Lecture Notes 10: Advanced Methods and Introduction to Conditionals
Objectives
By the end of this module, for simple programs with real
numbers, you will be able to:
- Evaluate Boolean expressions.
- Construct Boolean expressions from
English descriptions.
- Mentally execute code with if,
if-else, and if-multi-else statements.
- Write and debug code with conditionals.
- Identify new syntactic elements related to the above.
Before Starting
- Read Sections 10.01 to 10.03 in the Codio Course
If you do not have your Codio course ready, use any text editor or simple IDE. Some possibilities are:
- Write using Sublime; Compile and run in the Terminal
- Use IDEs like: IntelliJ, DrJava, or JGrasp
Catching Up
Before we move forward, let's catch up (complete any remaining work from the previous module)
In this case, make sure we've got:
- String API: Searching the API and implementing combinations of loops and API calls to achieve complex string manipulation.
Writing and using Methods that use Input and/or return Output
In the previous module, we practiced using methods that resolve (or return) something and that might need input parameters to run.
In this segment we'll practice writing and invoking such methods.
First, two definitions:
- Parameters are those specified in the Method Declaration as being required as input for the method to function
.
- Arguments are the actual explicit values or variables whose values we want to pass to the method by putting the inside the parenthesis when doing the method Invocation.
Look at this program:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18 |
public class IOMethods1
{
public static void main(String args[])
{
int num1 = 5;
int num2 = 13;
// Invoke the myOperation method and save result in the num3 variable
int num3 = myOperation(num1, num2); // myOperation(num1, num2) resolves into the resulting value
System.out.println ("myOperation on " + num1 + " and " + num2 + " is: " + num3);
}
public static int myOperation(int localNum1, int localNum2)
{
int localNum3 = (localNum1 * localNum2) - (localNum1 - localNum2);
// this causes the method invocation to resolve into localNum3
return localNum3;
}
}
|
Note the following:
- Since the method does not know how it will be invoked, it has default names for the input parameters. For example, it could be called like this:
- int z = myOperation (8,2);
And it has to place the arguments 8 and 2 somewhere!
- The parameters that the method myOperation takes are two ints that will be locally called localNum1 and localNum2 (but that you could rename to whatever you want as long as you use them properly inside the method).
- When we invoke the method, the argument values are copied from the invocation arguments to the method's input parameters.
That is, from num1 to localNum1, and from num2 to localNum2.
- The local method variables are only "alive" during the execution of the method. Once the method returns, those variables are no longer in existence.
Activity 1:
In
IOMethods1.java
write the above program and see what gets printed.
Now, let us run it inside the
Java Visualizer to understand the way these parameters are passed around.
Activity 2:
In
IOMethods1.java
inside the method
myOperation, modify the values of localNum1 and localNum2 before returning localNum3 by using these two lines:
localNum1+=99;
localNum2+=99;
then, inside the main, add the following statement:
System.out.println ("num1 after modifying localNum1 is: " + num1 );
System.out.println ("num2 after modifying localNum2 is: " + num2 );
What do you print? why?
Now Look at this program:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30 |
public class IOMethods2
{
public static void main(String args[])
{
String base = "Pizza should not have pineapple";
String part = "pine";
// Invoke the extract method and save result in out variable
String out = extract(base, part); // extract(base, part) resolves into the resulting string
System.out.println ("The new String is: " + out);
}
public static String extract(String localBase, String localPart)
{
// The return variable
String localOut = "";
// Get the index of localPart inside localBase
int idx = localBase.indexOf ( localPart );
// Get the size of localPart
int partSize = localPart.length();
// copy localBase up to the start of localPart
localOut = localBase.substring (0,idx);
// copy localBase after localPart until the end of localBase
localOut = localOut + localBase.substring (idx+partSize);
return localOut;
}
}
|
Activity 3:
In
IOMethods2.java
write the above program and see what gets printed.
Now, let us run it inside the Java Visualizer to understand the way these parameters are passed around.
Note the following:
- Since the method does not know how it will be invoked, it has default names for the input parameters. For example, it could be called like this:
- String out = extract ("catastrophe","cat");
- The parameters that the method extract takes are two Strings that will be locally called localBase and localPart.
- When we invoke the method, the values inside the calling arguments are copied into the input parameters.
- However, since base and part are Strings, which are Reference types the contents of those variables are references to where the object is located!! so we actually end up copying the references, NOT the values.
This is called "passing By reference"
- One last thing is: as we mentioned, once created, Strings cannot be modified. They are called Immutable objects. So, if we modify the String inside the called method, the local variable will point to a newly created String. Let's see that example below:
Activity 4:
In
IOMethods2.java
inside the method
extract, modify the values of localBase and localPart before returning localOut by using these two lines:
localBase = "New Base";
localPart = "New Part";
then, inside the main, add the following statement:
System.out.println ("base after modifying localBase is: " + base );
System.out.println ("part after modifying localPart is: " + part );
What do you print? why?
