By the end of this module, for simple programs with real
numbers, you will be able to:
- Demonstrate ability to declare unidimensional
arrays and populate them, with integer and doubles.
- Demonstrate knowledge of array limits, length.
- Demonstrate simple operations like printing and copying.
- Demonstrate mental execution and debugging of
array-based programs.
- Identify new syntactic elements related to the above.
A group-of-items variable
First, recall our "box" analogy for a variable:
- Suppose we declare and assign a value to an int
variable i
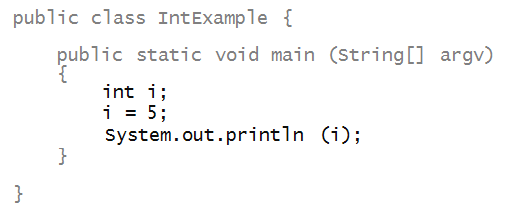
- The variable is like a "box" that can hold one
single integer value at a time.
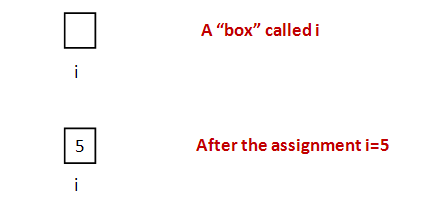
An array:
- The analogy for an array: a "box" that
stores a group of related items arranged linearly:
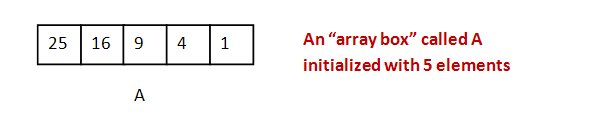
- The code that created the above:
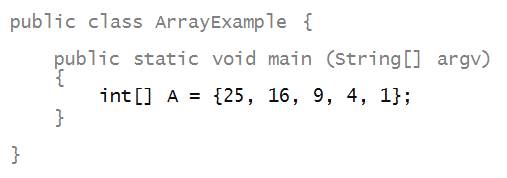
- Note: the following is not allowed in Java:
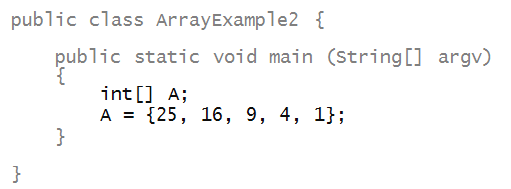
- Here is another way of accomplishing the assignment:
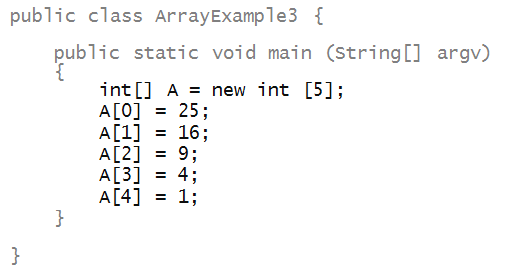
- Perhaps the most common way looks like this:
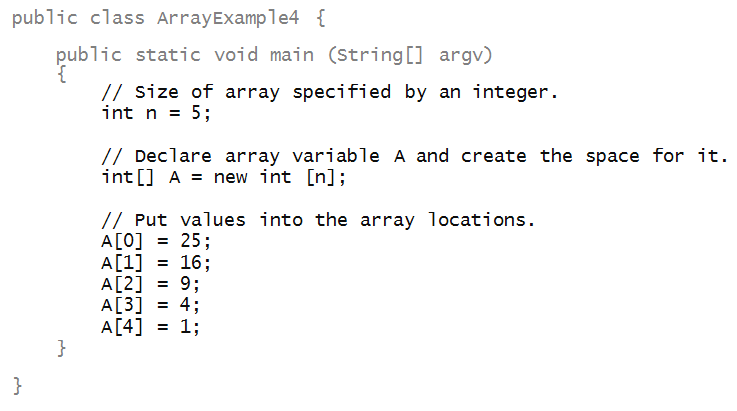
- Next, let's print the elements of the array:

In-Class Exercise 1:
Modify the above program (ArrayExample5.java)
to include a sixth element so that the elements
in order are: 36, 25, 16, 9, 4, 1. Print
the elements and the array length.
Let's look at a few details carefully:
- First, the declaration:

- It starts with the type of value in
each location of the array. Here: int.

