Lecture Notes 4: Decision Making and Conditional Statements
Objectives
By the end of this module, for simple programs with real
numbers, you will be able to:
- Evaluate Boolean expressions.
- Construct Boolean expressions from
English descriptions.
- Mentally execute code with if,
if-else, and if-multi-else statements.
- Write and debug code with conditionals.
- Write and debug code with nested conditionals.
- Identify new syntactic elements related to the above.
Boolean variables
In order to make a decision, we need a way to represent a situation that is true,
versus one that is false. In Java, there is a special type used for just that: boolean.
A boolean variable (or expression) has either the value true or the value
false -- both of these are reserved words in the language:

With the compiler recognizing boolean types and expressios, it is now possible to
have the program make decisions at runtime, based on the values stored in variables.
We achieve this through the use of conditional statements.
Why are conditionals useful in computation?
- Classification of outcomes, such as an online symptom checker
- Choose-your-own-adventure style games and products
- Conditional logic is THE big difference between something like a calculator vs your computer
- Other examples?
Conditionals ... a simple example
Consider this program:
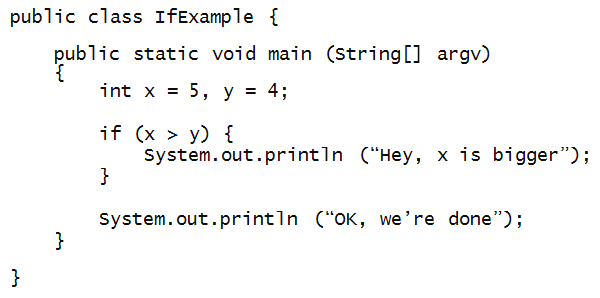
About the if-statement:
- The if-statement above consists of several parts:
- The reserved word if:

- The condition (x>y)

- The block of code associated with the if:

- Execution:
- The condition (x>y) is evaluated.
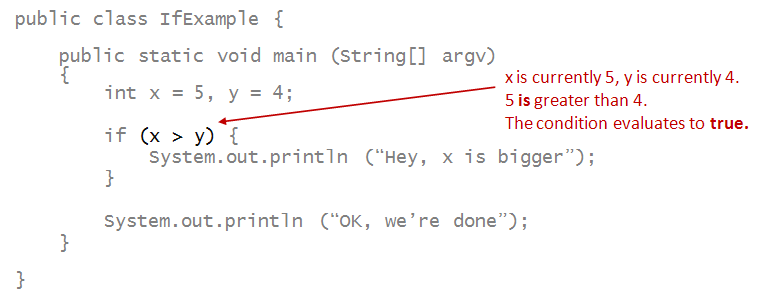
- If it turns out to be true at the moment the
condition executes,
the statements inside the if-block are executed.
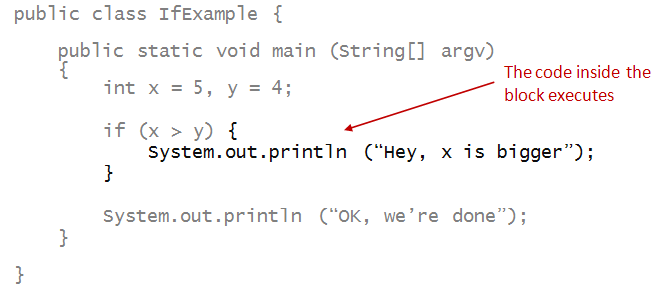
- Then, execution continues after the block:
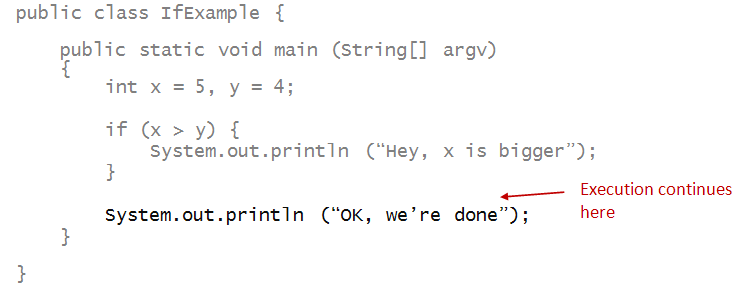
- If such a condition is not true, execution jumps to just
after the if-block.