Activity 5:
In IOMethods3.java
Write a method that takes no parameters but returns a value. Then invoke that method from main and print the return value.
Activity 6:
In IOMethods4.java
Write a method that takes one String parameter (called text), one integer parameter (called idx), and one char parameter (called ch).
The method should construct a new String which is a version of the String text with the character at index idx replaced with the character ch. Then invoke that method from main and print the return value.
Conditionals!! ... A simple example
Consider this program:
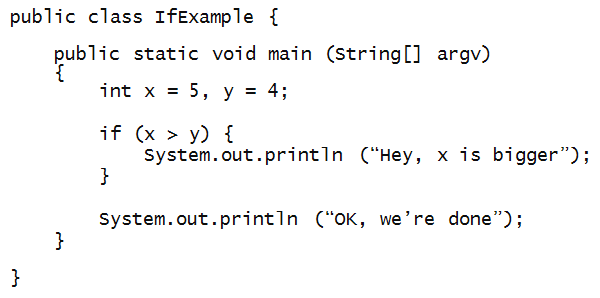
Activity 7:
In MyIfExample.java,
add an additional println right below the "Hey, ..." println.
Compile and execute the program. Then, change
the value of y to 6 and compile/execute.
What is the output?
About the if-statement:
- The if-statement above consists of several parts:
- The reserved word if:

- The condition (x>y)

- The block of code associated with the if:

- Execution:
- The condition (x>y) is evaluated.
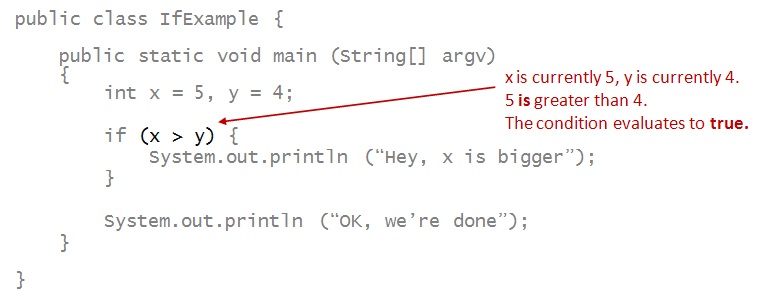
- If it turns out to be true at the moment the
condition executes,
the statements inside the if-block are executed.
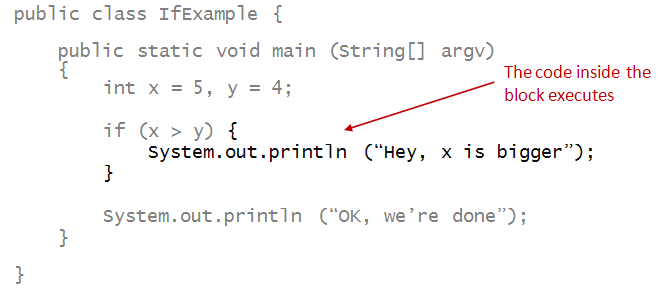
- Then, execution continues after the block:
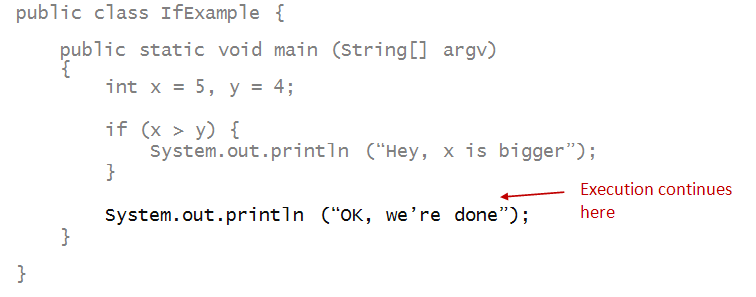
- If such a condition is not true, execution jumps to just
after the if-block.
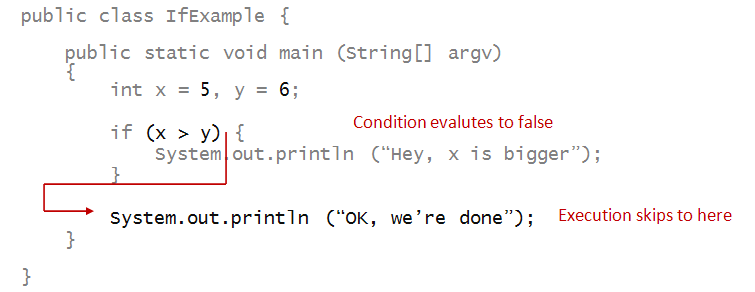
Activity 8:
Consider this (part of a) program.