- Then the square brackets together.

- Finally, the array variable name. Here it's A.
=> This is a name we chose.

- Next, unlike int's or double's,
we need to explicitly create space for arrays:

- Uses the reserved word new:

- This is followed by the type (here, int):

- Which is followed by the desired size in square brackets:

- Note: the value of the int in n is
used for the array size.
- So, when do we use
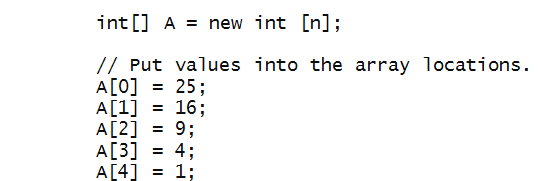
versus

- Use the latter for small arrays when all the array
contents are known.
- Use the former in all other cases.
- The value used to access one of the array locations
is called an array index:
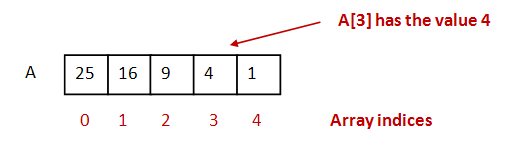
- The first location always has index 0.
- If the size is n, the last index is n-1.
- An array index is always a non-negative integer.
- Finally, every array variable has an associated
length:

- This length is an integer.
- Note: the length is NOT the last index.
=> It is one more than the last index.
- The length will be useful later.
How to read aloud:
Let's now deliberately make a mistake to see what
happens: we'll use an index that's "out of bounds"
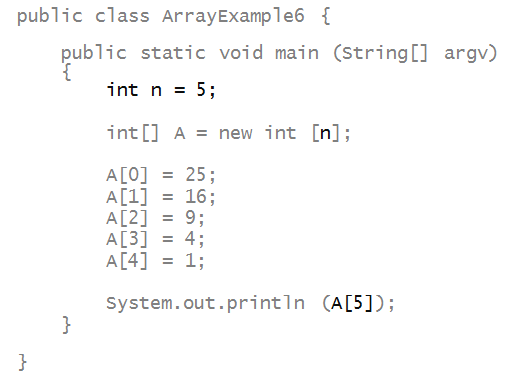
In-Class Exercise 2:
Edit, compile and execute the above program to see what happens.
Arrays and for-loops
Arrays go hand-in-hand with for-loops.
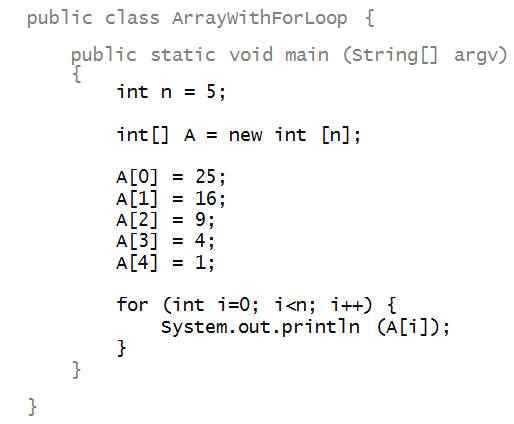
- Note that the loop variable is used as the array index:

