Consider the following program:
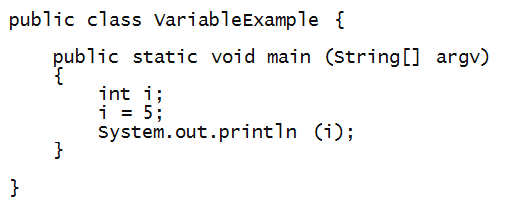
In-Class Exercise 2:
Edit, compile and execute the above program.
Now let's examine key parts of this program:
- First, i is the name of a "box" (of sorts).
- The term used for "box" is variable.
=> i is a variable.
- Variables must be "typed"
=> i can only store integers.
- To create and name a variable, we use a variable declaration:
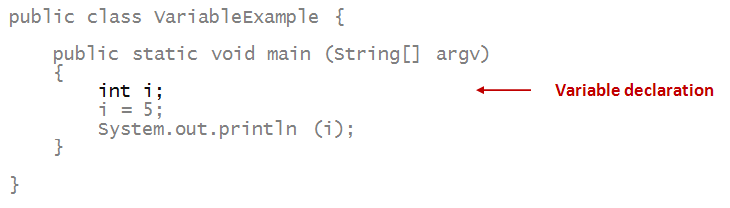
- Here, int is a reserved word in Java.
- To put something in a variable, we use assignment
=> with the repurposed = (equals) sign.
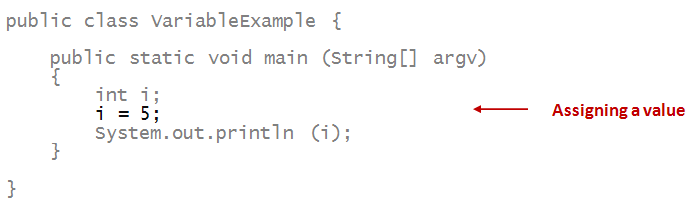
- When we print a variable, what gets printed is its value.
=> Thus, the number 5 gets printed
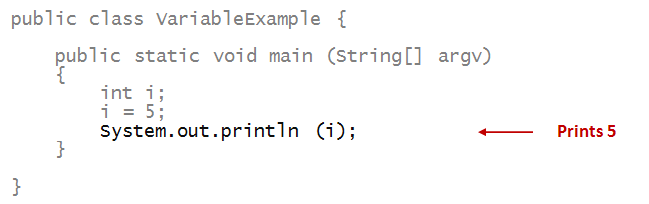
Next, let's look at assignment between variables:
- This is the analogue of cloning.
- Consider this addition to the above program:
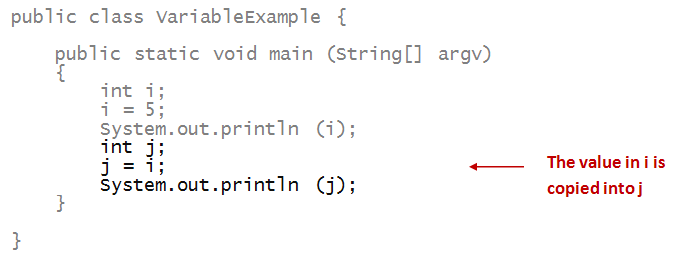
- We say, in short, "i is assigned to j".
In-Class Exercise 3:
What happens if you don't place a value in a variable?
Find out by editing and compiling this program:
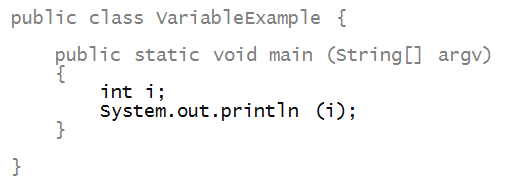
In-Class Exercise 4:
Identify the output of this program just by reading
(mental execution).
