We'll make two modifications to HelloWorld:
- Change the class name to HelloWorld2
- Add another println statement.
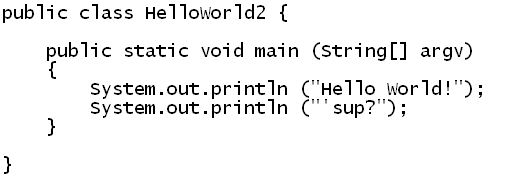
In-Class Exercise 1:
Type in the above program, but write your own
3-line greeting (3 println's,
then save the file as HelloWorld2.java,
compile and execute.
What did we learn?
- The filename needs to be the same as the class name
with .java appended.
- You can have multiple println statements,
as many as you like.
In-Class Exercise 2:
Write a program using your last name as the
class name. Your program should print
out a large 5-line version of your last name initial
using the * symbol.
For example, the program Simha.java prints
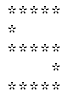
Parts of the HelloWorld program
To start to understand syntactic elements of
the HelloWorld program, let's first alternate colors
to identify ALL parts:
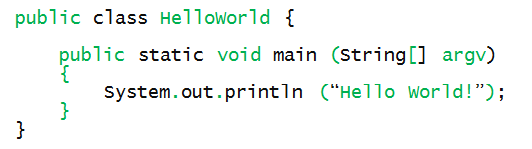
Next, we'll identify some important parts or aspects.
The class:
- Think of a class as a higher level unit,
or grouping of lower-level constructs:
public class HelloWorld {
public static void main (String[] argv)
{
System.out.println ("Hello World!");
}
}
- Here's an example with additional lower-level constructs:
public class HelloWorld {
public static void main (String[] argv)
{
printStuff ();
}
public static void printStuff ()
{
System.out.println ("Hello World!");
}
}
- Words like public and class are
called reserved words
=> come defined in the Java language and can only be used in some ways.
- Words like HelloWorld are words we pick
for our convenience
=> They are called identifiers.
- As we'll see, there are many kinds of identifiers.
- The contents of a class are the lower-level
constructs that lie between the braces that define the class.
=> Notice how the closing brace lines up under the p
of public.
- The reserved word public has to do with
visibility, an advanced topic
=> For simplicity, we'll leave everything public for now.
- Generally, you have one class per file.
- Sometimes can you have more, but it's not common, e.g,
class X {
public static void printStuff ()
{
System.out.println ("Hello World!");
}
}
public class HelloWorld {
public static void main (String[] argv)
{
X.printStuff ();
}
}
- Observe the two sets of class braces.
- If there's more than one class in a file,
only one can be public, and the
one that has main has to be the public one.
- For the most part, we'll stick to one class per file.
(And use multiple files if we have multiple classes.)
Types of brackets:
- There are four types of brackets in Java, as this example
shows:
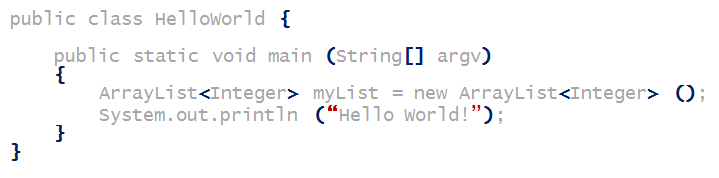
- Brackets always come in pairs: a left one and a
matching right one.
- When matching brackets have something between
the pair, think of them as "enclosing" something.
- We could consider the double-quote
" to be
a type of bracket
=> A pair of them always encloses a string.
- Note: A string is a bunch of characters
(letters, digits, symbols etc) in a row.
The level of group (or unit) below: a method
- The program below has two methods,
one called main and one called printStuff.
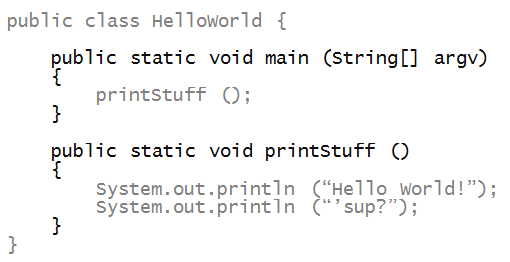
- Notice that a method "has stuff" within the braces
associated with the method.
- For now, we won't worry about the reserved words
public and static that qualify methods.