- Here, the loop variable i takes values
0, 1, 2, 3, 4 in successive iterations.
- For example, in the third iteration
- i is 2.
- Therefore, A[2] is given to
System.out.println.
- The value of A[2] is 9 (third element).
- The value printed is therefore 9.
- Here, we are cleverly using the for-loop variable i
for two different purposes:
- One is to serially traverse the array.
- The second is to use its value in an arithmetic expression,
whose value is eventually stored in the array.
We can use for-loops for filling in the elements of
an array, e.g.,
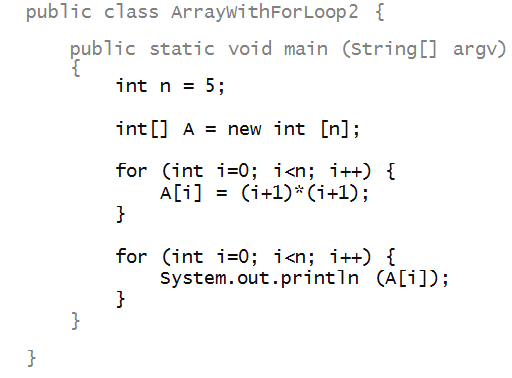
In-Class Exercise 3:
Trace through the first for loop iteration by iteration,
and draw the contents of the array.
In-Class Exercise 4:
Modify the above program
to include a sixth element, 36.
Also, change the order of elements so that the order is:
36, 25, 16, 9, 4, 1. That is, the first element
in the array (A[0]) is 36, the second is
25 ... etc. Print the elements.
In-Class Exercise 5:
For your modified array above, change the for-loop
to print the elements in
reverse order, starting with A[5].
Arrays of double's
Consider the following example:
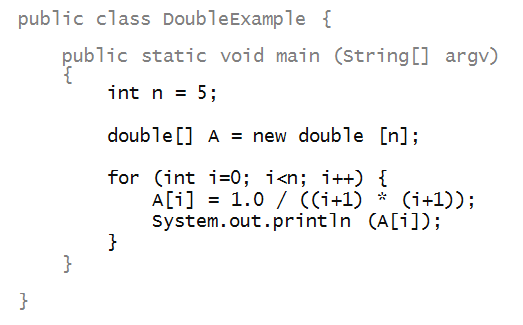
- Here, we have declared an array variable A
for an array of double values:

- And created space for it (5 slots):

- Then, we assigned values in a for-loop:

- And used those values in printing:

In-Class Exercise 6:
Modify the above code to compute the sum (of the
first n terms) of the series
s = 1 + 1/22 + 1/32 + ... + 1/n2.
Then, compute the value 6s and find its square root
(perhaps using a calculator). Try this for different values
of n. What do you think the series converges to?
Default values
Consider this program:
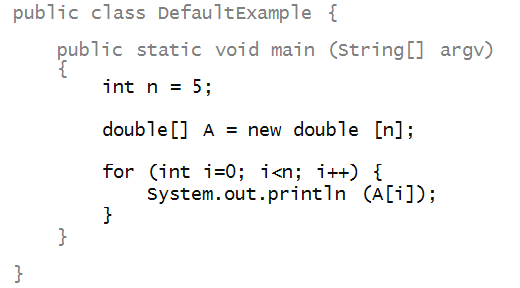
In-Class Exercise 7:
What does the above program print? What do you conclude
about the default values placed inside an array?
Is it the same for integer arrays?
Assignment and copying with arrays
Consider the following program:
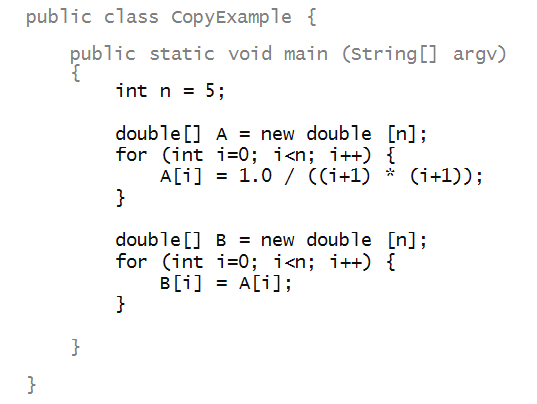
- Here, we have created a second array B:

- Each element is copied one by one:

In-Class Exercise 8:
Draw out each array and trace through both for-loops
showing, step-by-step in pictures, how elements get
copied from one array to the other.
Now for something strange:
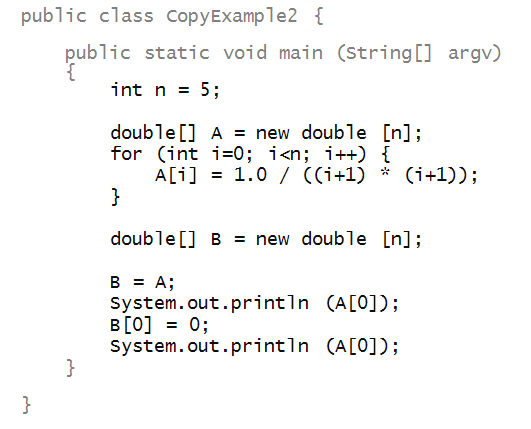
In-Class Exercise 9:
What does the program print? Try it and see if you can
explain the result.
About array copying and assignment:
- Yes, it's a little strange.
- When array variables are used in direct assignment, as in

