In this module, you will learn how to:
- Download, compile and execute a Java program.
- Learn a little about software in general.
- Modify a Java program.
Background needed:
- You need to be comfortable using a web browser.
- You should be familiar with basic computer-use concepts
such as:
- Files
- Editing plain text, using something like Wordpad or Notepad.
- Internet use: browsing, downloading from a website.
- Unpacking a file-collection (e.g., a Zip file).
- Installing software.
Note: We will describe how to get things done in
Windows (Win-XP or Win-2000).
Linux users already know how to issue commands from the
commandline. Mc-OS users may know, but if not, can
run a Unix-like commandline. Luckily, for compiling
and running Java programs, the actual commands are very
similar in all three operating systems.
Getting used to the commandline
There are two ways to compile and run programs:
- Method 1: Use a development environment. These
are sophisicated software packages entirely targeted at
helping programmers write programs. Examples include:
JGrasp,
Eclipse,
SunOne and
JBuilder.
These combine an editor, compiler and project-manager all into one
along with some program development tools beginners don't need.
- Method 2: Install a compiler, use your own editor and
compile/execute from the command-line.
It's worth trying both, just to see how they work. We'll focus
on the more elemental command-line approach here.
- Step 1: First, lfire up a command-line window:
- Go to "Start", then "Run".
- This will bring up the "Run" window. Type cmd
in the "open" field, and hit return or "OK".
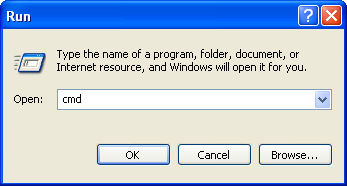
- This should bring up a command-line window that looks this:
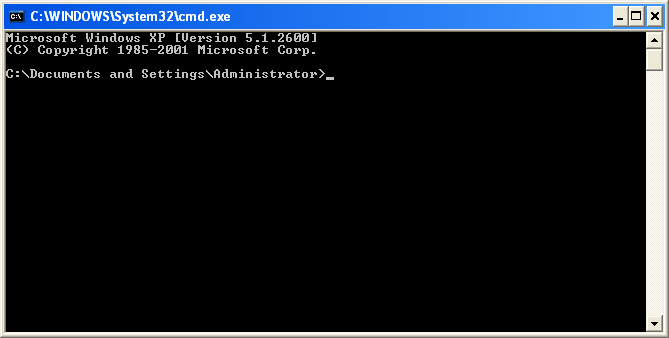
- Step 2: Create a new directory in which to play:
- Use cd .. to go up in the directory structure,
mkdir to make new directory, and dir to
examine the contents.
- For example, let's create a directory called myproject
off of the c: drive. Notice that we backed up from
a directory called Administrator (which happens to
be the username of this user), then applied mkdir myproject
to create the directory, and then typed cd myproject
to enter the directory itself. Finally, we examine the list
of files (none) in the directory itself using dir.
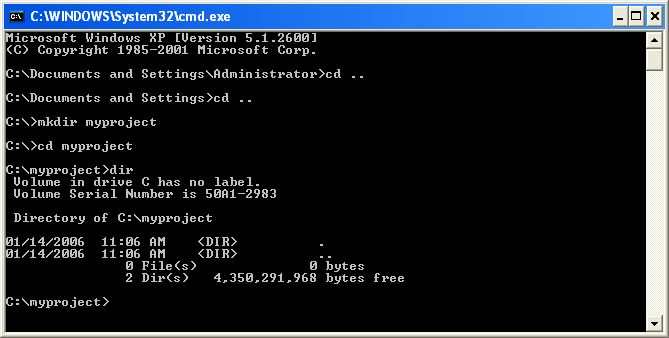
Editing
Now, we will use a simple text editor like Notepad
or Wordpad and create a Java program:
- Step 1: Fire up the editor. Go to "Start", then
"Programs", then "Accessories", then click on either
Notepad or Wordpad. This will bring up
the editor.
- Step 2: In your browser, go to
this link.
- Step 3: Cut and paste from the browser into Notepad:
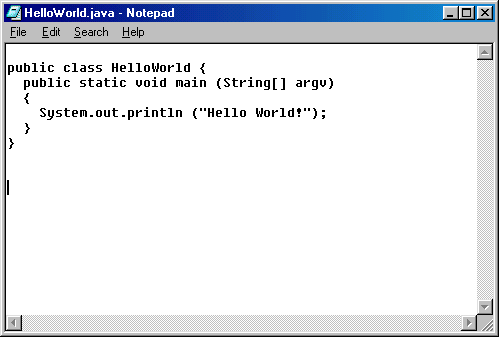
- Step 4: Save the file into the new directory that
you created above.
- Important: Don't make any changes to the text
that you pasted into Notepad.
Installing Java
Compiling and executing
Now that Java has been installed, you should be able to compile
our test program called HelloWorld.java.
- Follow Step 2 of these instructions
to compile and execute.
- When you typed javac, that part is
called compilation, which prepares a Java program for
execution.
