Consider the following program:
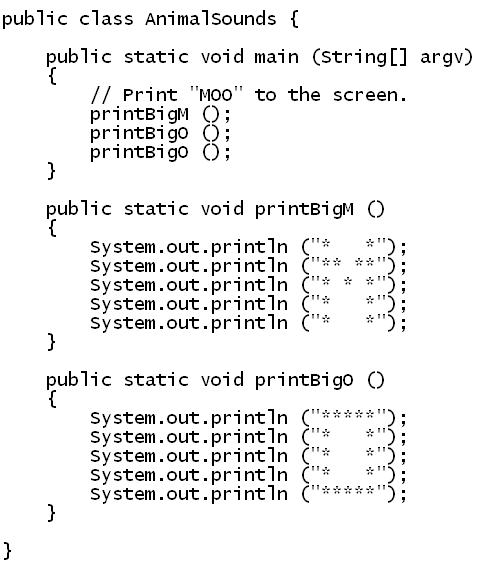
In-Class Exercise 1:
Type, compile and execute the above program.
Now let's point out a few things:
- First, the structure of the overall program: a class
with three methods inside:
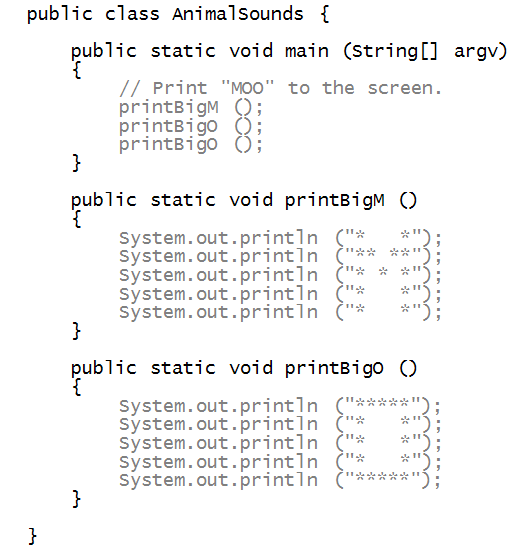
- The three are: main, printBigM, and printBigO.
- Next, observe that the word printBigM appears twice:
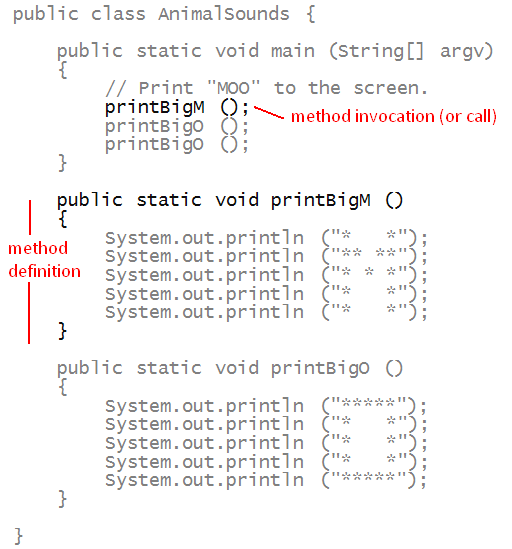
- It's essential to distinguish between the two.
- The single-line use is a method call or
method invocation.
- The bigger structure with lines of code is
called the method declaration (or definition).
- There is similarly, a declaration for
printBigO.
- But there are two calls for printBigO.
- A method can have only a single declaration, but
can be called any number of times.
=> In fact, that is the whole point of methods.
- Jargon alert: in other programming languages, methods are
referred to as functions, procedures or subroutines.
In-Class Exercise 2:
Write your own animal sound that uses one method at least
thrice with an exclamation mark at the end, e.g., print
the big version of BAAA!.
Once your program is working, say the sound out aloud
to the class.