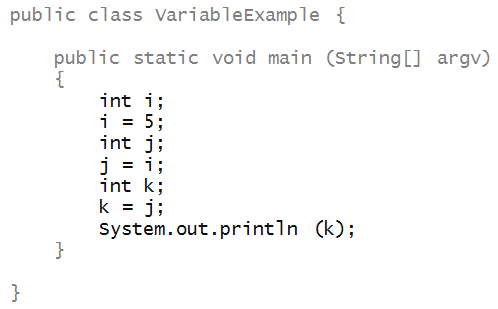
In-Class Exercise 5:
Identify the output of this program just by reading
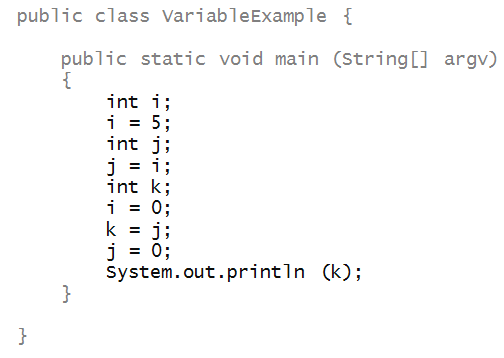
A rule: a variable must be declared before it is
used (assigned a value or have a value copied from it).
In-Class Exercise 6:
What is the compiler error you get for this program?
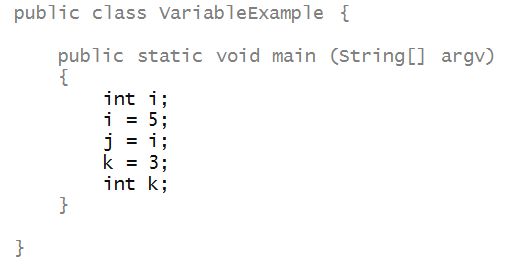
Integer operators
Let's examine the familiar binary operators
+, -, *, /
- Addition: +
- Subtraction: -
- Multiplication: *
- Division: /
- Consider this example with addition:
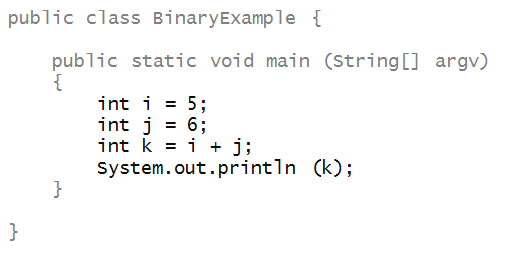
- What happens during execution:
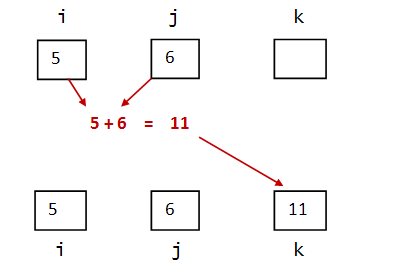
- The values in i and j
are added.
- The resulting value goes into
variable k.
- A long-ish way of saying this aloud:
=>
"k is assigned the sum of the values of
i and j"
- A shorter way:
=>
"k is assigned i plus j"
- Here's an example with multiplication and division:
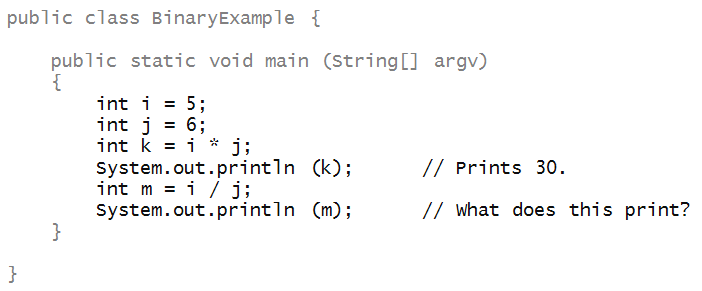
In-Class Exercise 7:
What does it print?
Next, let's consider some unary operators:
- These are operators that apply to a single variable.