- When you typed java, that actually causes
a Java program to execute.
Compiling and executing a more complex program
- Download this Java program:
HelloWorldWindow.java.
- Compile at the commandline by typing javac HelloWorldWindow.java
- Execute by typing java HelloWorldWindow
- This is what you should see:
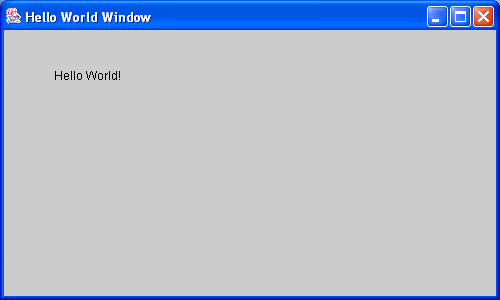
How do you get rid of a Java program when you're done? Two ways:
- The easiest is to type Control-C (control key and "C" held
together) in the commandline window from which you executed
the program.
- If your program is set up for "quitting", then you can
close the window by clicking on the "X" at the top-right.
Note: This won't always work because not all programs have
been set up for it, in which case you need to use the Control-C
option.
Exercise:
Instead of printing "HelloWorld!" to the screen,
can you make the program print your name instead?
- Bring up the program in an editor.
- Go to the line where it says drawString ... etc.
- Change the string inside the double-quotes to your name.
- Compile and execute.
Congrats, you've just edited (slightly!) a program, compiled
it and executed it.
Exercise:
Sometimes programs come in multiple files. For example,
the same HelloWorld program can be written in three
files. To see how this works,
download the three files
HelloWorldWindow2.java,
TestFrame2.java,
and TestPanel2.java,
then compile the first one and execute by typing
java HelloWorldWindow2 at the command-line.
Exercise:
You need to try one more thing: unpacking an archive.
Create a fresh directory, then download
this ZIP file into the
new directory and unpack it. Then follow the steps in
the exercise above.
What does it all mean?
OK, thus far we've learned how to compile and execute
a program, possibly without understanding what we're doing.
So, let's explain.
Let's start by looking at compilation and execution:
- First, software vs. hardware.
Hardware, as the word sounds, is all the solid stuff a
computer is made of, that will make a sound if you drop
it on the floor. Software, on the other hand, lives
entirely in electronic form, and consists of programs,
each of which are a collection of instructions that "run"
or "execute" on the hardware. All it is, really, is a collection
of detailed instructions telling the hardware what to do and when.
- Now, the hardware is only able to execute programs written
in "hardware" language, consisting of instructions that are
so low-level that nobody wants to write programs directly
for hardware. A program that's written in low-level instructions
that is ready to execute is called an executable program.
- Software developers instead write programs in higher-level
languages that have names you may have heard of: C, C++, Java
and so on.
- Since the hardware can't directly execute these high-level
programs, we use a special tool (itself a piece of software)
called a compiler that translates the high-level program
into a low-level program suitable for the hardware.
Thus, when you run a compiler, it (the compiler) examines
a high-level program (sometimes called the source-code),
and translates that into an executable.
- All software that you download and install consists
of executable programs. You very rarely (as you did today),
download high-level programs to first compile and then execute.
- If you work with the source-code, then you can make
changes to the program, compile and execute (as you did
in the exercise above).
- If you wish, you can create your own programs from
scratch in a high-level language. This is the task called
programming, or software development
when it's done at a larger scale. To do this, however,
you need to learn a little computer science.
Now, let's say a few words about Java:
- Java is one among many high-level languages.
- One of the simplest examples of a Java program is
the first HelloWorld.java program you saw earlier.
- Let's examine this program:
public class HelloWorld {
public static void main (String[] argv)
{
System.out.println ("Hello World!");
}
}
- Note that, like English has words,
this language has words like static and argv
(How can you not like a word like argv?!) as well
as syntactic elements like braces, parentheses and semi-colons.
- Generally, while English is somewhat forgiving in that
a writing course might forgive spelling errors, a compiler
will refuse to compile a program that isn't 100% syntactically
correct. This fussiness causes beginning programmers to pull
their teeth initially, but all programmers eventually get
good at avoiding syntax errors.
Exercise:
Deliberately create a syntax error and see what happens.
For example, misspell the word class.
- If you like, you can learn more about a simple Java
program by reading through
this material,
which is taken from an intro course (no background needed).
- Do not expect to understand programs without taking
courses or spending time on this matter. It's like learning
a foreign language - it's not going to happen in a week.
What next
To learn more about programming and computer science:
- See this article:
Why Scientists
Should Learn Computer Science. It's written
for faculty, but should make sense to students too.
- Learn yourself: You can use
this
intro course on Java that's based on Java applets.
This will take time, and you won't become an expert
anytime soon.
- There are a gazillion books that proclaim to teach you
Java or C or whatever in 21 days. There are equally
many websites with the same claim.
For a contrasting view, see
this article by Peter Norvig.
- Take courses. At GW, you would take
CSci-53 and CSci-133 to get started. Or talk to an advisor.