=> Most of our methods will look like this, unless we need to make a distinction.
- To understand the difference between static and non-static
methods, one needs to understand the more advanced topic of
objects. We can happily ignore that for now.
- Every method will have a name, like main
or printStuff.
- We made up the name printStuff, but
the name main is special.
=> Good practice: don't make up another method with this name.
- main is the place where execution begins.
- Every method name is followed by a pair of parentheses.
- Sometimes the parens have something between them; other times not.
- Observe that the name printStuff occurs twice
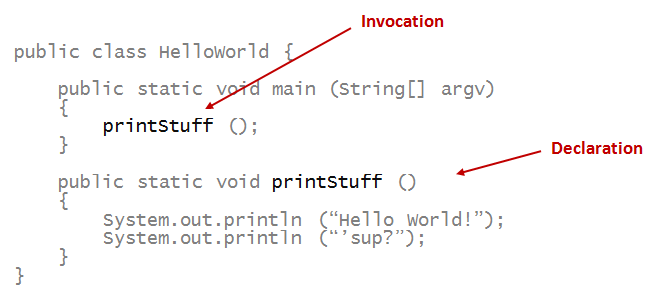
- A method is declared just once:
=> Analogy: it's a definition.
- A method can be invoked or called
any number of times.
Next, let's look at some punctuation:
- For example, in our HelloWorld program:
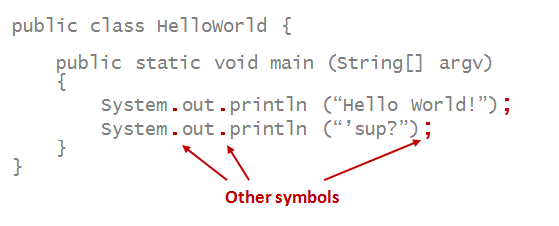
- Some of these will turn out to be operators
like + or * (multiplication).
- The semicolon is special; it is used to mark the
end of a statement.
- The "dot" operator above is used with some features
of objects.
=> We will not get deeper into this until we learn more about objects.
- For now, we will think of System.out.println
as a single entity.
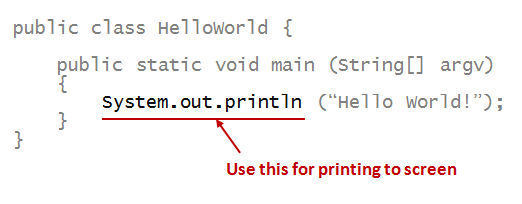
In-Class Exercise 3:
The program below has several mistakes. Can you
identify them?
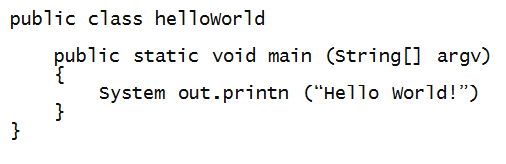
How to read programs
We can't emphasisize this enough: reading
programs is an all-important skill.
Start with the class:
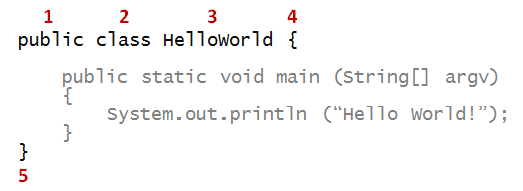
- (3) When you read the class name, pause,
and ask whether the file name matches.
- When you see the opening brace (4), immediately
look for and identify the closing brace (5).
- In general, for every left bracket, your eye should
linger on the matching right one, sort-of absorbing
the space between.
Next, go to main:
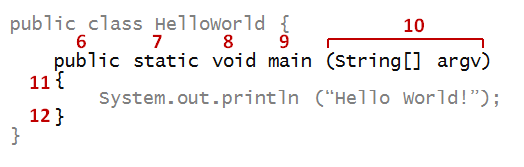
- Check to see that it's properly formed (6 - 10).
- Check the method braces (11-12) and get a sense
for how much "stuff" there is between.
Next, read the one statement inside:
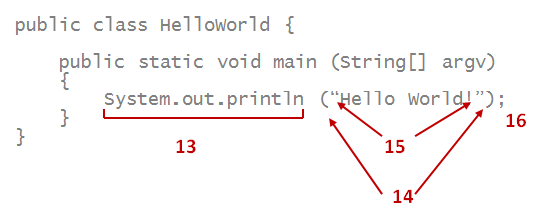
- Read System.out.println as one entity (13).
