By the end of this module, for simple programs,
you will be able to:
- Evaluate Boolean expressions.
- Construct Boolean expressions from
English descriptions.
- Mentally execute code with if,
if-else, and if-multi-else statements.
- Write and debug code with conditionals.
- Write and debug code with conditionals inside loops.
- Declare and use Boolean variables.
- Identify new syntactic elements related to the above.
A simple example
Consider this program:
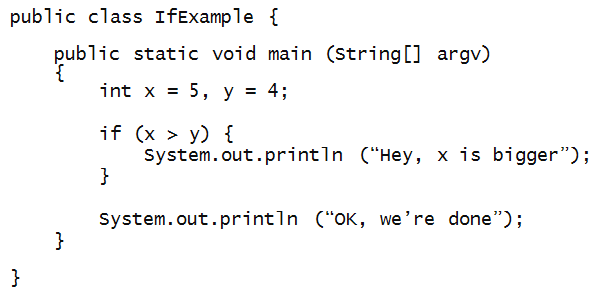
In-Class Exercise 1:
Add an additional println right below the "Hey, ..." println.
Compile and execute the program. Then, change
the value of y to 6 and compile/execute.
What is the output?
About the if-statement:
- The if-statement above consists of several parts:
- The reserved word if:

- The condition (x>y)

- The block of code associated with the if:

- Execution:
- The condition (x>y) is evaluated.
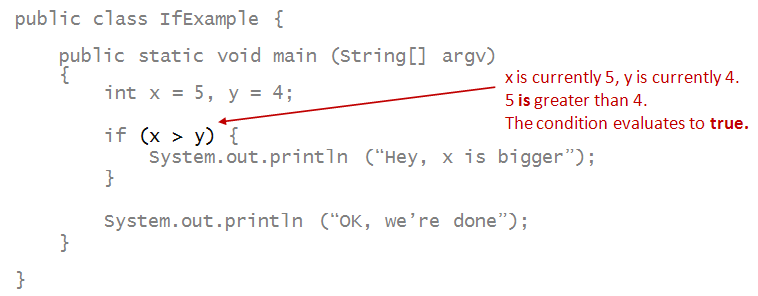
- If it turns out to be true at the moment of execution,
the statements inside the if-block are executed.
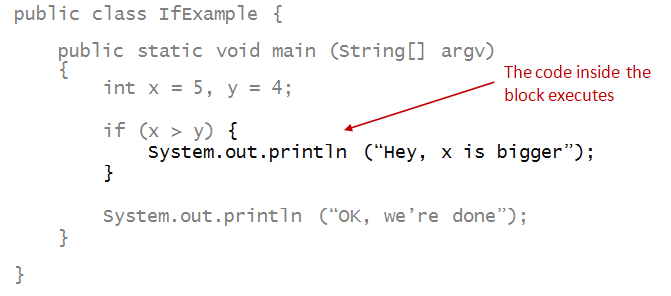
- Then, execution continues after the block:
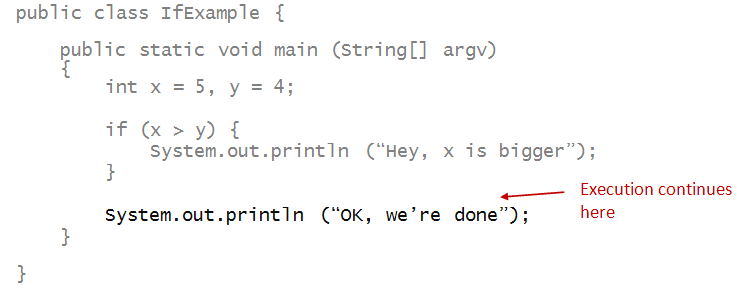
- If such a condition is not true, execution jumps to just
after the if-block.