- The variable B gets associated with the
same array space as A's.
- We say that "A and B
point to the same array space".
- This is why B[0] and A[0] are
the same "box".
- This is true for the other slots.
- For example, B[1] and A[1] are
the same "box" too.
In-Class Exercise 10:
Does it work the other way around?
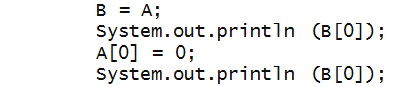
What gets printed out?
In-Class Exercise 11:
Consider this program:
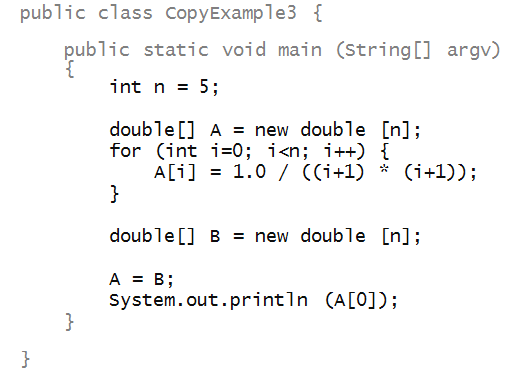
What gets printed out?
More examples
Example 1: let's write a program to "rotate" an array:
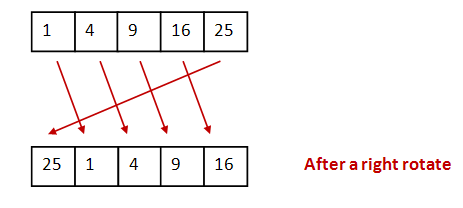
- Thus, we want to shift each element into the next position rightwards.
- The last element gets into the first position.
- First, we'll use the help of an additional array to
make this happen:
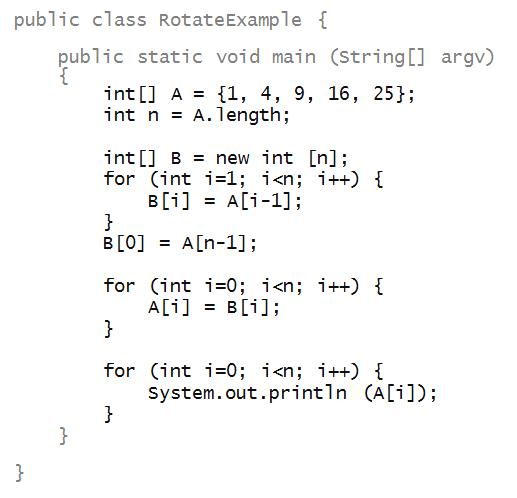
- We first copied elements A[0],...,A[n-2] into
locations ("boxes") B[1], ..., B[n-1].
- Then, we copied A[n-1] into B[0].
- The array B is in the desired form.
- Lastly, we copied all of B into A
so that A has the desired rotation.
- It is possible to do without an additional array:
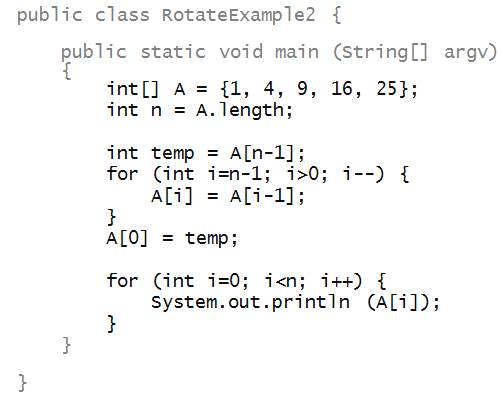
In-Class Exercise 12:
Trace through the above example (RotateExample2.java)
by hand to see how it works. Then, also trace through
the version below and explain why it does not work:
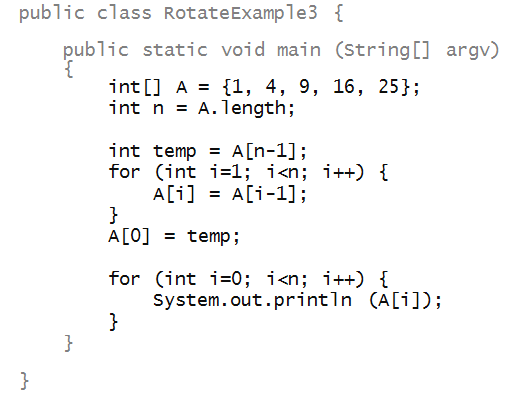
Next, in the second example, we'll write code to reverse an array:
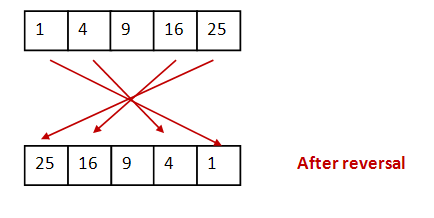
Here's a version that uses an additional