Next, let's see how methods "work" by
drawing an analogy:
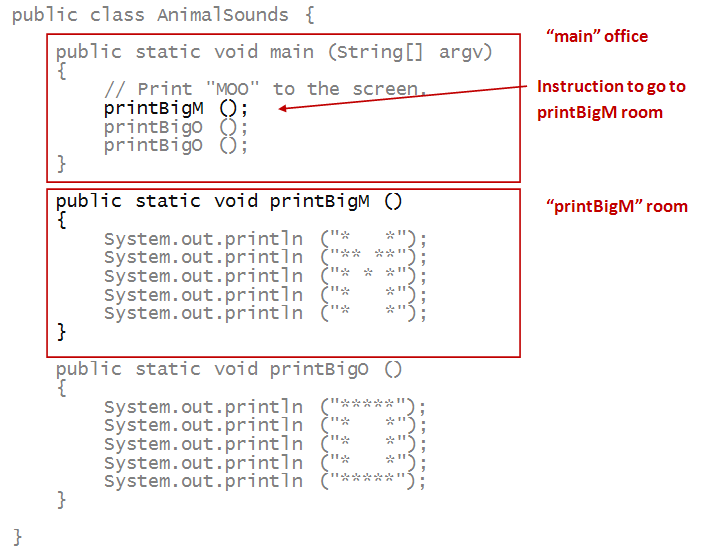
- Think of the class as a building.
- Think of the method main as the entry point
=> Lobby or main office.
- A Java program will always start executing with
the first statement in main.
- Each method corresponds to a room in the building.
- Here, a method call is a command to go to that
particular room.
- Each room has its own commands (Java statements).
=> These are executed upon entry to the "room".
- Important: When you complete the statements in a room,
you go back to the place in main just after where
the "room" was invoked.
Using numbers, let's trace each step in sequence:
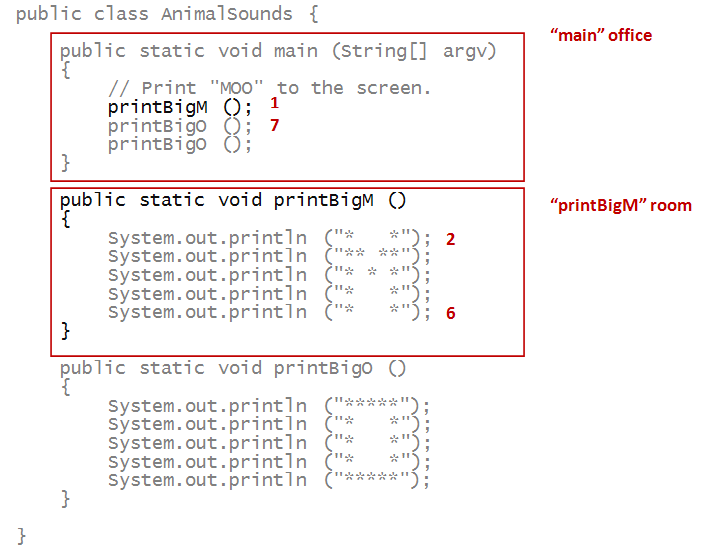
In-Class Exercise 3:
Try these questions for the above program:
- What is the 12-th statement to be executed?
- What step-number corresponds to the second method call
to printBigO?
- What is the 15-th statement to be executed?
Calling methods from other methods
Consider this program:
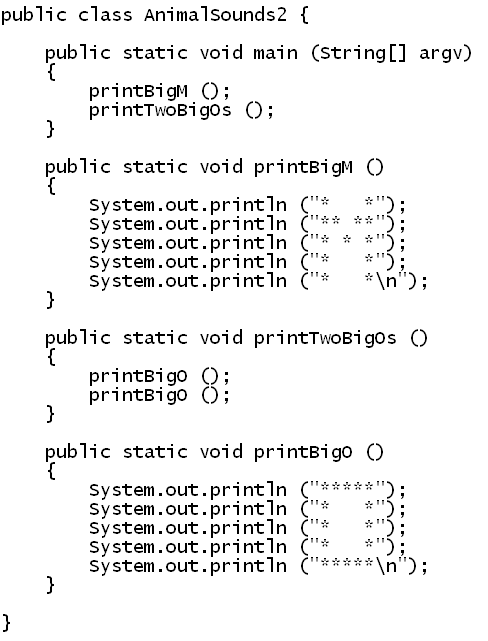
- Yes, we can write our methods that call our own
methods.