- For example:
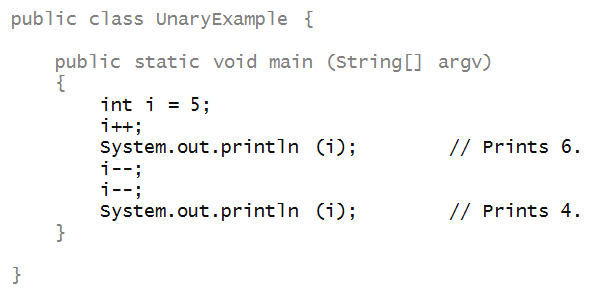
- The unary operators here are the ++ (increment)
and -- (decrement):
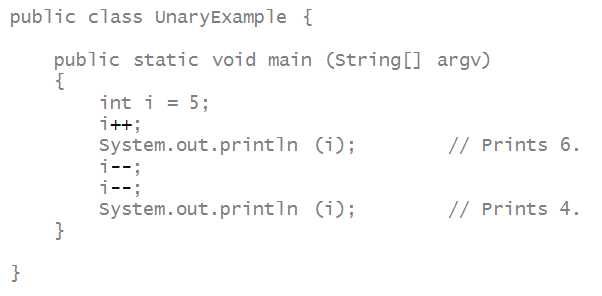
- Note writing style: no space between variable and unary operator, but this is also acceptable:
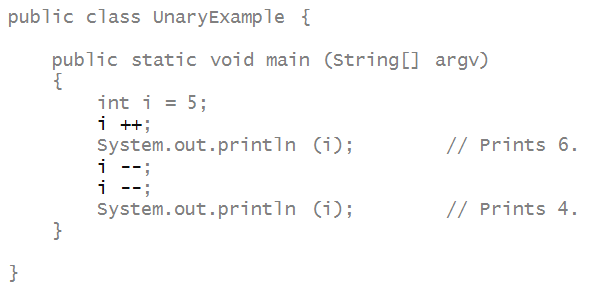
In-Class Exercise 8:
Mentally execute this program - what does it print?
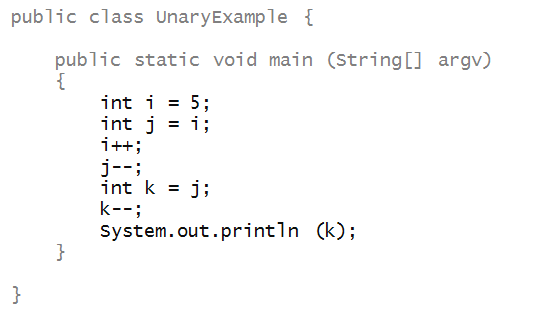
Variations
The following variations are worth knowing:
In-Class Exercise 9:
Consider the following partially-complete program:
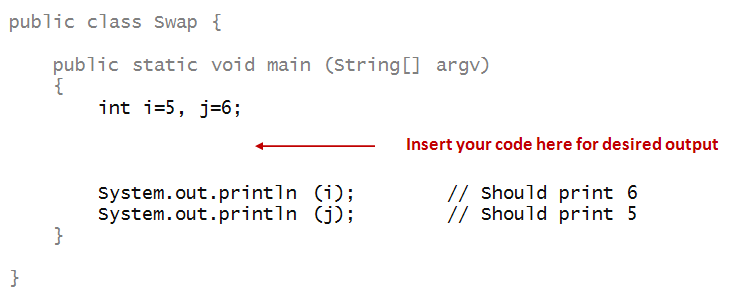
Insert code where indicated to have the program first
print 6, then 5. Do this only by using assignments between
variables.
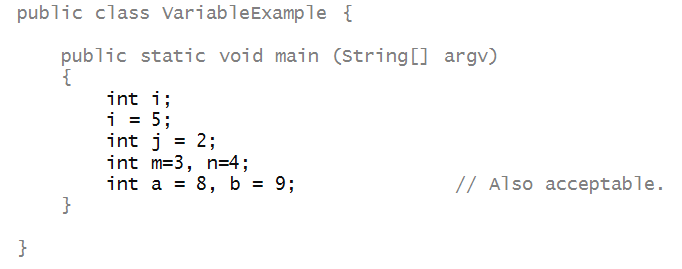
Note writing style:
- A space on either side of the equals sign for assignment.
- The same when you combine declaration and assignment
for a single variable.
- It's customary to remove the space for multiple declarations.
Expressions and operator-precedence
Consider the following program:
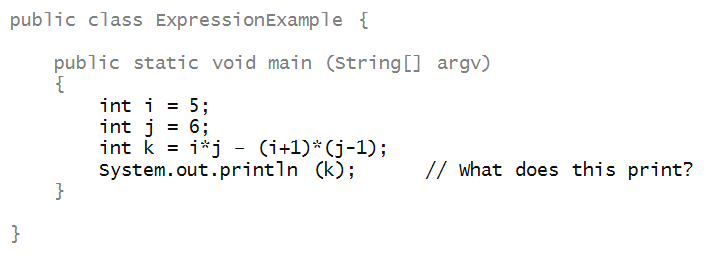
In-Class Exercise 10:
What does it print?