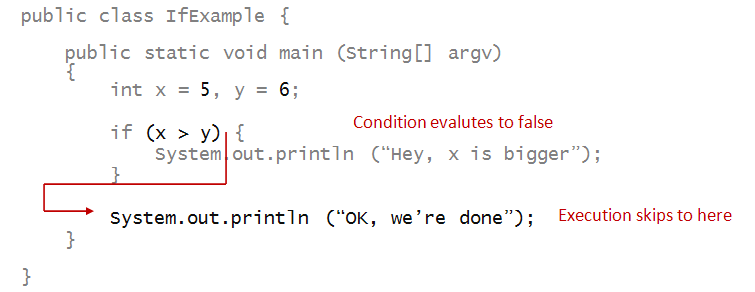
If-else
Consider this program:
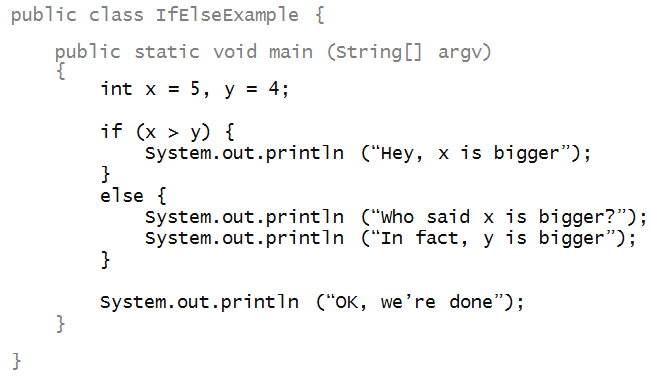
- If the if-condition (x>y) evaluates to true,
the if-block executes:
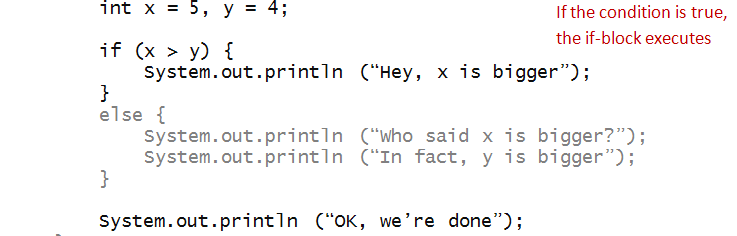
- Otherwise, the else block executes:
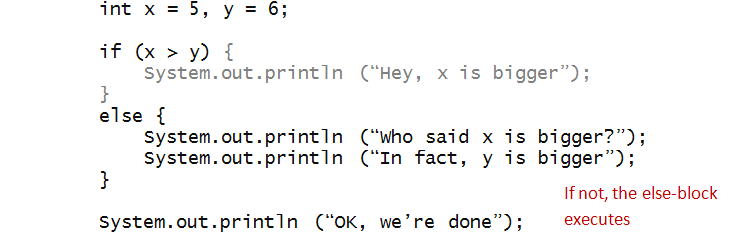
We can combine an else clause with an added if-clause:
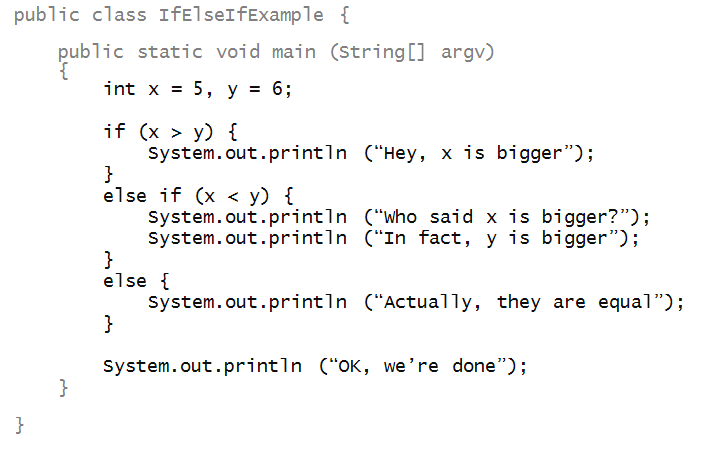
- If the if condition is true, only the if-block
executes:
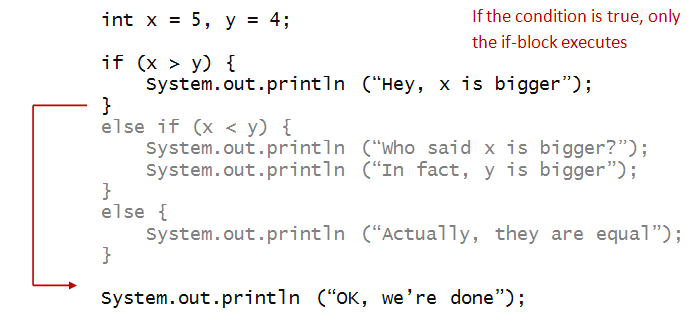
- If not, the else-if condition is evaluated:
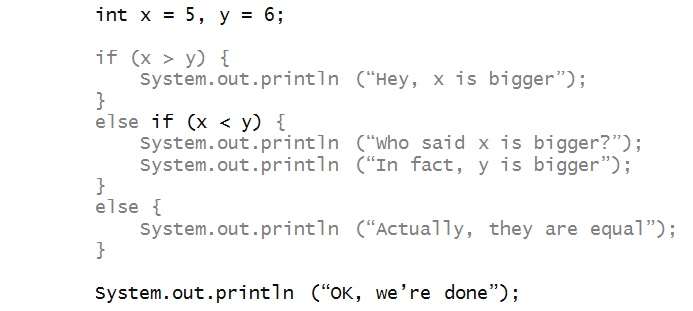
- If that's true, the appropriate block is executed:
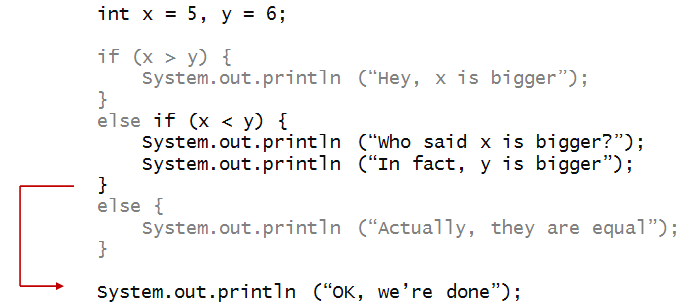
- If the second if-condition is false, the last else-clause
executes:
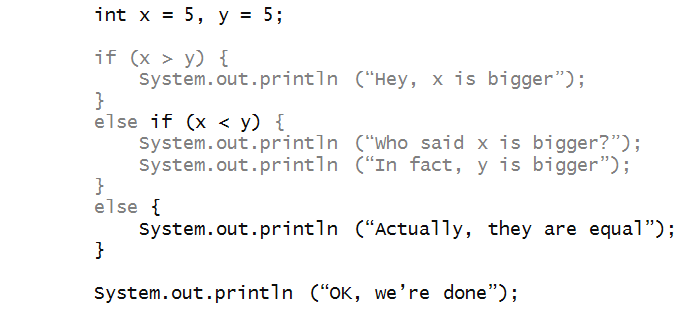