- Incidentally, did you notice the escape sequences?
=> We improved the output.
In-Class Exercise 4:
Re-write your own animal sound using this idea.
That is, use a single method for the letter that
repeats, then call a single method from main
that then has the repetitive method calls.
Once again, let's trace through some steps:
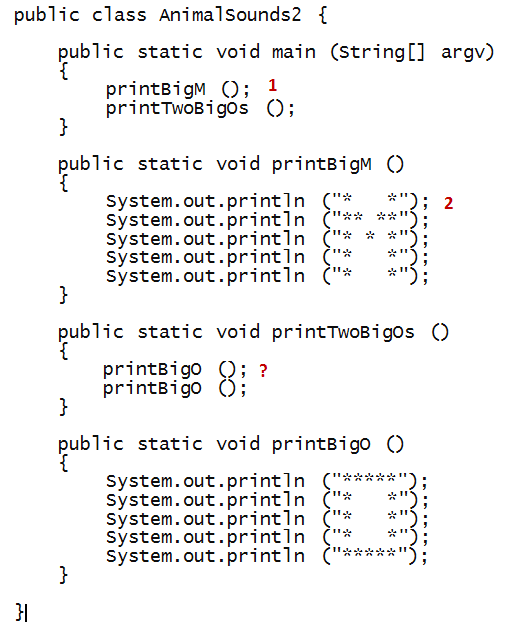
In-Class Exercise 5:
Which step corresponds to the question mark above?
Where in the program would we be at the 15 step?
Mental execution:
- We will use the term mental execution
for the above exercise of tracing through the execution
without actually compiling and running the program.
- Note: Mental execution is extremely important in
developing programming skill
=> Please be sure to practice this with every program you read or write.
- We can't emphasisize this enough. Really.
Reading and Writing
First, let's talk about writing:
- As usual, when writing methods, type in both
parens and both braces before typing what's in between.
- Generally, use the style we have been using:
- Proper alignment of braces under the p of public.
- A space between the method name and the parens.
- You can make up method names, but follow the preferred style:
- Start with a lowercase letter.
- Capitalize the start of different parts of the name.
- Note: class names (like AnimalSounds)
should start with a capital letter.
An important thing to understand about identifiers:
- Consider the method name printBigM.
- This is a name (identifier) we chose.
- We could just as easily have used myCrazyMethod:
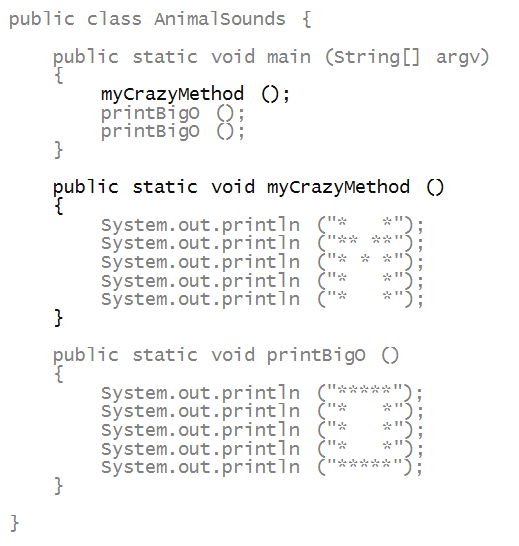
- In other words, the compiler does not look into the
English meaning of identifiers.