About expressions:
- An expression combines constants (like 1, above), and
variables using operators.
- Example:
i*j - (i+1)*(j-1).
- The above expression is really equivalent to:
(i*j) - ((i+1) * (j-1)).
Here, we added some clarifying parentheses.
- Operator precedence allows us to reduce the
number of clarifying parentheses.
- Java precedence follows standard predence in math:
/, *, +, -.
- The above expression is NOT the same as:
i*j - i+1*j-1.
In-Class Exercise 11:
Add parentheses to clarify the
expression i*j - i+1*j-1.
What does it
evaluate to when i=7 and j=3?
More about expressions and assignment
Here are two more operators, one unary (negative sign)
and one binary (remainder or mod operator), that are commonly used:
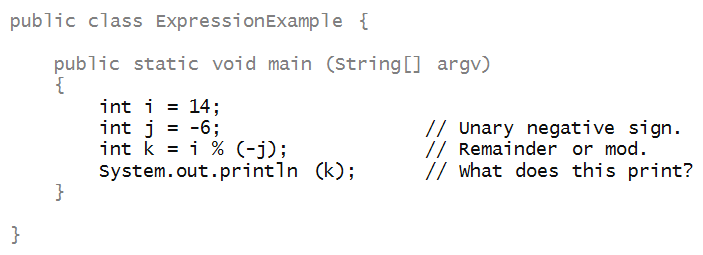
In-Class Exercise 12:
What does it print?
Now we'll look at a strange (initially) but very useful type of
assignment:
- Consider this program:
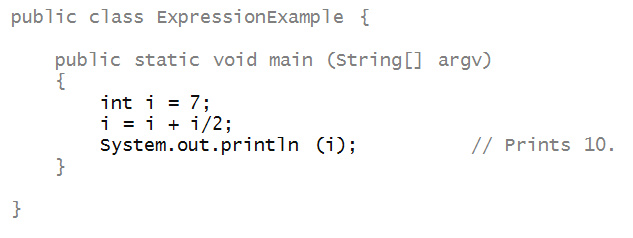
- Prior to evaluating the expression, i has value 7.
- On the right side, the current value of i is used
to evaluate the expression.
=> Thus, the expression evaluates to (7 + 7/2) = 10.
- This evaluated value then goes into variable i.
=> After the assignment, i has the value 10.
When things go wrong
As you might imagine, there are many ways to inadvertently
create errors.
Let's start with compilers errors.
In-Class Exercise 13:
Try to identify the compiler error (or errors),
by reading each program below.
Program 1:
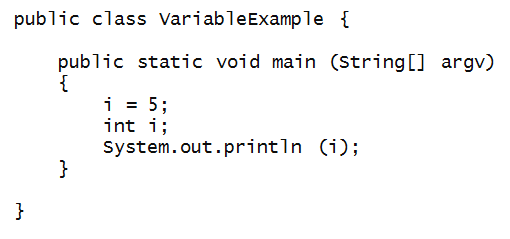
Program 2:
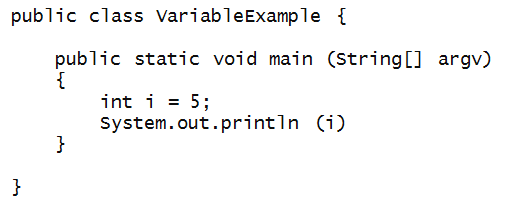
Program 3:
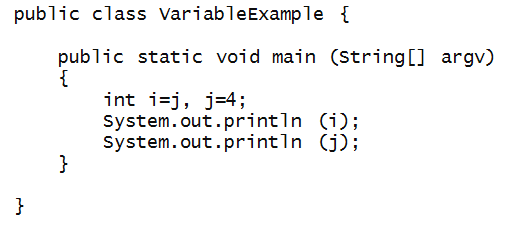
Program 4:
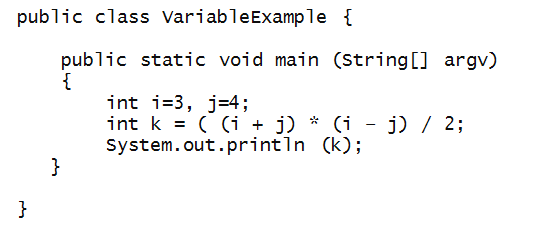