Here is a program that reads a single integer from
the console:
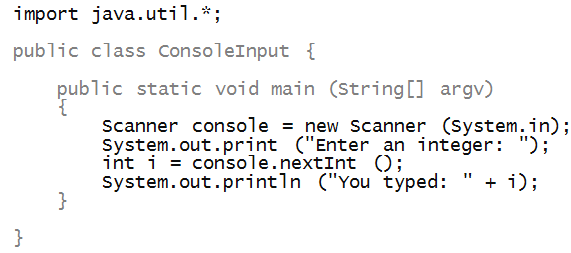
In-Class Exercise 1:
Try out the above program. What happens if you were
to hit enter instead of typing in an integer?
What happens when you type a char or double
instead? What if your integer is too big?
Let's point out a few things:
- The Scanner class is a library class in the java.util
library included in the language:

- Here, we used our variable name console:

We could have called our variable anything, like x or
commandlineWindow.
- We have written a "prompt" to the commandline window.

Notice: the use of print instead of println.
In-Class Exercise 2:
What happens when you use println instead of print?
Is it possible not to print a prompt at all? Try it.
- We used the nextInt method to get the
integer that got typed in.

- This results in the value being stored in our
variable i.
- Notice that these methods are similar to the
kinds of "object" methods for String variables:
String s = "Hello World!";
int k = s.length ();
char c = s.charAt (k-1);
String s = s.substring (0,5);
Let's now write code to input multiple integers on
a single line:

And to acquire an int and a double:

In-Class Exercise 3:
What happens if you type in two int's?
Next, a string:

- Notice that, in the output, we enclose the string in brackets.
=> This is so that it's clear what's in the string.
In-Class Exercise 4:
Type in a string with spaces on either side and in the
middle, and see what happens.
Applying Scanner to strings
The Scanner class can be applied to strings:

- Notice how the Scanner is applied to the string s.

- We changed our variable name to stringScanner,
just for clarity.

- Note: both numbers were extracted from the string s.
Thus, as an alternative to reading two numbers from
the console, we could have written:
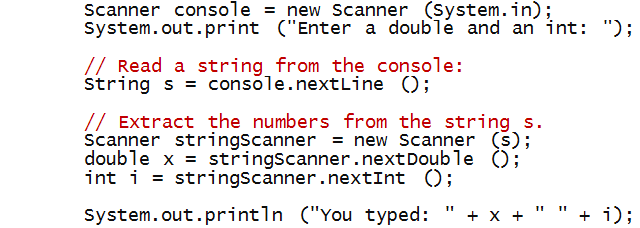
Reading from a file
We'll start by reading a single integer from a file:
Next, let's read multiple integers:
- Suppose our file has multiple integers, one per line:
1
4
9
16
25
- We will assume that we don't know ahead of time how
many lines are in the file.
- Here's the program:
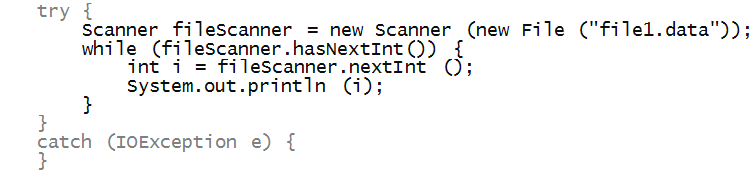
- First, we use a while loop because we
don't know how many lines the file has:

- We use the hasNextInt method (which returns
true or false) depending on whether
or not the file has more integer's remaining to be read:

- As long as there are more integers, a call to nextInt
method will extract the next one.
- When there are no more integers, nextInt returns
false and execution goes past the while-loop.
Reading from a file - more examples
Let's now read a bunch of (x,y) coordinates
from a file.
In-Class Exercise 5:
What happens if there are more than 5 points in the file but
the first line still has 5? What if the first line has 5,
but there are fewer than 5 points in the file?
In-Class Exercise 6:
Rewrite the above using a while-loop instead of a for-loop.
Next, let's consider the case where we don't
know how many points are in the file:
- Suppose our data looks like this:
1.0 1.0
2.0 4.0
3.0 9.0
4.0 16.0
5.0 25.0
- What we need to do: first count how many lines, then read the
lines one by one.
- We'll need to do a full read of the file to count the
number of lines.
- And then, read it all over again to read the points.
- Here's the program:
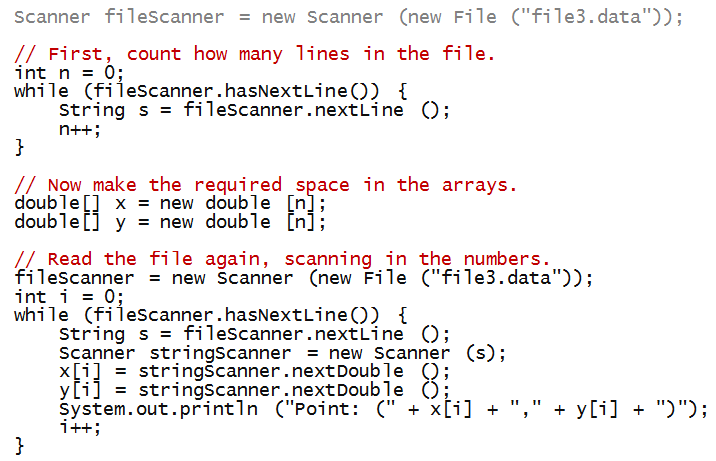
- Notice that we first read line-by-line (just
as strings, without looking for numbers), just to count the
number of lines:
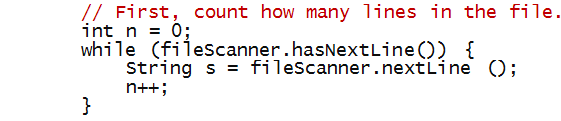
- Once we know how many points, we can size the array:

