Consider this simple program:
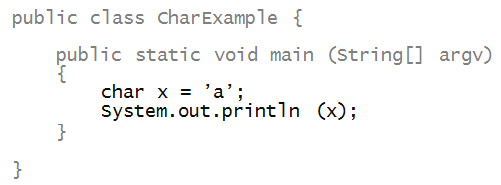
- Here we used the reserved word char:

- Notice how actual characters are enclosed in apostrophes:

- The variable declaration and assignment are very
similar to int's or double's,
except for the apostrophes:
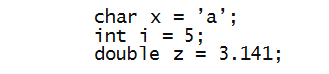
More examples:
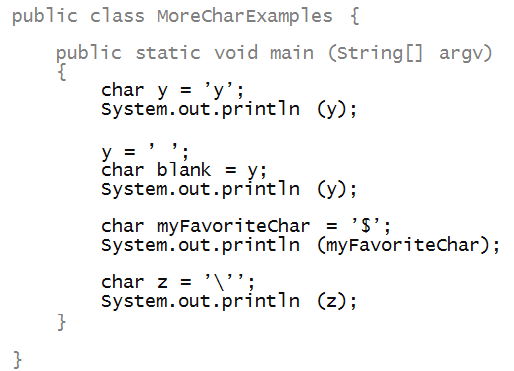
- Note the syntactic distinction between the variable
y

and the character 'y':

- Notice how space or blank is used
as a character:

- One can copy across variables just as we did with
other basic types:

- The same rules about naming variables apply:
a variable name can have letters or numbers but must
start with a letter:

- To use the apostrophe as a character value itself,
we need the "escaped" version:

Operators for char variables:
- With int's and double's we had
arithmetic and comparison operators.
- For char's only the comparison operators make sense:
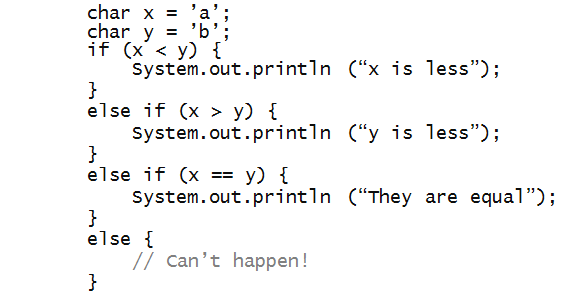
The (useful) relationship between int and char
Consider this program:
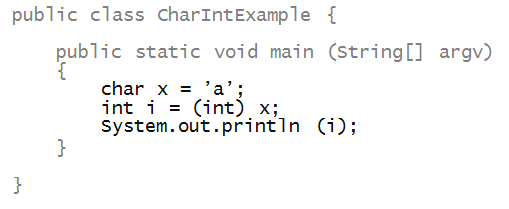
In-Class Exercise 1:
What does the above program print?
In-Class Exercise 2:
Now let's go the other way. Assign the value
100 to an int variable and then
from there copy it (with casting) to a char variable. Print the
char variable. What is the output?
About the conversion:
- Under the hood, char's are represented
by integer codes.
- It is this code that we see as the number, after casting.
Strings
Consider this example:
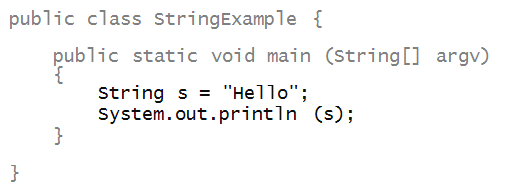
- A string is a sequence of characters.
- Notice how a string is declared:

- Important: String is NOT a reserved word.
=> - String is a special kind of object in the
Java language.
- The (zero or more) characters in a string are enclosed
in double-quotes:

Java defines a special operator for concatenating
strings:
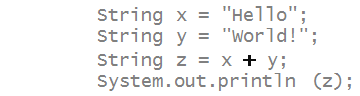
In-Class Exercise 3:
Modify the code below so that only a single System.out.println
is used.
public class ArrayPrint {
public static void main (String[] argv)
{
int i = 5;
int j = i * i;
System.out.print ("The square of ");
System.out.print (i);
System.out.print (" is ");
System.out.println (j);
}
}
String methods:
- Because strings are objects, it is possible
to define methods inside them.
- The Java String object has many such useful methods:
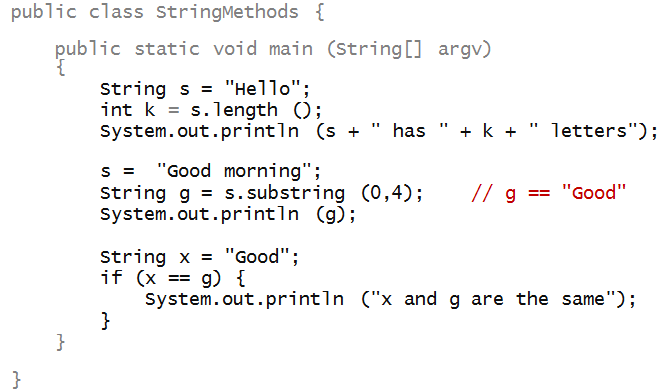
- Note that length is a method and that
it is called using the dot operator with the variable:

- The method takes no parameters but returns an integer:

- One can extract a sub-string from a given string using
the substring method:
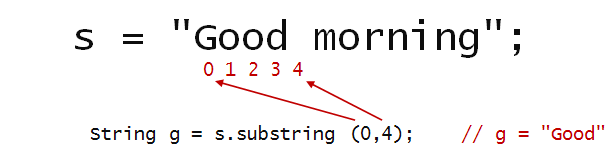
- Think of the characters in a string being
indexed, as in an array, starting with 0.
- The first parameter to substring is
the index of the start of the substring.
- The second parameter is one more than the index
of the last char of the substring.
In-Class Exercise 4:
Type up the above program
(StringMethods)
and execute. What goes wrong?
To see if two strings are the same,
use the equals method in
one of the strings to be compared:

To compare two strings alphabetically, use
the compareTo method:
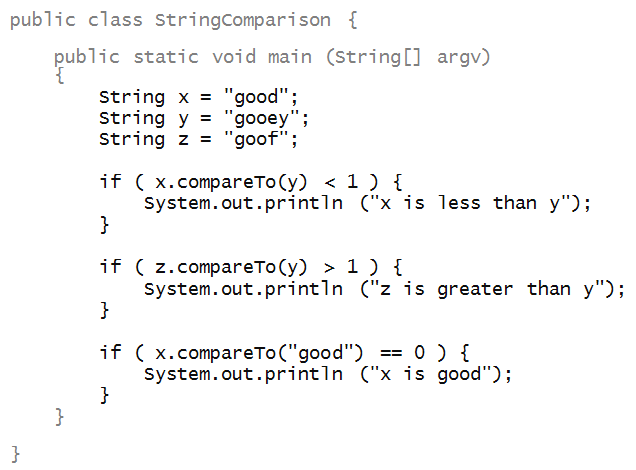
The compareTo method
returns one of three integers:
- -1, if the parameter string is greater.
- 1, if the parameter string is less.
- 0, otherwise.
There are other useful methods for strings. Here
are three more:
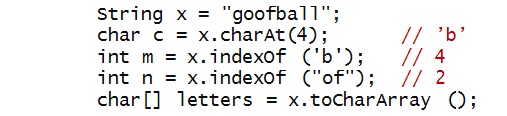
- To obtain the character at the i-th position of
the string, use charAt(i).
- Use indexOf with either a char
or String parameter to see whether the
parameter exists in the string. The first position
found is returned (or -1, if not found).
- One can extract a char
array from a string using
the toCharArray method. Ignore
this method for now.
More on Java "utility classes":
For a full list of String methods, see
the javadocs.
One of the strongest features of Java is the extensive
libraries. You can find these by browsing the javadocs, or often by
typing "javadocs X" into google where "X" is the class you are
curious about (e.g. String, or Math).
Reading the Javadocs and method overloading
An API is an Application Programming Interface, and is a set of
methods you can use. Javadocs provides documentation on the APIs
that Java supports. Lets understand how to read the APIs a little
better.
When reading one of the methods within the Javadocs, do the
following:
- Read the method name. What do you think it does?
- Look at the types and number of parameters. What do you think it does?
- Look at the return type. What do you think it does?
- Read the English description. What do you think it does?
Take the time to read each component separately, and try and figure
out what the method does.
Example:
int indexOf(int ch)
Returns the index within this string of the first occurrence of the
specified character.
Other strange things you see in the javadocs:
int indexOf(int ch, int fromIndex)
Returns the index within this string of the first occurrence of the
specified character, starting the search at the specified index.
int indexOf(String str)
Returns the index within this string of the first occurrence of the
specified substring.
int indexOf(String str, int fromIndex)
Returns the index within this string of the first occurrence of the
specified substring, starting at the specified index.
What does this mean?
Method overloading
Multiple methods with the same name (identifier).
Each instance has parameters of different
types.
The invoked method is the one with the types that match the
invocation.
public class OverloadingExample {
public static void main(String[] args) {
myIndexOf("hey");
myIndexOf('a', 10);
}
public static int myIndexOf(int ch) {
System.out.println("int parameter");
}
public static int myIndexOf(int ch, int fromIndex) {
System.out.println("int parameter, with offset");
}
public static int myIndexOf(String str) {
System.out.println("String parameter");
}
public static int myIndexOf(String str, int fromIndex) {
System.out.println("String parameter, with offset");
}
}
In-Class Exercise 5:
What does the previous code output?
Parsing and creative use of APIs
Parsing is the act of making sense of a string of text. Java parses
the code you write, and interprets it as code. Java is just reading
in a large string, and trying to understand its syntax!
Where have you seen strings that your program will want to
parse?
In-Class Exercise 6:
For the following sets of input, and output strings, which string
methods could I use to transform the strings? Use
the String javadoc
to find methods to help you. Think of these as a puzzle.
Input strings: "Hello World!", "Hello Class!", ...
Desired output: "Goodbye World!", "Goodbye Class!", ...
Input strings: "HEllO World!", "heLLo Class!", ...
Desired output: "goodbye world!", "goodbye class!", ...
Input strings: " asdf", "
jkl; ", ...
Desired output: "asdf", "jkl;", ...
Input strings: "Lucky number (10)!", "I like (40)!", ...
Desired output: "10", "40", ...
Please implement and test each transformation as a separate method.
In-Class Exercise 7: Write a simple
program that will determine the winner in a simple card game of
"war". Write a method that takes as input a string of the format
"4s", which denotes the four of spaces. The suit is denoted by "s"
= spades, "d" = diamonds, "h" = hearts, and "c" = clubs. In this
exercise, you will write a war method to
take two strings as input, and returns 0 if the first string
contains a "higher value" card, 1 if the second string does, and -1
if the inputs are not correctly formatted.
The card with the higher numerical value, wins. These values are
between 0 and 9. No 10s, aces, kings, queens, or jacks. If two
cards have the same value, then the suit breaks the tie. In that
case, clubs > diamonds > hearts > spades.
- Write a method to check if a card is correctly
formatted. How could the data be
ill-formatted? formattedCorrectly
should check if a String is formatted
correctly.
- Write the war method.
- Challenge: Make your program as small and simple as possible
with the fewest possible conditionals.