- We choose identifiers to help us read programs.
A few more writing issues:
- It is possible to write a method declaration before
or after any invocation. For example, we could have written:
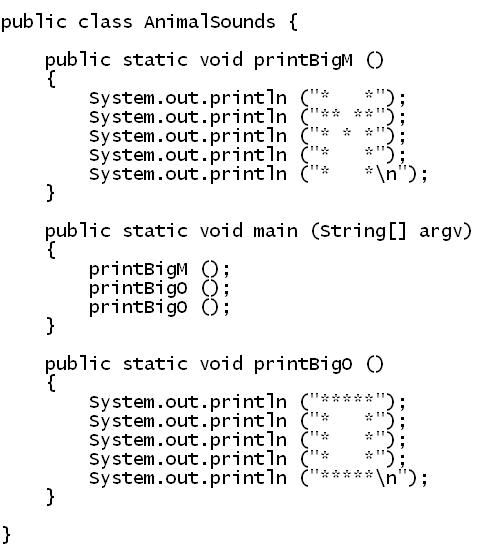
- There are three conventions typically used:
- Always declare methods before their first invocation.
- Try to declare methods near their first invocation (just before
or just after).
- Or, group method declarations according to common theme.
(For large classes with many methods.)
- For main, there are also two conventions:
- main is the first method in the class.
- main is the last method in the class.
- What cannot be done: write a method declaration outside
a class:
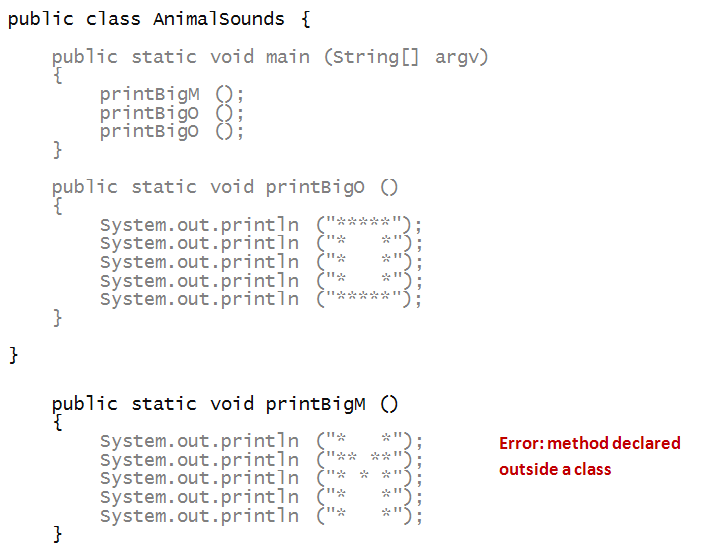
- Also, we cannot declare a method inside another method, as in:
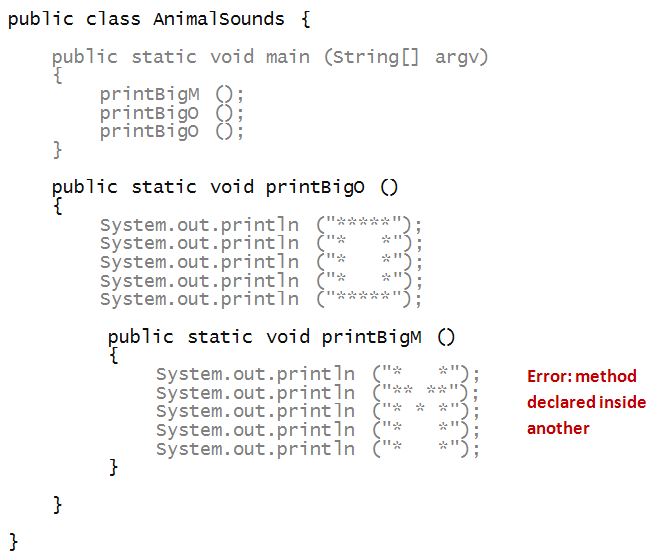
- Not that you'd want to, but
you can declare a method but not invoke it anywhere.