array:
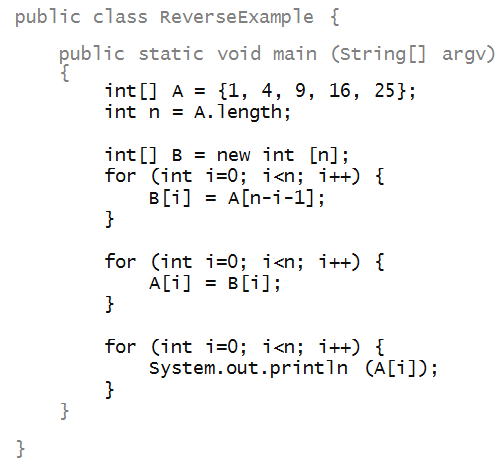
In-Class Exercise 13:
Trace through the program above.
Can you think of a way of achieving the reversal
in the same array A?
Example 3: adding two arrays
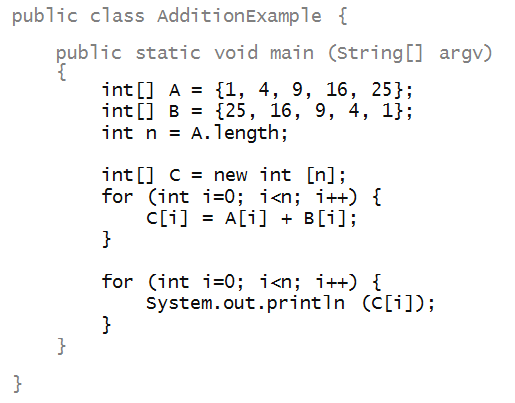
- Here, we have added two arrays element-by-element.
- The resulting values are put into a third array
(C).
In-Class Exercise 14:
Trace through the program above. Then write another
program (call it AdditionExample2) that
takes an array A and computes the sum of
the array A and its reversal, putting the
result into array B. Can you do
this without using a third array?
In-Class Exercise 15:
Trace through the program below by hand.
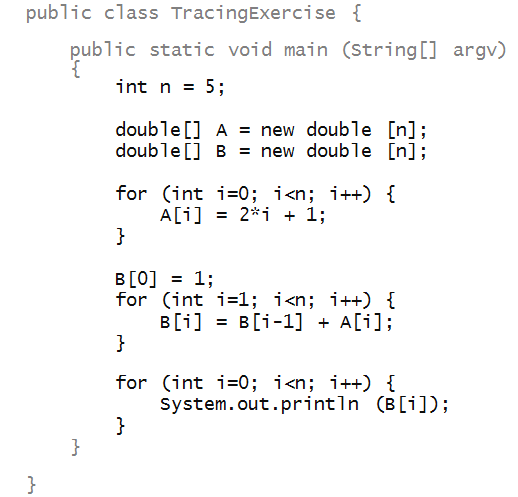
What does it print?
In-Class Exercise 16:
Trace through the program below by hand.
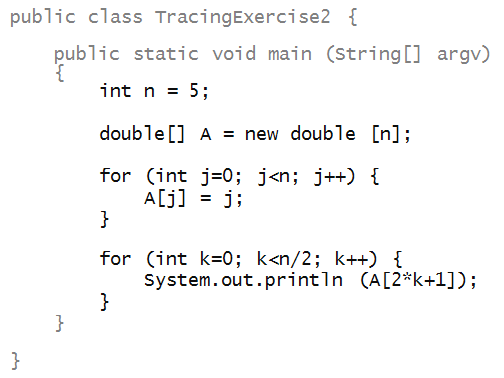
What does it print?
In-Class Exercise 17:
Trace through the program below by hand.
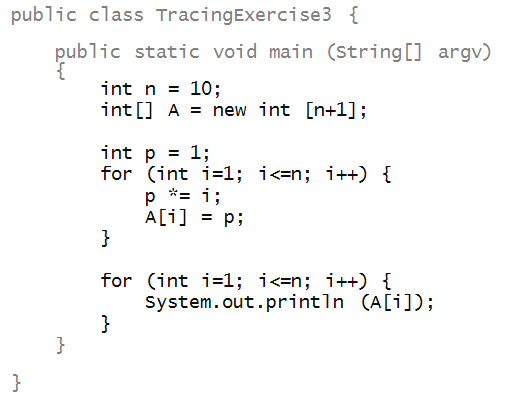
What does it print? Look up the definition of
factorial somewhere and relate that to
the computation above.
Note: in the exercise above, we learned a few new
things about using arrays:
- Sometimes, we create one additional slot so that
we can use the index A[n] when convenient.
- A for-loop can have other variables that change
with the loop besides the loop variable.
Reading and writing
Let's look at an example and how to read the code:
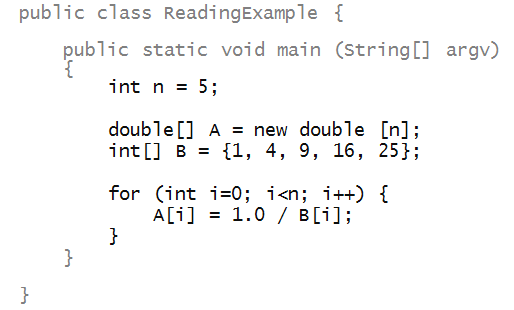
- First, look for the array declaration and identify the
type (e.g, int or double etc):