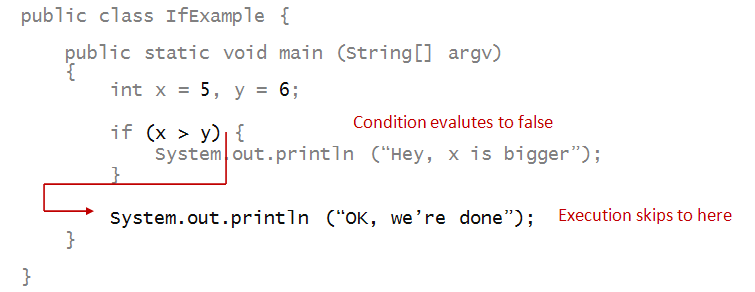
If-else
Consider this program:
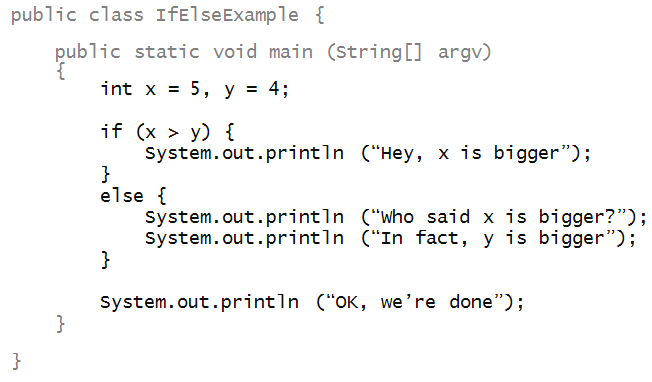
- If the if-condition (x>y) evaluates to true,
the if-block executes:
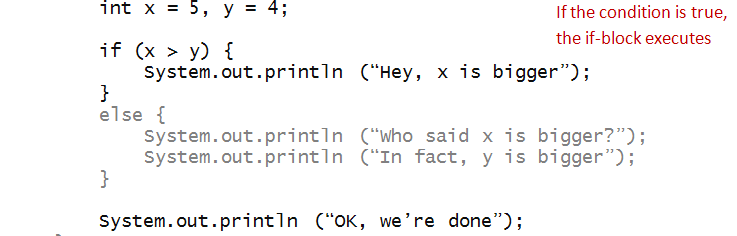
- Otherwise, the else block executes:
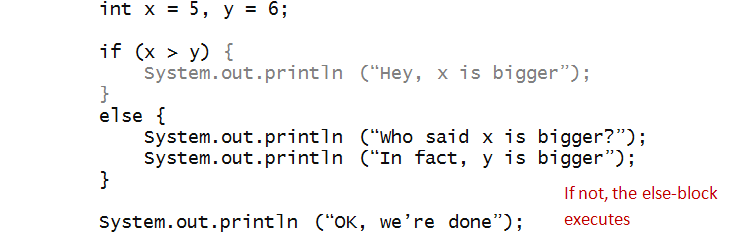
We can combine an
else clause with an added
if-clause:
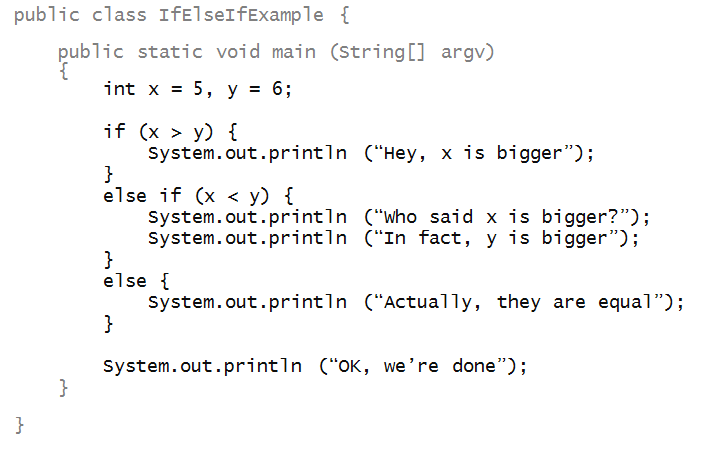
- If the if condition is true, only the if-block
executes:
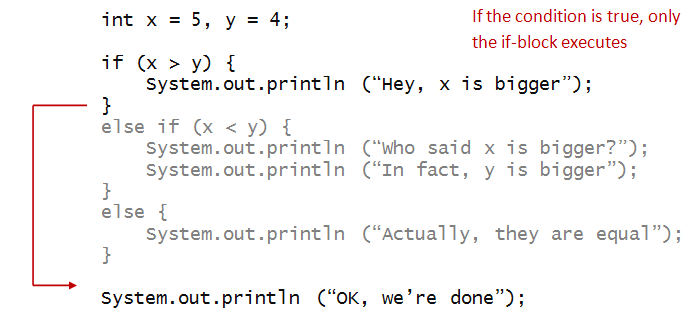
- If not, the else-if condition is evaluated:
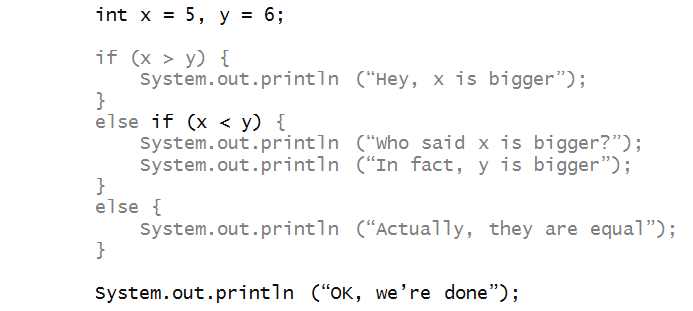
- If that's true, the appropriate block is executed:
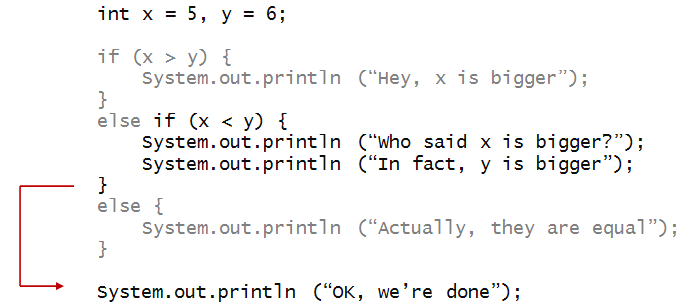
- If the second if-condition is false, the last else-clause
executes:
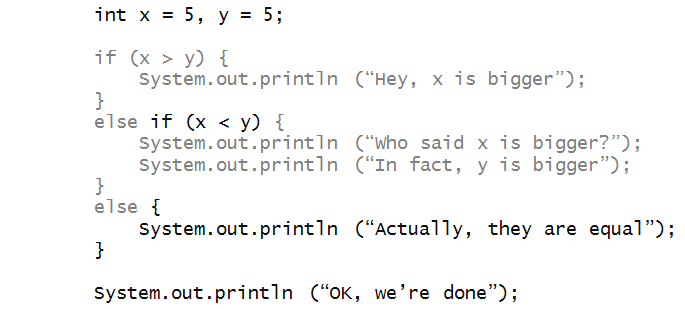
Activity 1:
Run
the above example in
and change the values of x one at a time so that you see
each block execute. That is, try one value of x to make
the if-block execute, then another value of x to make the
else-if block execute etc. Then, modify the code by adding a new
variable,
z
, and include a conditional that should
evaluate to
true
before the
else
clause.
How does it evaluate?
Note that, in a single if-else block (which can have more than two
clauses),
one and only one clause executes, even if multiple clauses
happen to be true. The first clause to evaluate to true is the only
one that gets executed.
Comparison operators
:
- Recall, these are Java's binary comparison operators:
(x < y) // Strictly less than.
(x <= y) // Less than or equal to.
(x > y) // Strictly greater than.
(x >= y) // Greater than or equal to.
(x == y) // Equal to.
(x != y) // Not equal to.
