One can insert comments into code that do not
affect the code itself:
- For example:
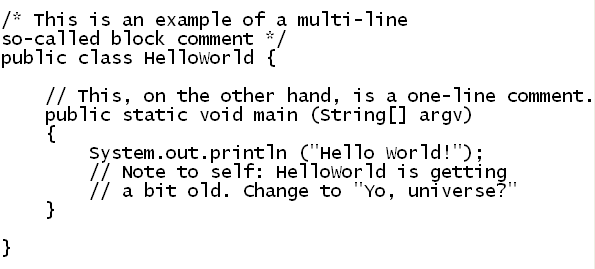
- Comments are similar to their analogue in editing
text documents, e.g.
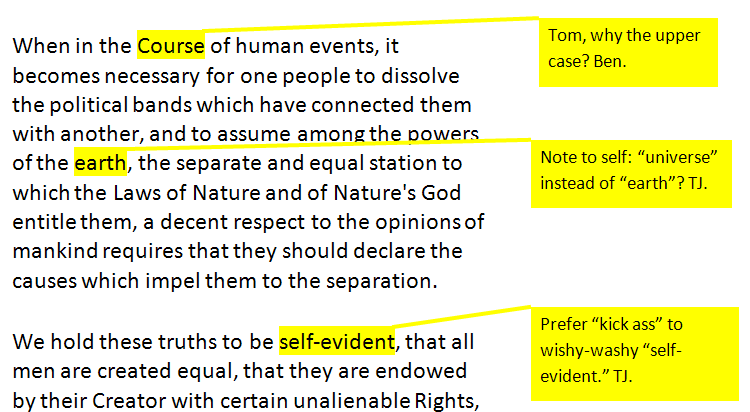
- There are three kinds of comments:
- Single line comments that begin with \\.
- Multi-line comments beginning with /*
and ending with */.
- Javadoc comments (for advanced use in automated documentation).
- We will use only the single-line comment in this course.
- A single line comment ends at the end of the line.
- This is a mistake:
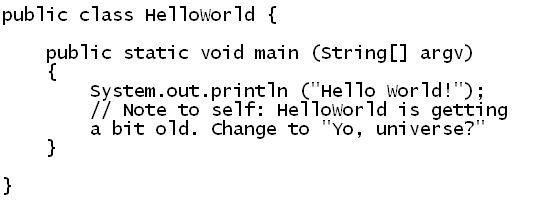
In-Class Exercise 1:
What is the compilation error for the above program?
Fix the error, add your own multi-line and single-line
comments and compile again.
Strings
About strings:
- A string in general computer science terminology
is a sequence of letters or symbols.
- A string in Java is any sequence of letters or symbols
enclosed by a matching pair of double-quotes.
- Examples of strings:
"Hello World!"
"'sup?"
"@#%&* [Expletive deleted]"
- Thus far, we have only used strings for printing:
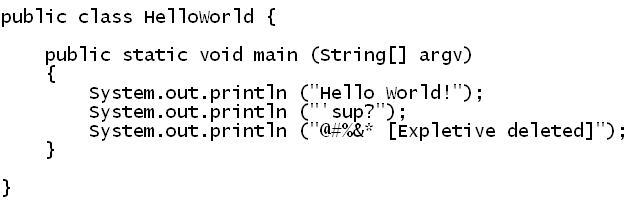
- Later, of course, we will see many other uses.
- A string cannot be typed over multiple lines.
- This is a mistake:

In-Class Exercise 2:
What is the compilation error for the above program?
Fix the error.
Escape sequences
The fact that strings begin and end with double quotes
begs the question: how does one print out a double-quote?
- To solve this problem, Java uses a trick:
=> A special code is used for a double-quote.
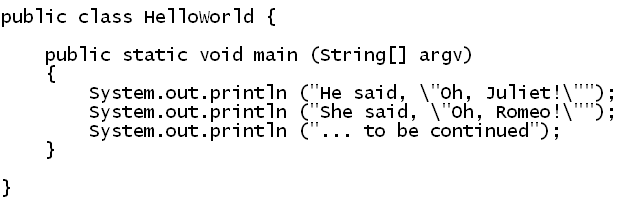
- But this begs the question: how do you print
a backslash?
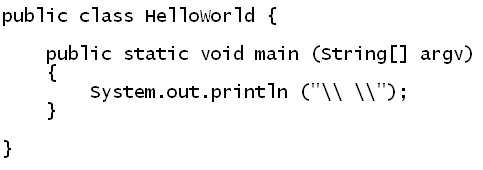
Answer: a double-backslash prints as a single one.
The above program prints out two backslashes, separated by a space.
- Java has a number of (esoteric) escape sequences.
=> There's no need to know them all.
- The important ones:
- \" for a double-quote.
- \\; for a backslash.
- \n; for a new line.
In-Class Exercise 3:
Write a program called MyInitials.java that will
print out both your initials side by side in large size, as in
(e.g., GP):
\\\\\ """"
\ " "
\ \\\ """"
\ \ "
\\\\\ "
print and println
You've been wondering why something that prints is
called println instead of print.
In-Class Exercise 4:
What does this program print to the screen?