Comparison operators:
- These are Java's comparison operators:
(x < y) // Strictly less than.
(x <= y) // Less than or equal to.
(x > y) // Strictly greater than.
(x >= y) // Greater than or equal to.
(x == y) // Equal to.
(x != y) // Not equal to.
- Thus, in the above example, we could have written:
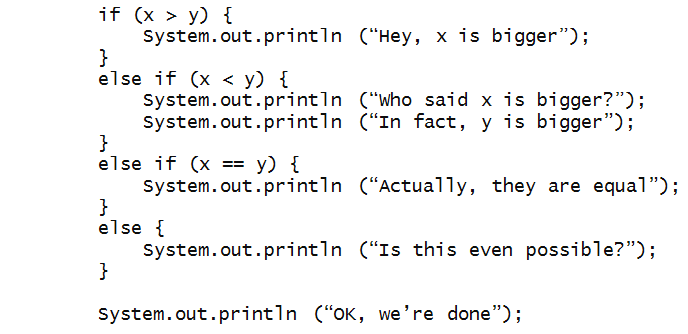
In-Class Exercise 2:
Are there any circumstances under which the last block executes?
- Here, logic dictates that only some blocks can
possibly execute.
- Thus, sometimes it is not necessary to write some blocks
of code if they'll never execute.
In-Class Exercise 3:
Suppose we want to identify whether x has
the largest value among x, y and z.
Why doesn't the following program work?
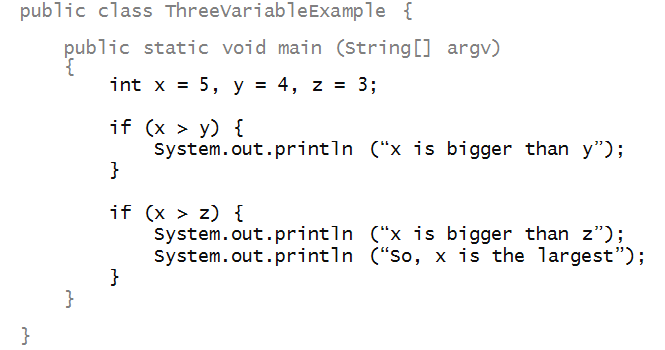
Can you alter the program to make it work?