Try to mentally execute and identify the output
before confirming in
MyIfExample2.java,
int s = 0;
for (int i=1; i<=5; i++) {
s = s + i;
}
if (s < 15) {
System.out.println ("Less than 15");
}
System.out.println ("End");
If-else
Consider this program:
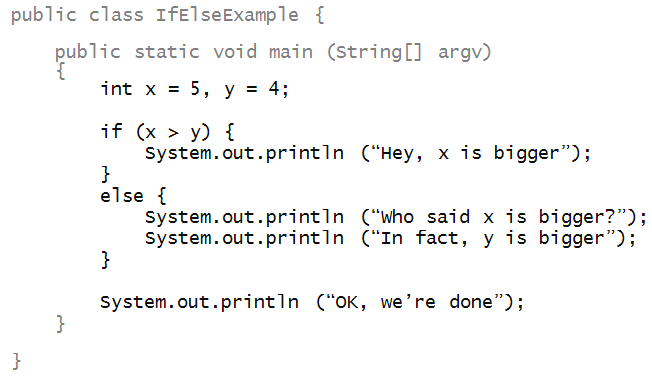
- If the if-condition (x>y) evaluates to true,
the if-block executes:
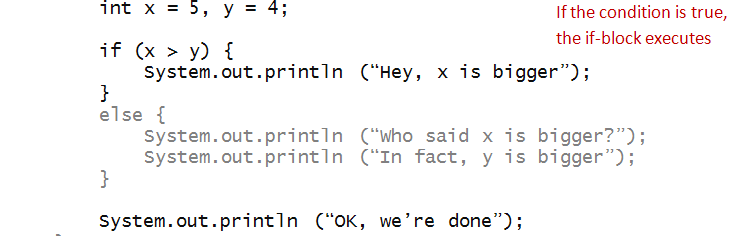
- Otherwise, the else block executes:
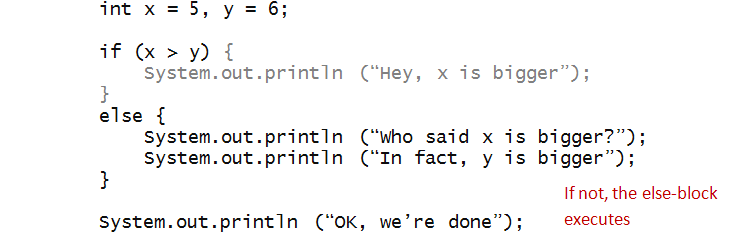
We can combine an
else clause with an added
if-clause:
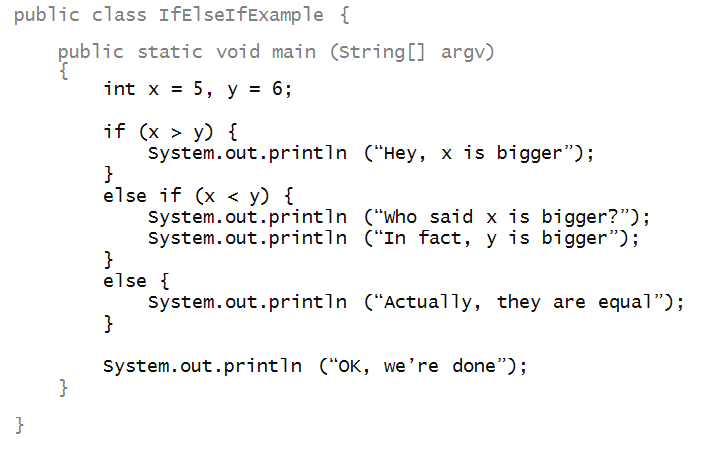
- If the if condition is true, only the if-block
executes:
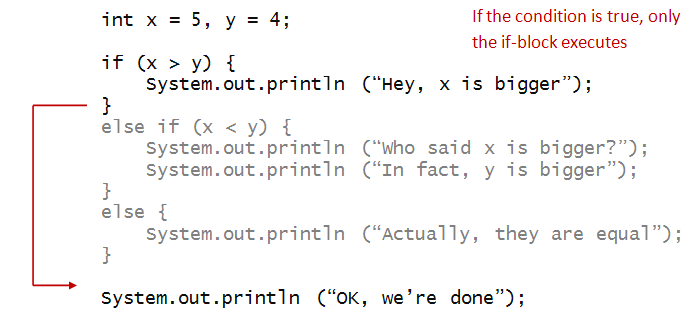
- If not, the else-if condition is evaluated:
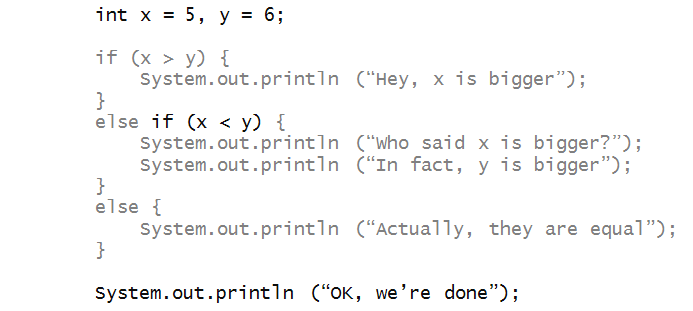
- If that's true, the appropriate block is executed:
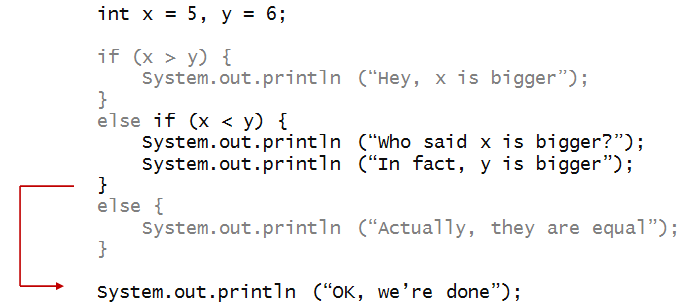
- If the second if-condition is false, the last else-clause
executes:
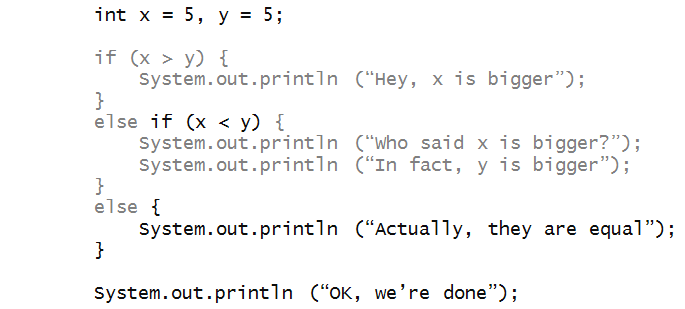
Activity 9:
Type up the above example in
IfElseExample.java,