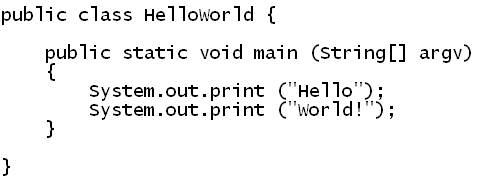
Compare the output to the standard HelloWorld program
very carefully.
In-Class Exercise 5:
Without using println, get the above program to
print exactly like the standard HelloWorld.
About print:
- Thus, println is essentially a print
followed by the escape sequence for a newline.
- Later, when we learn for-loops, we will
see a use for print.
Identifiers
There are three kinds of "words" in Java:
- The first kind are the core language words, called
reserved words such as class and void:
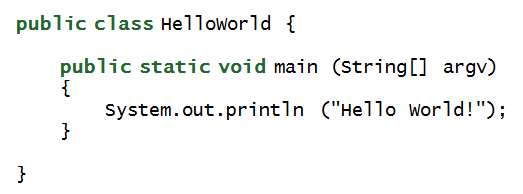
- Reserved words are used to define program structure and control.
- Reserved words may only be used in the ways they are meant for.
In-Class Exercise 6:
Look up the list of reserved words in Java - either in your
book or on the internet. How many are there?
- Words that we make up for our programs, such as:
HelloWorld:
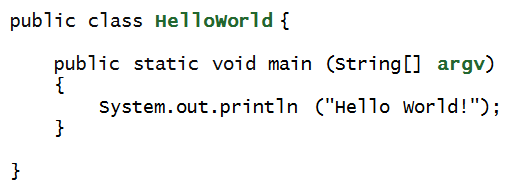
- These are words we can change.
=> Recall: we've changed the name of the class before.
- It may come as a surprise that argv is something
we could re-name.
=> We will understand this when we learn about method parameters.
- The third kind are words chosen by other programmers,
especially those who designed code in the Java library,
such as:
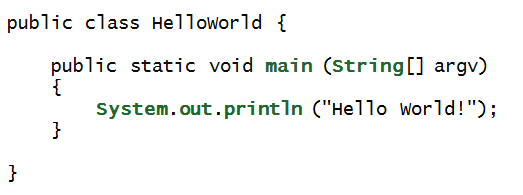
- We have no choice in using these words.
=> We can't change them.
About identifiers:
- The latter two kinds of words are called identifiers.
- There are some restrictions on creating identifiers:
- They must start with a letter.
- They must use only letters, numbers, or the
underscore _ symbol.
- There are also some stylistic guidelines in choosing
identifiers. We'll talk about these later.
Let us next explore errors made with these words.
In-Class Exercise 7:
For each of the programs below:
(1) Try to identify the problem (if any) just by reading.
(2) Then, use the edit-compile-test process
to see what the error is (if any).
Program 1:
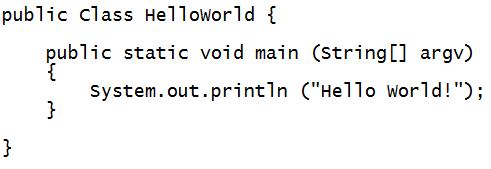
Program 2:
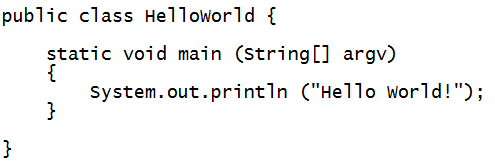
Program 3:
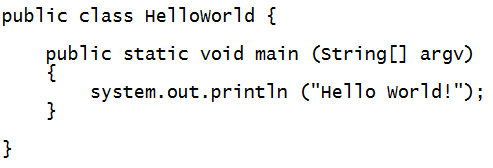
Program 4:
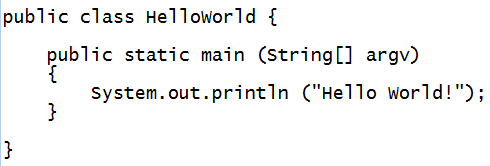
Program 5:
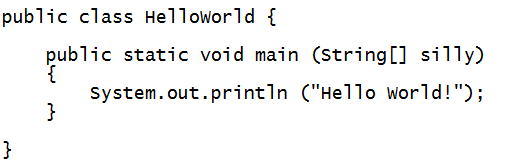
Good writing habits
First, let's point out how NOT to do it:
- Write programs without full concentration.
- Write carelessly, thinking that repeated compilation
will find your syntax errors anyway.
- Write by looking at existing code, letter by letter.
- Write in sequence from the first letter to the last.
Good writing habits:
For example, with HelloWorld:
- First type in the class:
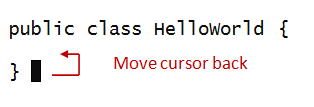
- Then the method outline:
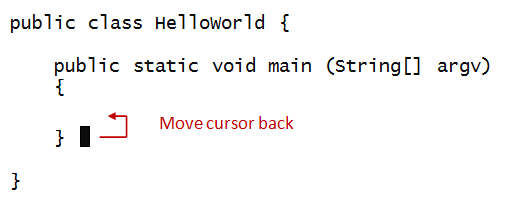
- And then the method code.
- Generally, if the brackets are going to be separated
by multiple lines, type both and then move the cursor backwards.