- Thus, in the above example, we could have written:
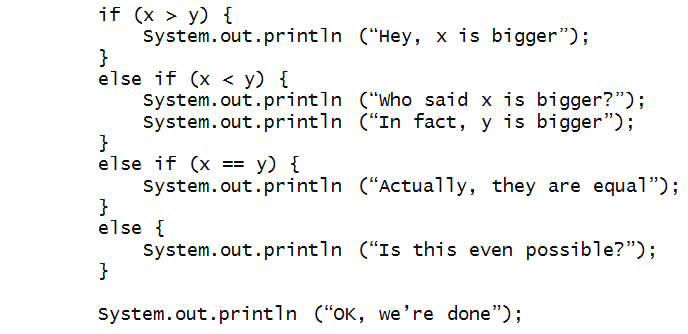
Activity 2:
Are there any circumstances under which the last block executes?
- Here, logic dictates that only some blocks can
possibly execute.
- Thus, sometimes it is not necessary to write some blocks
of code if they'll never execute.
Activity 3:
Suppose we want to identify whether
x has
the largest value among
x, y and
z.
Why doesn't the following program work?
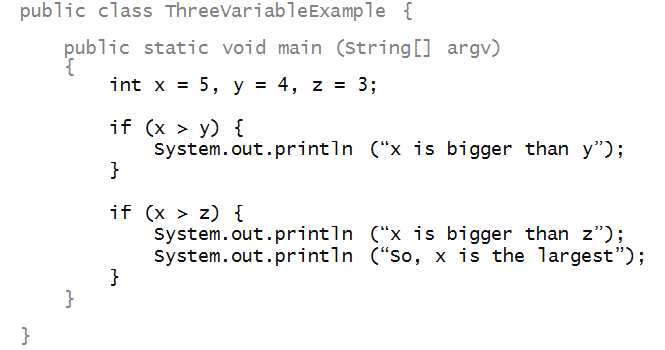
How would you alter the program
(in ThreeVariableExample.java)
to make it work?
Nested conditionals
Consider this program:
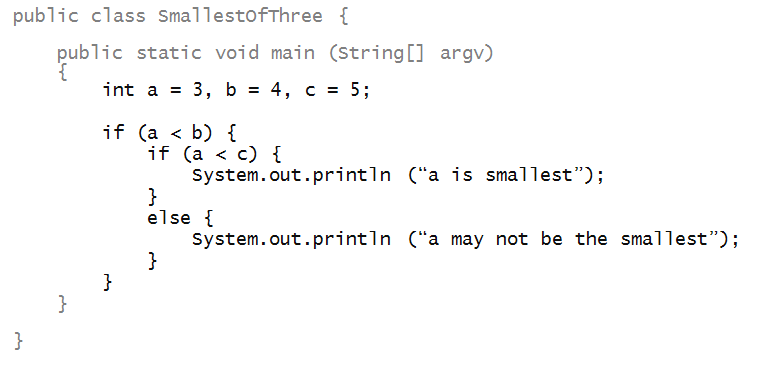
- First, the outer if condition is evaluated:
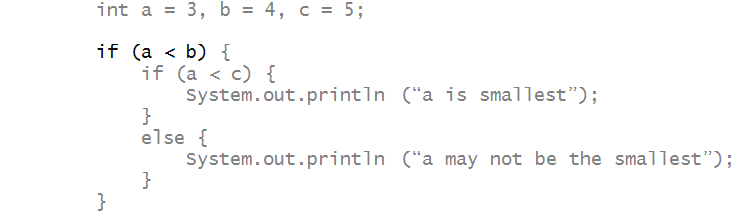
- Since that's true here, we get inside the if block:
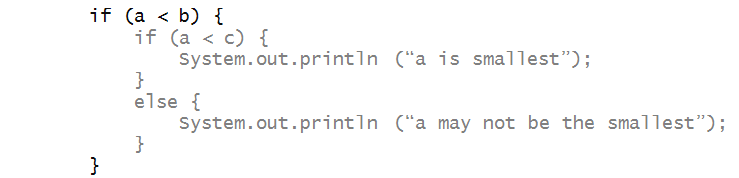
- The next thing to be executed is the inner if condition:
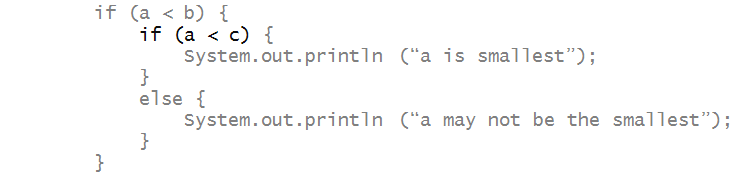