Nested conditionals
Consider this program:
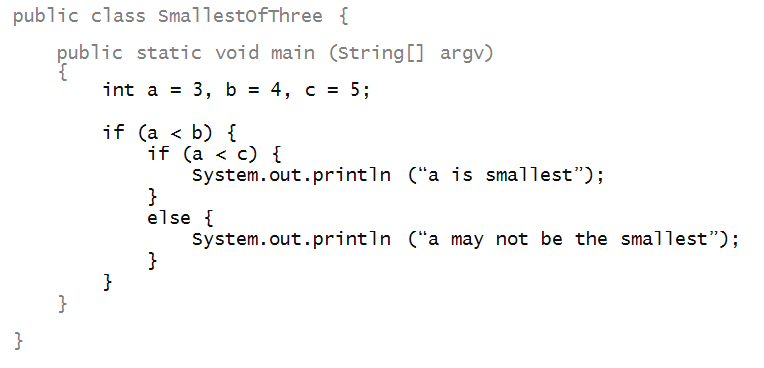
- First, the outer if condition is evaluated:
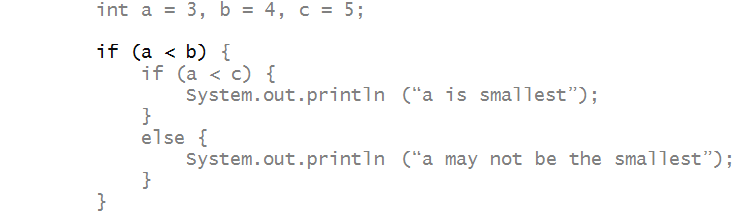
- Since that's true here, we get inside the if block:
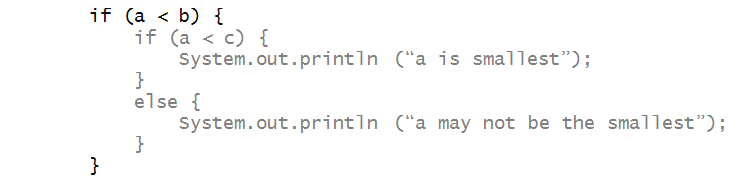
- The next thing to be executed is the inner if condition:
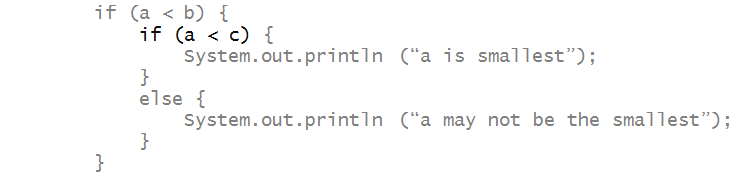
- Since that's true here, we get inside that if-statement's
if-block:
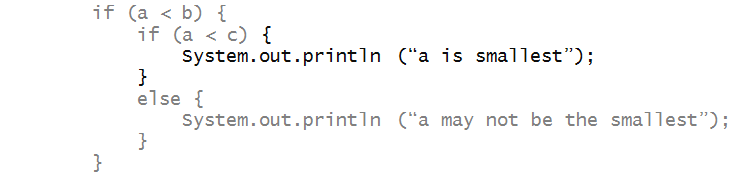
- Note how execution goes from there:

In-Class Exercise 4:
Modify the above program so that it prints out,
appropriately, one of "a is the smallest", "b is
the smallest" or "c is the smallest", depending
on the actual values of a, b and c.
Try different values of these variables to make
sure your program is working correctly.
Combining conditions
Consider this program:
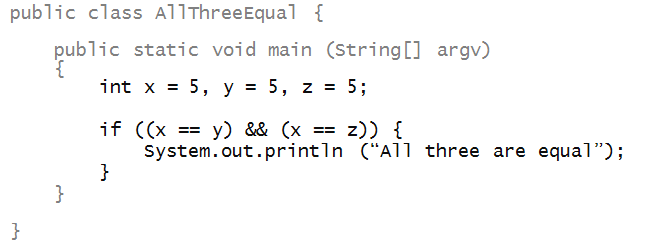
- The if condition combines two comparisons:

- The combination uses the Boolean operator &&

- The "AND" operator works like this:
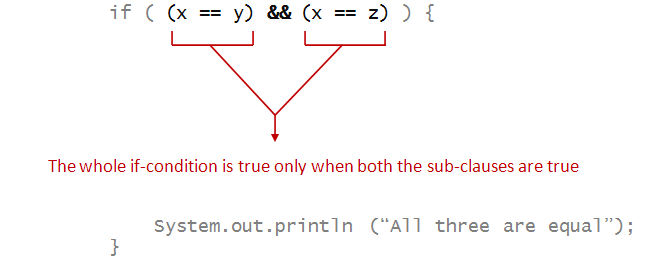
- The overall combination evaluates to true only when
BOTH the individual conditions are true.
