The Runtime Stack
An example without objects
What is the runtime stack?
- This is an actual stack used by the Java runtime system.
- Every language uses some type of runtime stack.
- The stack stores the following for each method:
- Local variables (variables declared inside methods).
- Parameter variables.
- Hidden temporary variables created by the compiler.
- The return address of the code that follows the call to a method.
- Bookkeeping info for the runtime stack manager.
- A method's "stuff" is on the stack only when the method is
active (has not completed).
- All the "stuff" (local variables etc) for a method is stored
together on the stack in a stack record for that particular
method at that moment in time.
- As soon as a method is invoked, a stack record is created
for it and populated.
- When a method completes execution, its stack record
disappears (popped off the stack).
- What's left when a stack record is "popped" is the
stack record below it.
Consider this example
public class RuntimeExample {
public static void main (String[] argv)
{
// 1.
methodA ();
// 7.
}
static void methodA ()
{
int i = 5;
// 2.
methodB (i);
// 6.
}
static void methodB (int k)
{
int j = 7;
k ++;
// 3.
methodC (k, j+1);
k ++;
// 5.
}
static void methodC (int a, int b)
{
// 4.
System.out.println (a + b);
}
}
Exercise 1:
Without implementing, mentally execute the above program
and determine what gets printed out.
Let's now draw the stack at each of the moments numbered 1 to 7 above.
- At the moment of execution marked by "1" above:

- At marker 2:
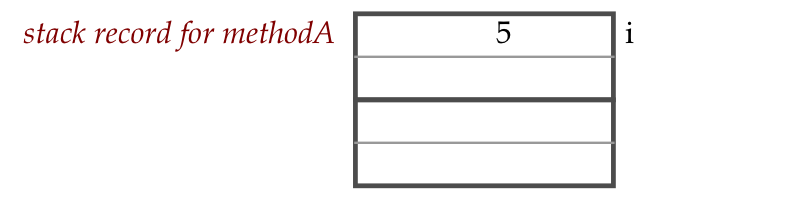
- At marker 3:
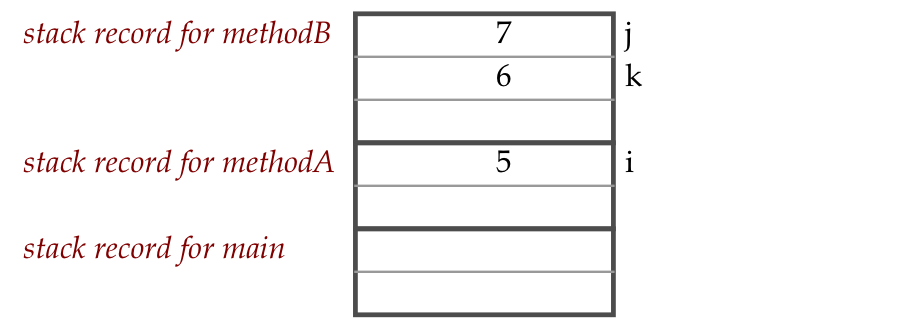
- At marker 4:
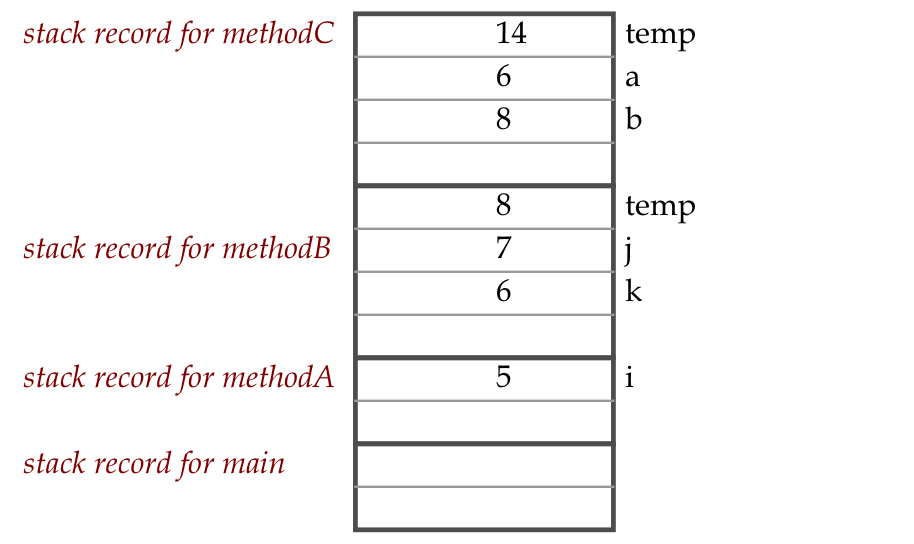
- At marker 5:
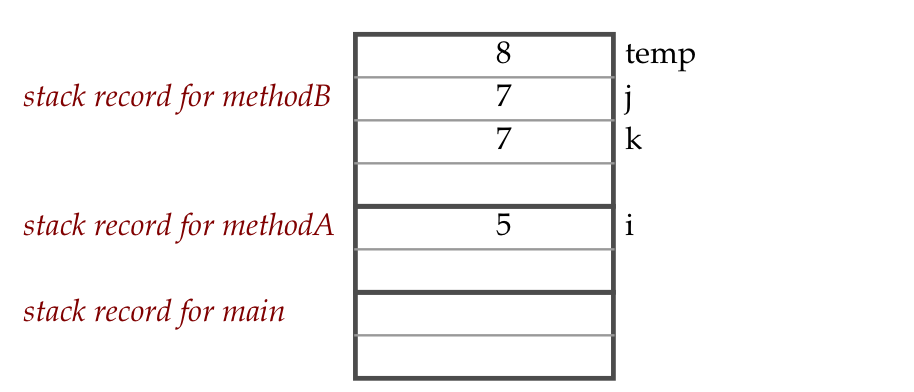
- At marker 6:
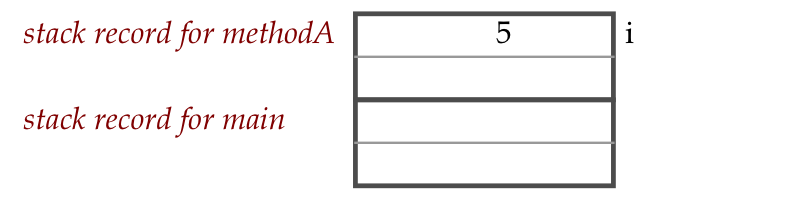
- At marker 7:

An example with objects
Consider this example
class ObjX {
int methodA (int k)
{
return methodB (k, k+1);
}
int methodB (int a, int b)
{
return (a + b);
}
}
public class RuntimeExample2 {
public static void main (String[] argv)
{
ObjX x = new ObjX ();
int d = x.methodA (5);
ObjX x2 = new ObjX ();
d = d + methodC (x2);
System.out.println (d);
}
static int methodC (ObjX y)
{
int z = y.methodA (6);
return z + 1;
}
}
Exercise 2:
Without implementing, mentally execute the above program
and determine what gets printed out.
Exercise 3:
Draw the various stages in the evolution of the stack.
That is, draw the state of the runtime stack each time
a new stack record is created or removed.