Computing Overview
Aspects of Computing
- Problems
- Algorithms and data structures
- Programming languages
- Operating System
- Hardware.
Software Life Cycle
Cooking Analogy
- Requirements: vegetables, sauce, rice, pasta,...
- Design: recipe
- Coding: actual cooking
- Testing: tasting
- Maintenance: adjusting salt-level, preservation,...
Algorithm Complexity
- Performance measure using big O notation
- Linear time algorithm: O(n) - running time linear in problem size
- Quadratic time algorithm: O(n2) - running
time is square of problem size
- NP-Complete: so far, no polynomial time algorithm is known for the problem
- Exponential time algorithm: O(en) running time exponential in problem size
Exercise 1:
- Given a set of numbers, describe an algorithm to find the minimum.
- What is the time complexity of your algorithm?
The field of computer science
- Only a few decades old (unlike biology).
- Current professions:
- Computer Scientist (BS, MS, PhD)
- Software Engineer (BS, MS)
- Computer Engineer (BS, MS)
- Information Technology Specialist (BS, MS, MBA)
- Research/Academia (MS, PhD)
- Major areas:
- Theory/Algorithms
- Systems (networks, OS, architecture, distributed computing)
- Embedded systems
- Software engineering
- Artificial intelligence (including robotics, natural
languages)
- Graphics, human-computer interfaces
- Languages (language design, compilers)
- Scientific computing
- Bioinformatics, computational biology
Computing Systems
Components of a Computing System:
- Hardware
- Operating System (OS)
- Support/development tools (e.g. compiler, editor)
- Applications
Hardware:
- Von Neumann Architecture: data and programs stored in memory to be accessed by processor.
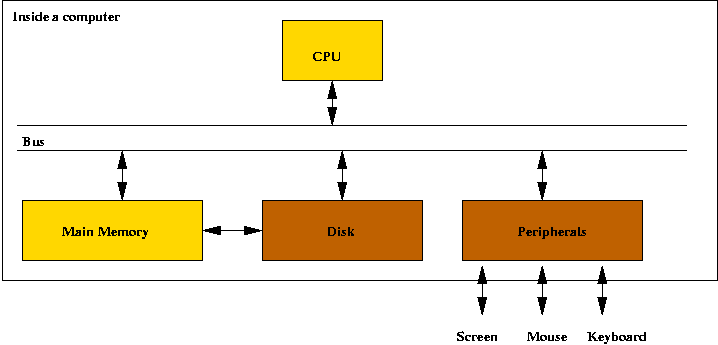
- Memory
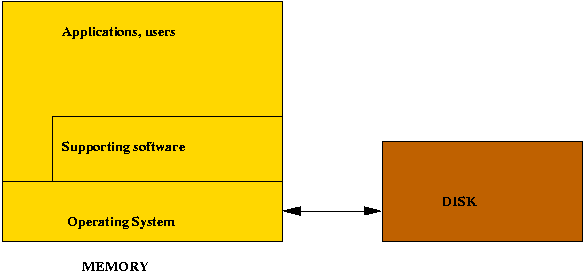
- Processor
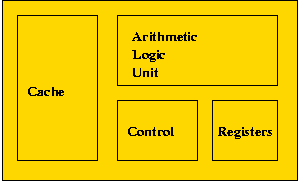
- I/O:
- Input: keyboard, mouse
- Output: screen, printer
- Input and output: floppy, CD/RW, zip-drive
- More sophisticated systems:
- Multiple caches (Memory bottleneck)
- Faster processors (instruction-level parallelism)
- Multiple processors
- Parallel/super-computers
- Distributed computing
Operating System
- Collection of software as layer between applications and hardware
- Control program that manages system resources
- Examples: Unix, Windows, Linux, MacOS,...
- Operating system concepts:
- Process:
- An executing program needs resources: memory, CPU, disk space
- Process: a "sandbox" for a program to execute in.
- OS manages/schedules multiple processes
- API (Application Programmer Interface):
- Mechanism for requesting resources from OS
- Programmers usually do this via a library in a
higher-level language
- Goals of an OS:
- Make hardware easy to use.
- Efficient use of resources.
- Facilitate multiple users, programs.
- Standardize communication between programs.
- Well-known OS's:
- UNIX:
- Created at Bell Labs in 70's.
- Many "flavors" of Unix: System V, BSD Unix (Berkeley), Solaris (SUN).
- Small variations among Unix flavors.
- Linux:
- Free Unix-type OS created by Linus Torvalds and programmers around the world.
- Originally for PC's, but now also for servers.
- Distributions: Redhat, Debian, Mandrake,...
- Windows:
System software
- Usually packed/sold with OS
- Independent of applications and common to all applications
- Examples: compilers, interpreters, libraries, user interface, database, resource management
- Needed for basic running of the computer
Programming (Low Level)
- Machine code: low-level instructions understood by hardware
- Assembly code: "human-readable form of machine code"
Slightly higher-level than machine code, usually 1-1 correspondence.
- Machine/assembly code is Processor dependent
- Initially, programs were written in machine code
- High level programming languages: more efficient, readable and portable.
- Consider the statement A = A + B:
It can be replaced by a sequence of instructions in assembly
Exercise 2:
Write assembly (low-level) code for A = B + C - A, by drawing
a picture similar to the one above.
High-level programming languages
Note: This program simply prints Hello World! to the screen.
It is a 6-line program.
Contrast: When converted into low-level code, it is over 400 bytes.
Compilation and Interpretation:
- A compiler translates from high-level code to machine
(low-level) code.
- Compilation: only translation and not execution; execution is separate.
- An interpreter translates and executes line-by-line.
- Interpretation is usually slower for overall execution.
- Compilation can optimize low-level code based on program structure.
Languages:
- FORTRAN (1960's): one of the earliest high-level compiled languages.
- C: (1970's) with UNIX, compiled language
- C++: (1980's) based on C, compiled object-oriented language
- Java:(1990's) syntax based on C++, interpreted object-oriented language
- Interpreted (scripting) languages: Perl, Python,...