In-Class Exercise 5:
Let's go back to this program:
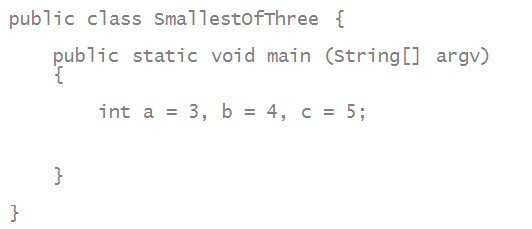
Use a two-subclause if-statement to identify
whether a is the smallest of the three.
In-Class Exercise 6:
Now extend this idea to identify which of the three
variables has the smallest value.
The OR operator:
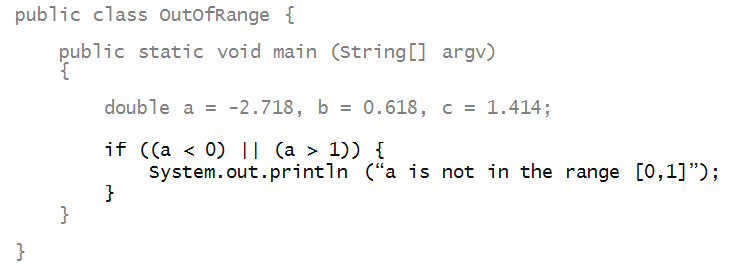
- Here, the || operator should be read as "OR".
- If either subclause is true, the whole is true:
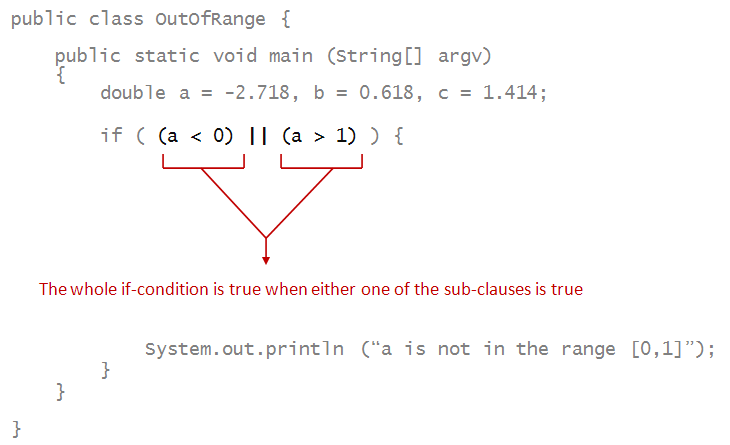
The NOT operator:
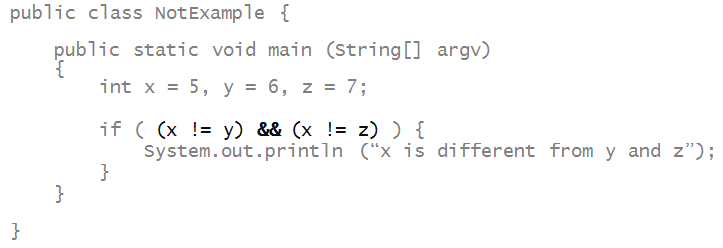
In-Class Exercise 7:
Modify the above to determine whether all three variables
have different values.
The NOT operator can be applied to a larger clause
made of sub-clauses:
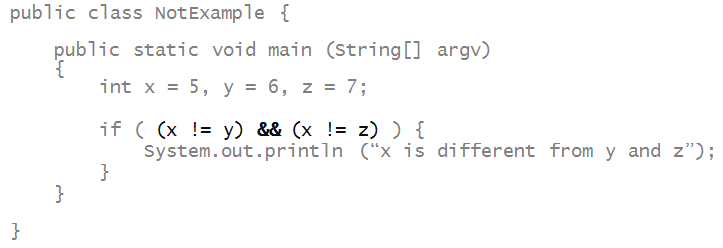
- Here, the inner clauses are first evaluated, and the
result is "flipped" to see if the NOT clause turns out to be true:

Boolean variables
What is a Boolean variable?
- An int variable takes integer values like:
12, 256, or -9.
- A double variable takes on values
like 3.141, -14.0, or 2.718.