- Say to yourself, "A is an array that holds
double's".
- "B is an array that holds
int's".
- Then, look to see how much space is created:

- Say to yourself, "A's size is 5, created
using the new operator".
- "A's elements are all initially 0".
- "B's size is 5, directly initialized to
particular values".
- Now, check that, when variables are used to access
array components (slots), the bounds are right:

Writing:
- Writing the declaration:

- No spaces between either the type (double),
nor between the brackets.
- A single space between the right bracket the array variable
name.
- Creating space:

- A space on either side of the assignment operator, =.
- A space on either side of the new reserved word.
- The space after the double is optional. (Here, we have
a space.)
- No spaces for the size and brackets.
- Prefer the use of the new operator except
for small-ish arrays whose initial contents are known and fixed.

- No spaces when using the values in an array:

Using the array's built-in length. Consider this variation:
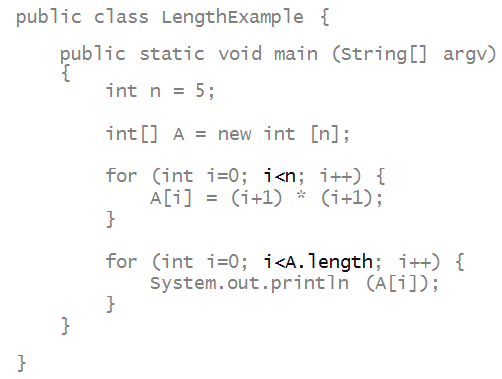
- We could either n or the array's length A.length.
- So, which one should we use?