and change the values of x one at a time so that you see
each block execute. That is, try one value of x to make
the if-block execute, then another value of x to make the
else-if block execute etc.
Comparison operators
:
- These are Java's comparison operators:
(x < y) // Strictly less than.
(x <= y) // Less than or equal to.
(x > y) // Strictly greater than.
(x >= y) // Greater than or equal to.
(x == y) // Equal to.
(x != y) // Not equal to.
- Thus, in the above example, we could have written:
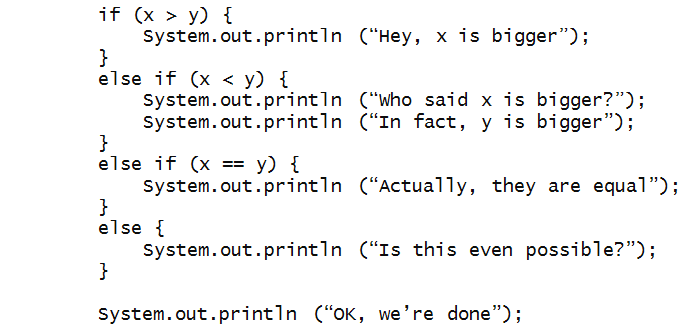
Activity 10:
Are there any circumstances under which the last block executes?
- Here, logic dictates that only some blocks can
possibly execute.
- Thus, sometimes it is not necessary to write some blocks
of code if they'll never execute.
Activity 11:
Suppose we want to identify whether
x has
the largest value among
x, y and
z.
Why doesn't the following program work?
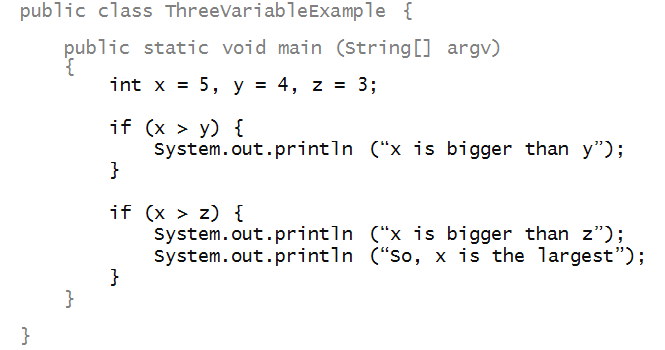
Can you alter the program
(in MyThreeVariableExample.java)
to make it work?