- When you see the opening paren, let your eyes
find the right one (14).
- Same thing for the double-quote (15).
- Don't forget the semicolon (16).
As we proceed, we will point out good reading habits.
In-Class Exercise 4:
As a reading exercise, go back to the two programs in
Module 0
that compute prime numbers, and apply the above ideas.
- Do the bracket-matching eye scan. How many pairs of
brackets? What are the different ways by which
the right-brackets are aligned?
- How many methods are there inside the class?
- Pay attention to indentation and semicolons. How
levels of indentation?
Do the same for the drawing programs from
Module 1: DrawTool.java
and
TestDraw.java.
Whitespace and style
About whitespace:
- Whitespace includes spaces and tabs.
- You can't insert whitespace inside words
=> The result would be treated as two words
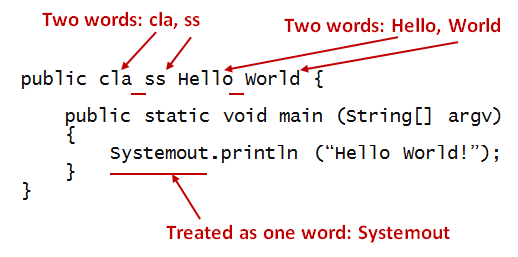
- The compiler treats contiguous characters (letters
and numbers, but not symbols) as a word.
- No whitespace is required on either side of
special symbols in Java such as the brackets, period, semicolon.
- You can't split a quoted string over two (or more) lines.
- You can insert whitespace pretty much anywhere else.
- Here's an example of HelloWorld written
with weird whitespace:
public class Whitespace
{
public
static
void main ( String [ ] argv){
System . out.println ("Hello World!");
}
}
The program compiles and runs perfectly.
Whitespace is used for style:
- Two identical programs may differ in whitespacing,
and therefore in style.
- Proper style is critical to readability.
- There is a standard style for Java.
- Some style rules:
- Indent 2 or 4 spaces when you go down one level in scope.
- Only one space between words on the same line.
- Do not use space with some operators, e.g., dot, brackets.
- Use space around others, such as =.
- You will learn about style as we proceed.
In-Class Exercise 5:
Write a (correctly compiled) HelloWorld with the least amount of
whitespace as possible. How many spaces did you use?
When things go wrong
Let's now deliberately make some mistakes:
- So that we learn what happens during compilation.
- So that we increase awareness of typical or common mistakes.
In-Class Exercise 6:
Write the HelloWorld program but save it in a file
called Wrong.java.
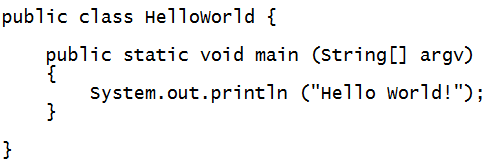
Then, compile. What do you observe?
In-Class Exercise 7:
Leave out the semicolon at the end of the println
statement. What is the compilation error?
In-Class Exercise 8:
Leave out the closing brace of main and identify
the compilation error.
In-Class Exercise 9:
Leave out the opening brace of main and identify
the compilation error.
In-Class Exercise 10:
Misspell main (say, as maine).
What do you observe?
Some observations:
- For some errors, the compiler is right on target in
identifying the error, even giving you the line number.
- For others, the compiler output is not so helpful.
=> This is unfortunately the nature of compilation.
- In some cases, there's no compiler
(javac) error, but
we see a runtime error
=> An error when we invoke java for execution.
In-Class Exercise 11:
The program below has an error:
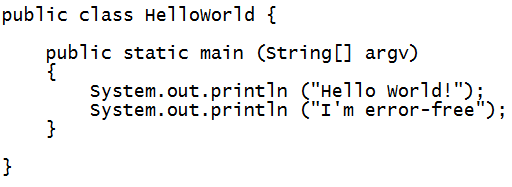
Can you identify the error just by reading (without
comparing it with the correct version)? Fix the error.
Detecting syntax errors as you construct programs:
- Through careful reading.
=> A habit worth developing.
- Type in your program and see what the compiler says.
=> Lazy approach, but always finds syntax errors.