- Since that's true here, we get inside that if-statement's
if-block:
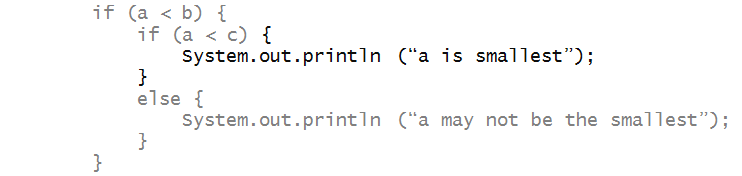
- Note how execution goes from there:
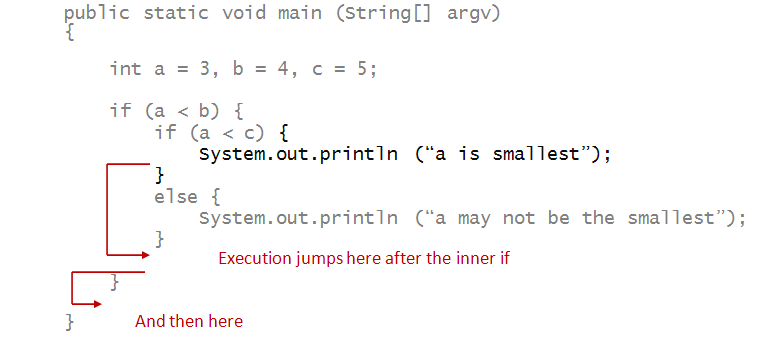
Combining conditions
Consider this program:
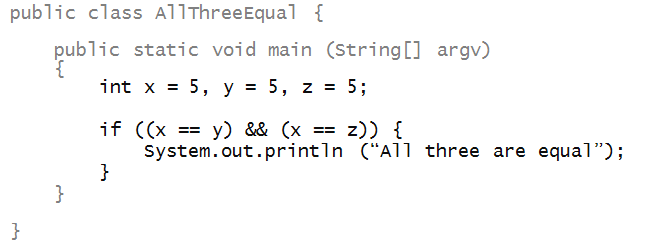
- The if condition combines two comparisons:

- The combination uses the Boolean operator &&

The AND operator
- The "AND" operator works like this:
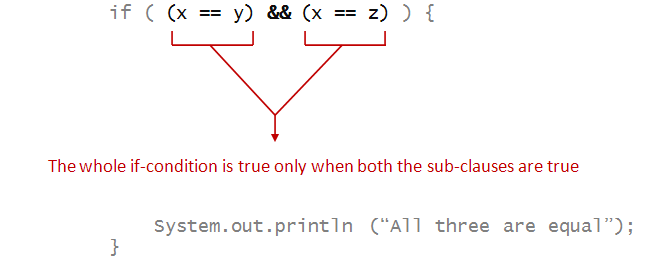
- The overall combination evaluates to true only when BOTH the individual conditions are true.
- Java uses a short-cut for efficiency purposes, that if the first condition evaluates to
false, it doesn't bother evaluating the second condition (because it's value
won't change the outcome of the expression overall). This is known as short-circuiting.
The OR operator
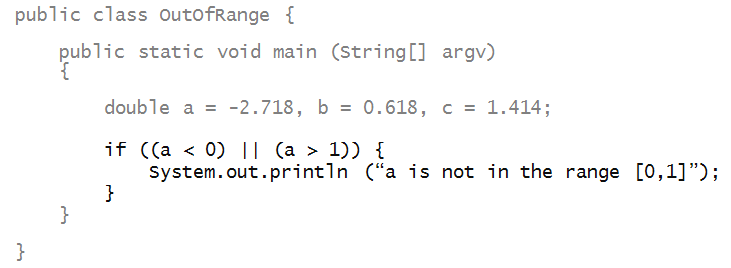
- Here, the || operator should be read as "OR".