- After which, we read the points into the arrays.
- To start again, we "re-create" the Scanner but
using the same variable:

Let's examine one more way to read the points:
- We'll read lines as strings, and then use a String scanner.
- Here's the idea:
1. Count the number of lines, n
2. Make the arrays of size n
3. In a for or while loop {
4. Read a line as a string
5. Extract the numbers from the string
6. Put the numbers in the array.
7. }
- Here's the program (showing the code in the try clause):

- Notice that we use a fresh new scanner for each string
that is read line-by-line:
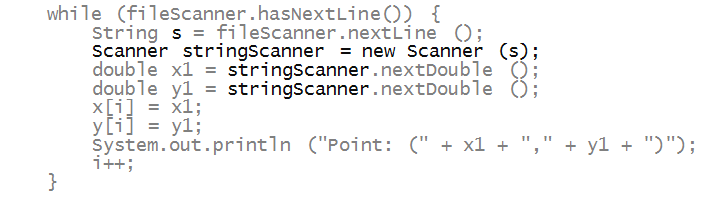
- When do we do this, as opposed to read numbers directly?
=> When each line could possibly be different, or have different data.
In-Class Exercise 7:
Add a println inside both while-loops to print the strings.
In-Class Exercise 8:
Re-write the second while-loop as a for-loop.
Writing to a file
To demonstrate writing, let's read from one file
and write to another, in effect, make a copy of the first file.
Let's now add code to take the filenames from the commandline.
Before that let's understand how commandline arguments work:
- Consider this program:

In-Class Exercise 9:
Run the program - what is the output?
In-Class Exercise 10:
Now run the program with some commandline arguments such as:
java CommandLineArguments hello world!
What is the output? Try more strings after CommandLineArguments.
File copy via commandline arguments:
- What we'd like to do is imitate file copying on Unix
at the commandline:
java Copy file3.data file4.data
This should copy from file3.data into a new file
called file4.data.
- We have already written the copying part.
- All that's left is extracting the names from the
commandline
=> We've seen how to do this from argv.
- Here is the program:
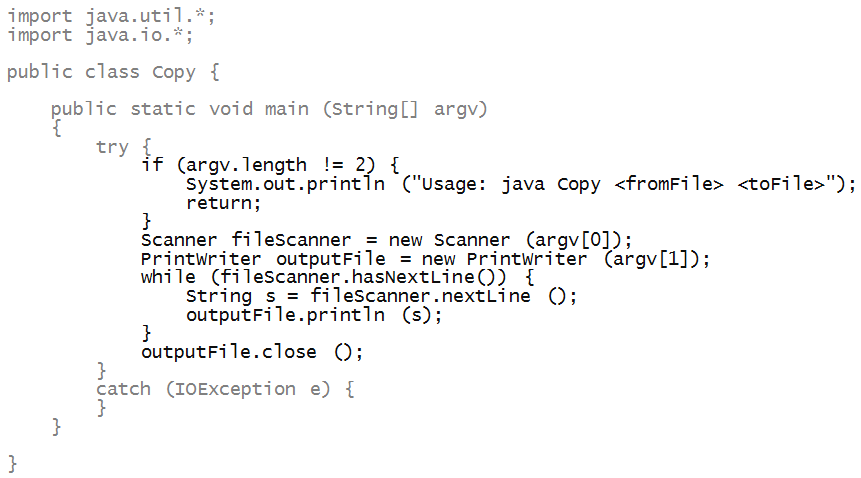
- Notice that we expect the "from" file's name to be in
argv[0] and the "to" file's name in argv[1].
- Of course, the user may not type in the correct
number of arguments
=> This is why we check that there are exactly two arguments.
In-Class Exercise 11:
Try it with the wrong number of arguments. What gets printed out?