- A boolean variables takes on one
of only two values: true or false.
An example:
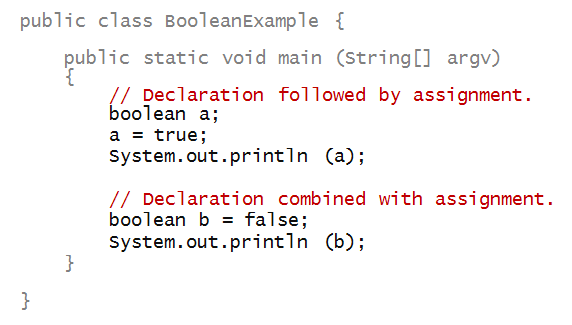
- boolean, true and false are reserved words.
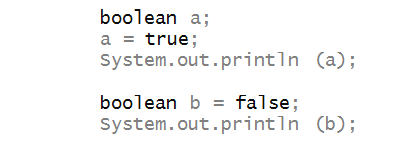
- Note: true and false are not in quotes.
Boolean operators:
Reading and writing
First, reading:
In-Class Exercise 8:
Draw a hierarchical picture that matches the conditional below.
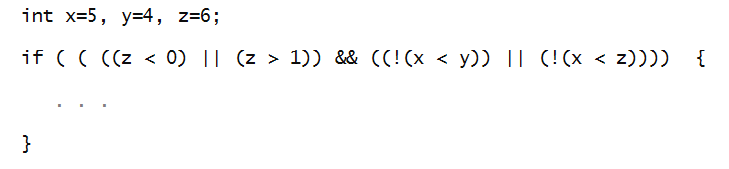
Then, evaluate it to see whether the result is true or false.
Write down the true/false values at intermediate levels of
the hierarchy.
Writing:
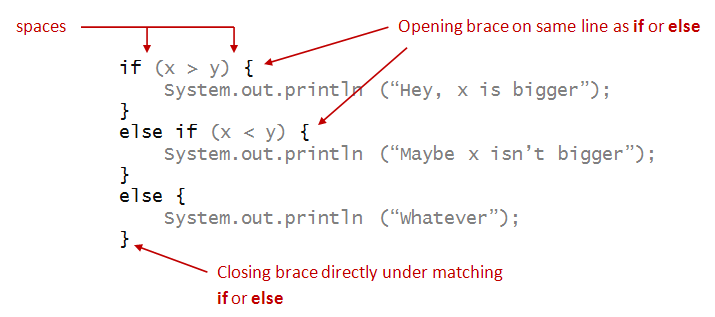
- Remember to type in the matching right bracket immediately
after typing in the left one.
- Use spacing and layout consistent with the above example.
When things go wrong
In-Class Exercise 9:
Identify the four errors in this piece of code:
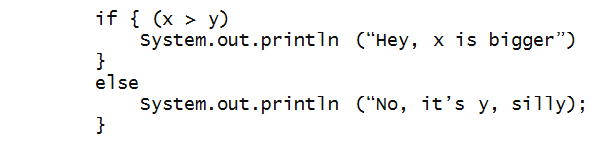
In-Class Exercise 10:
Fix the errors in the if condition to make
this print "Success".
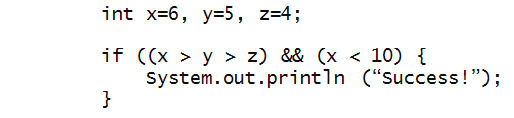
First, try to find the problems without compiling.
Then, fix the code and see if you were right while reading.
More Exercises...
In-Class Exercise 11:
Create a program to output health information based on BMI. Use at
least two variables: mass and height.
mass(lb) x 703
--------------------
height(in)2
BMI stands for Body Mass Index. This is a numerical value of your
weight in relation to your height. BMIs are good indicators of healthy
or unhealthy weights for adult men and women, regardless of body frame size.
- A BMI of 30 or higher indicated obesity.
- A BMI of greater than 25, but less than 30 is
considered overweight.
- A BMI of less than 25, but greater than 18.5 indicates a healthy weight.
- A BMI less than 18.5 is
considered underweight.
Do the following exercises:
- Write a solution that uses logical binary operators (e.g. && and ||)
and if, else, and/or else if.
- Write a solution that uses logical binary operators (e.g. && and
||) but no else/else if.
- Write a solution that uses nested conditionals (you can
use if and else), and no logical binary
operators.
- Draw a control flow diagram for each program.
Which version was the most difficult to understand? Which was the
simplest? Why?