- If either subclause is true, the whole is true:
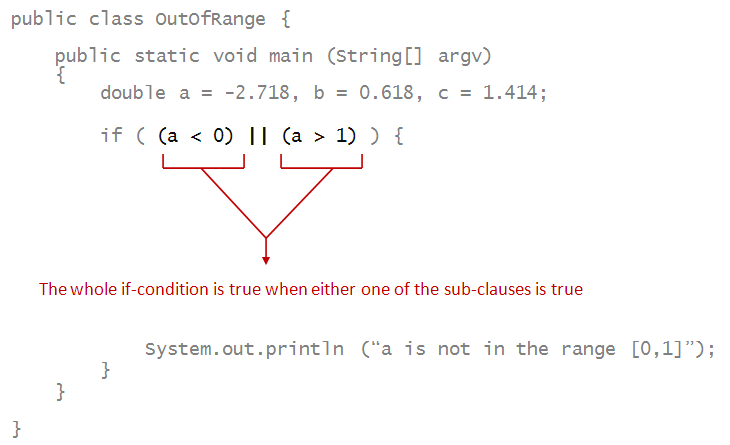
- Note: both sub-clauses can be true, which would make the
whole expression true.
- Again, Java uses short-circuiting for efficiency purposes here as well with ||,
that if the first condition evaluates to
true, it doesn't bother evaluating the second condition (because it's value
won't change the outcome of the expression overall).
The NOT operator
The NOT operator can be applied to a larger clause
made of sub-clauses. Here, the inner clauses are first evaluated, and the
result is "flipped" to see if the NOT clause turns out to be true:

Activity 4:
Suppose integer variables
a,b,c,d,e
have values a=1, b=1, c=3, d=4, e=5.
Consider the following three expressions:
( (a <= b) && (c+d > e) && (d > 1) )
( (a > c) || ( (c+1 < e) && (c-b > a) ) )
! ( (b == d-c) && (a > b) || (c < d) )
Try to evaluate each expression by hand.
Use the Java Visualizer to see if the
result matches with your hand-evaluation.
Boolean operator summary
We can capture the behavior of the and, or, and not operators using a truth table:
x
|
y
|
x && y
|
x || y
|
!x
|
True
|
True
|
True
|
True
|
False
|
True
|
False
|
False
|
True
|
False
|
False
|
True
|
False
|
True
|
True
|
False
|
False
|
False
|
False
|
True
|
When things go wrong
Activity 5:
Identify the four errors in this piece of code:
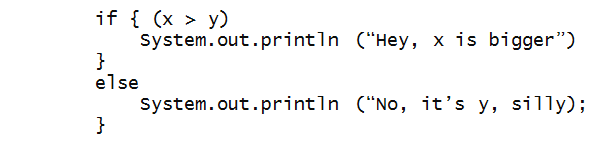
Activity 6:
Fix the errors in the if condition to make
this print "Success".
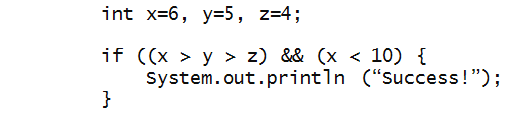
First, try to find the problems without compiling.
Then, fix the code
and see if you were right while reading.
Meta
This has been a challenging module:
intricate code, with many moving parts, and looooong.
A few things to keep in mind:
- It is exactly this type of intricate
detail that needs to be mastered and is critical to developing "code-like thinking".
- It is natural and perfectly acceptable to feel overwhelmed by the sheer amount of detail. All those traces!
- At the present moment it will certainly feel that way.
- By the end of the course, you will be able to come back to this module and feel a lot better about it.
- Many modules later, this will all seem rather easy, and you'll wonder why you ever thought it was tough.
- This is simply the nature of how complex skills are learned.
What's in our toolbox at this point:
Our current toolbox by this lecture
Next class:
We'll go through some more in-class exercises on conditionals and decision making.
Assignments for next lecture:
None.