As you saw in class, even the A* planner takes a long
time for some goal-configurations in the arm problem.
In this assignment, you will explore the idea of storing
pre-computed plans and using them. The idea is this:
if we are able to pre-compute and store k plans, then
the end-configurations of those plans can each be used as
starting points for an altogether new goal. The thinking is,
perhaps one of those end-configurations is close to the
goal, in which case a plan can be generated quickly
by combining the pre-computed plan with the plan that starts
with the end-configuration of the pre-computed plan.
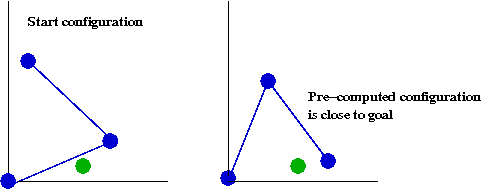
In the picture above, we are given a start configuration (left)
and a goal (in green). If we had already pre-computed a few
plans, one of them could be the configuration at the right.
This means we know how to get to that configuration quickly
from the start configuration. Thus, in a new problem, we
can start the search from there, compute the steps
to the goal node, and add these steps to the pre-computed plan.
- Modify the A* algorithm to compute k=5 stored plans.
Naturally, you ought to pick these plans carefully so that
they, in a sense, "cover" the range of difficult situations.
- Then select a few new targets and compare regular A*
with your new algorithm, which we'll call pre-A*.
How will you choose which pre-stored plan to use?
Alternatively, you could run all 5 of them (by stepping
through each in turn).
How often does pre-A* outperform A*?
- [Optional for undergrads] Experiment with k
and implement a parallel search, in which you treat each
pre-stored plan as a separate search from that starting point,
stepping through each in turn.
At what values of k does the parallel-search become
worse than the single run of A*? To make a fair comparison,
add up the total time for a number of different goal states.
- [Optional for undergrads] Use an arm with 4 links instead of 3.
How does this change your findings? You can change the number
of links by setting
numLinks=4 in
ArmProblem.java.