- You can declare any number of methods inside a
class.
In-Class Exercise 6:
What would happen if we changed the order of method
invocation? For example:
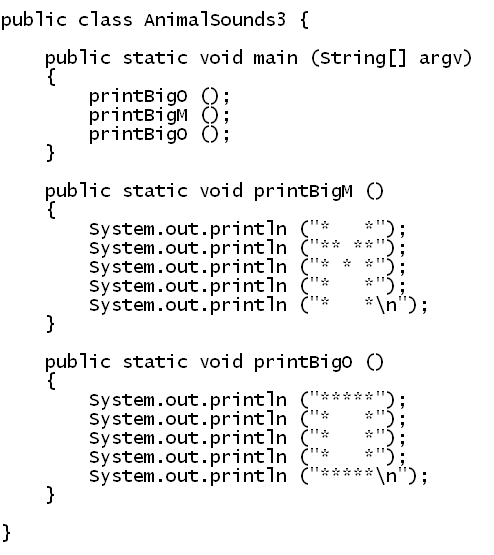
About name clashes:
- Java allows two methods to have the same, provided
they are different in some ways defined by the language.
- This is a somewhat complex issue at this point,
so we will simply give different method names.
- One can also use the class name as
a method name, but this is considered poor stylistic practice.
When things go wrong
In-Class Exercise 7:
What is the compiler error you get when a method is
accidentally declared outside a class?
In-Class Exercise 8:
What is the compiler error you get when a method is
accidentally declared inside another method?
In-Class Exercise 9:
What is the compiler error if we misspell
printBigM in the first AnimalSounds program?
And now for something strange
Consider the following program:
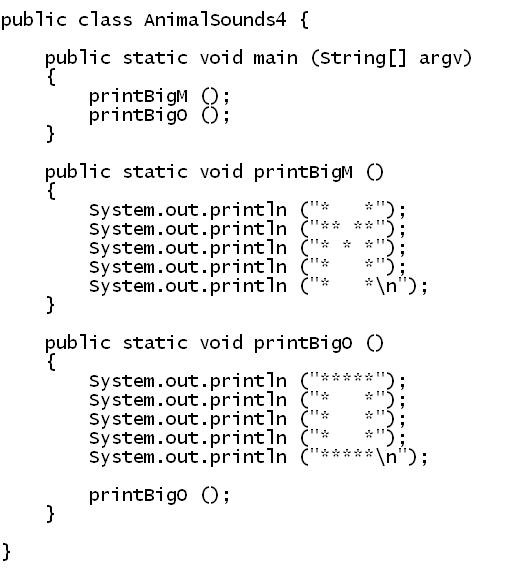
In-Class Exercise 10:
Mentally execute the above program. Which statement
gets executed in the 10-th step of execution?
What about the 15-th step?
In-Class Exercise 11:
Compile and execute the above program. What do you notice?
The term recursion is used when method calls itself:
- In the above example, it's an obvious error
=> Nothing useful is accomplished.
- Later, we'll learn to use recursion to solve problems.
- In fact, recursion is one of the most powerful
computational problem-solving paradigms.
Stopping something at the command-line with control-c:
- When something executes for too long, and you want to stop it,
type control-c (press the control key and
the c key simultaneously.
- control-c (sometimes abbreviated in discussion to
ctrl-c) is the standard way of stopping something
at the command-line.
- control-c works in all three systems: Windows, Mac,
and Unix.
In-Class Exercise 12:
Download DrawTool.java
and TestDraw.java.
Then compile and execute TestDraw, which will bring
up a window. Now go to the command-line window and type
control-c to see how it ends the program's execution.
In-Class Exercise 13:
Execute the recursive program above and stop it
during execution using control-c.