folder. (It might
be presented as javac, without the .exe part.)
- Now that you've found it, you'll need to be ready to
type the full sequence of folders starting from "C:".
Using the example above, this will be something like
"C:\Program Files\Java\jdk1.8.0_65\bin;" (but without
the quotes).
- The string above, such as
C:\Program Files\Java\jdk1.8.0_65\bin;
is called the full pathname to the directory that has the
compiler.
- Next, set this aside but do NOT remove the Explorer window yet.
- Now, locate the place where you can change environment variables.
- Go to the Control Panel via Settings
and from there to the System menu,
and from there to "Advanced".
Note: in Windows-8 and later, you may need to switch to the
"classic" view just to find the Systems menu. There are youtube
videos that show you how to find the environment variables.
- Now click on setting the "environment variables", after which
you'll see a box that's divided into two parts: (1) the top part
has user-defined environment variables; and (2) the bottom part
has system-defined ones.
- Scroll down the system variables until you see the PATH (or Path)
variable. Then click to "edit" its "value" field. Do NOT
change the name of the variable.
- The "value" part probably already has a long string in it.
Go to the beginning of this string WITHOUT deleting anything,
and add the full pathname
C:\Program Files\Java\jdk1.8.0_65\bin; to
the beginning.
- This is IMPORTANT:
- The above pathname is only my example. Your version or
pathname could be different on your computer. Use your pathname.
- It is ESSENTIAL to type in the string exactly, including
the blank betweeen "Program" and "Files" and the semi-colon
at the end.
- Now click OK on all the boxes as you back out towards the
main control panel.
- Next, test that it's working:
- Open a command-prompt. To do this, you can use one
of two ways: (1)
use traditional (Windows-7 or earlier) "start" menu, then
to "all programs", then to "accessories" and then "command prompt";
or (2) search for command-prompt.
- The command-prompt is typically an all-black window with
a simple text interface.
- Type javac and hit return. If the Java JDK
installed correctly, you will see a whole bunch of text (more
than a screenful) spewed out, most of which has lines that
begin with the minus sign. If it did not work, you will
get a "command not recognized" error.