- Generally, when the limit is a mathematically meaningful
variable like n above, you can use n.
- Otherwise, use the array's length.
- Sometimes, using an array's length variable can make
a program needlessly verbose.
- For example, here's the array-reversal example using
the length variable:
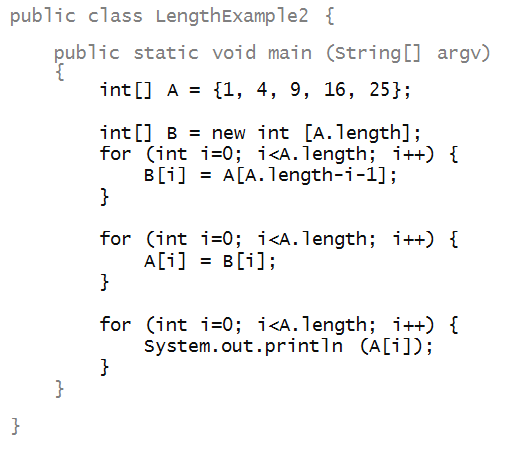
Variable names:
- Here is the first length example, re-written with
different variable names:
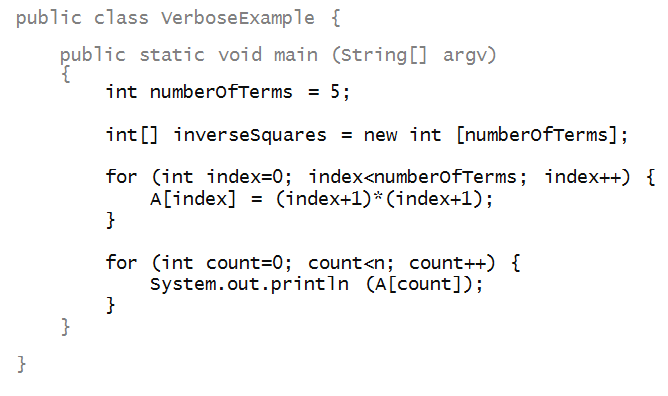
- For a program this short, it is probably a bit verbose.
- Notice: we used different names for the loop variables
in the two for-loops.
When things go wrong
In-Class Exercise 18:
Which of the following have errors (syntax or bugs)
and what are they? Can you tell just by reading?
Don't pay attention to the purpose - just whether
the program will compile or run.
Program 1:
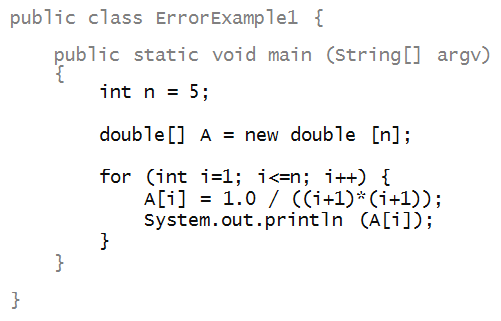
Program 2:
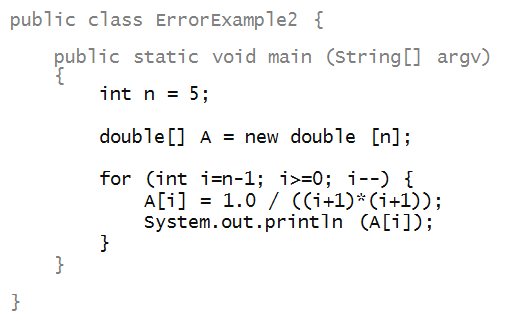
Program 3:
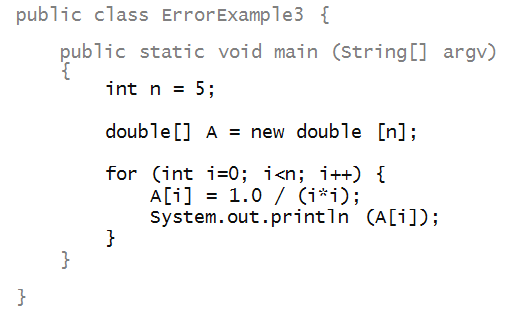
In-Class Exercise 19:
The following program has multiple errors. See if you
can spot them by reading. Then fix the errors by
editing the program.
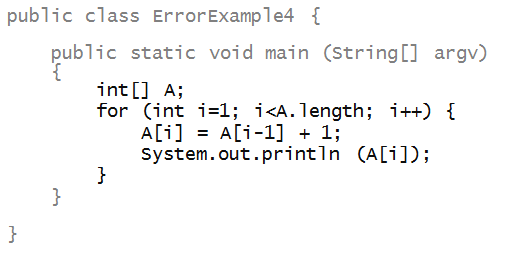