Consider the following program:
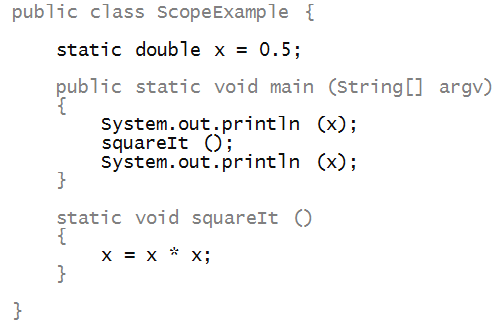
In-Class Exercise 1:
Execute the above program. What does it print?
Let us point out a few things:
- The variable x is declared outside
any of the methods, including main:
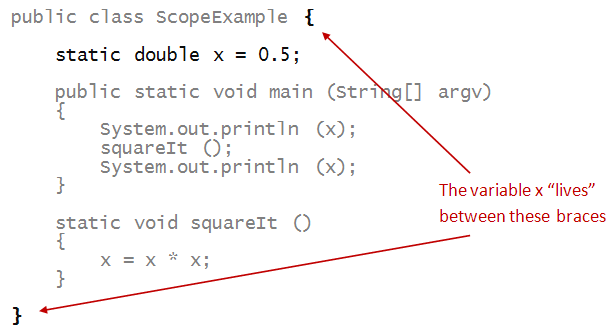
- It is accessible in any method defined in the class:
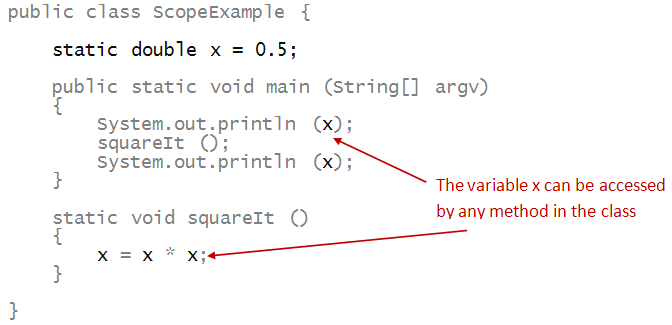
- Such a variable is called a global variable.
=> It's global to all the methods in the class.
In-Class Exercise 2:
Define another global variable, an int, and
two new methods. Then use (and modify) the new variable in both
methods. Demonstrate its use by printing the values
of the variable both before and after modification.
Why are globals useful? Let's look at an example:
- Suppose we have the following data in the file file.data.
5
2.0 1.0
0.5 3.0
3.1 5.2
4.0 3.6
5.0 0.5
The integer on the first line is the number of points. Then, each
line has one (x,y) pair.
- Goal: read the points and then compute the minimum, maximum
of the x and y values.
- Here's the program:
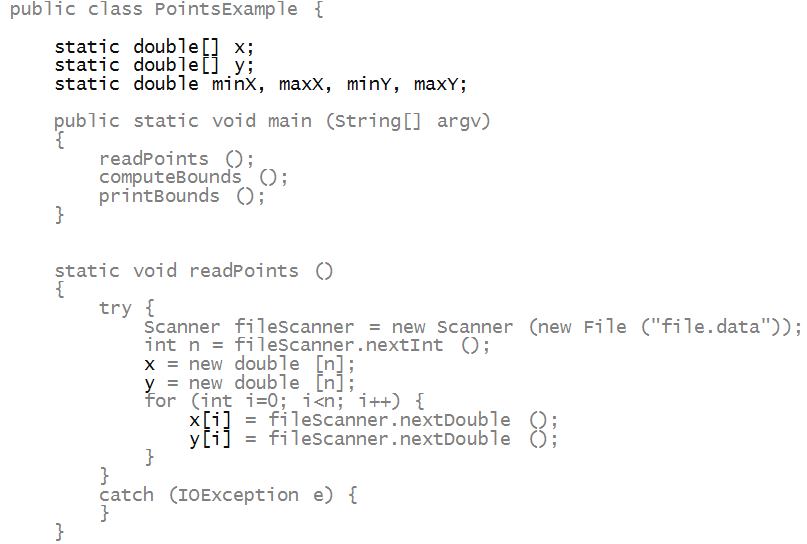
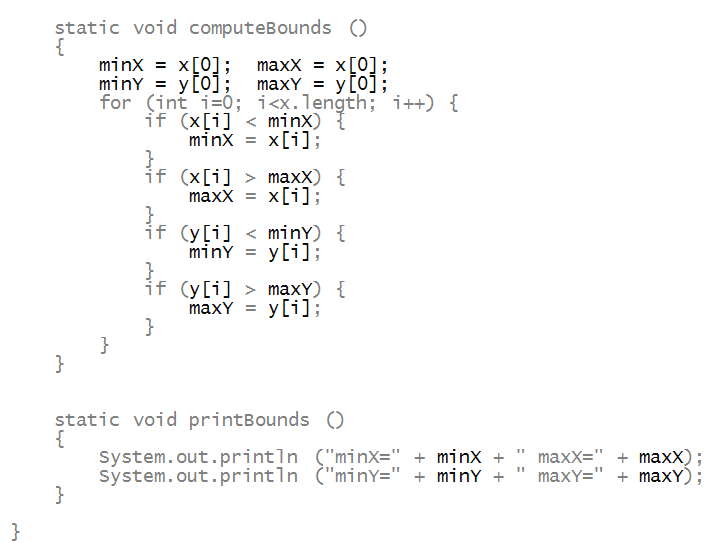
- Here, we've conveniently used a single set of
globals that is accessed by multiple methods.
- If we did NOT use globals, we would have to use
return values and parameters.
In-Class Exercise 3:
Achieve the same results without global variables.
Instead of computeBounds, you can define
one method each for the min and max of x values,
and one method each for the min and max of y values.
Variable shadowing and scope
Consider this program:
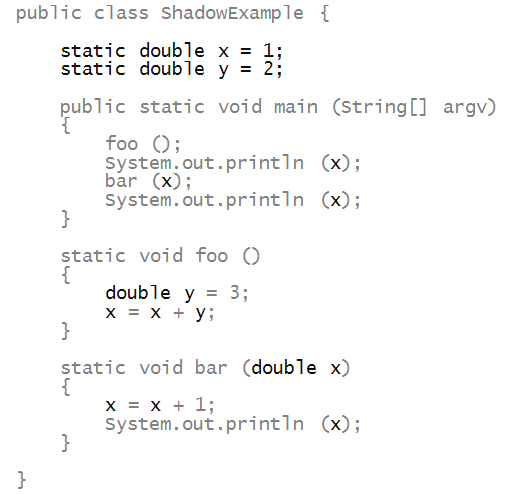
- Consider the method foo:
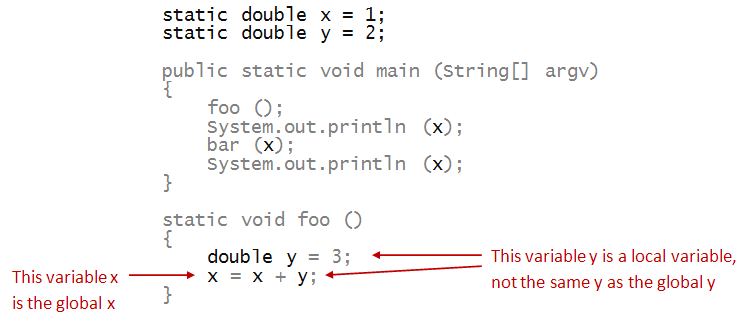
The local definition of a variable with the same name y
shadows the name of the global.
- Something similar happens with the method bar:

Thus, parameter variables shadow (obscure) global variables
of the same name.
In general, variables can be defined in any block
of code:
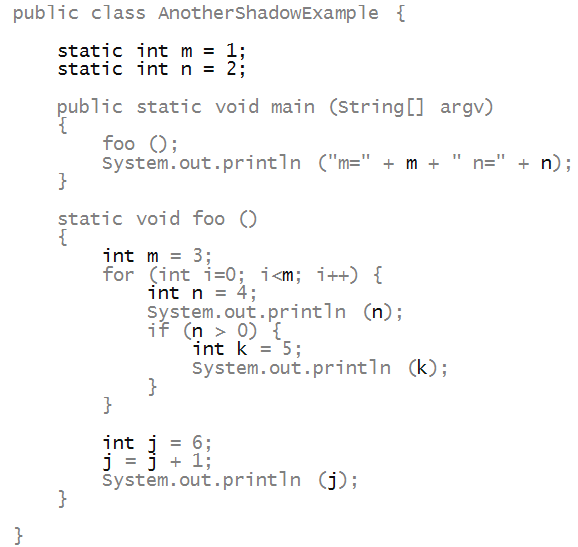
- Here, the m and n in main
refer to the global m and n
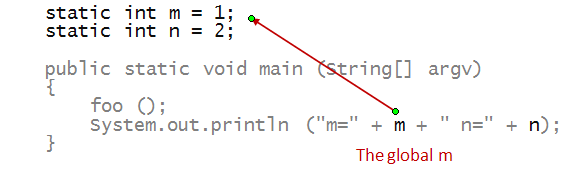
- However, the m in foo is a local variable
that shadows the global one:

- The scope of a variable is the block of code
that can access a variable.
- The scope of the global variable m is
any method inside the class.

- Similarly, one can see the scope of the other variables
in method foo, for example m:
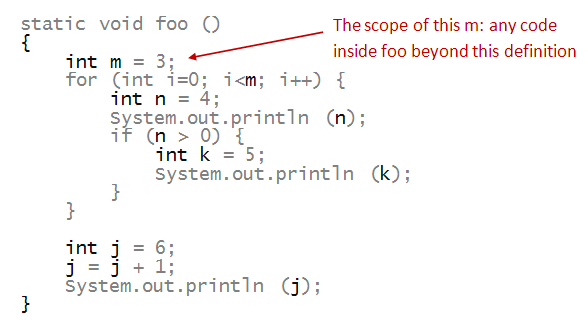
- And the others:
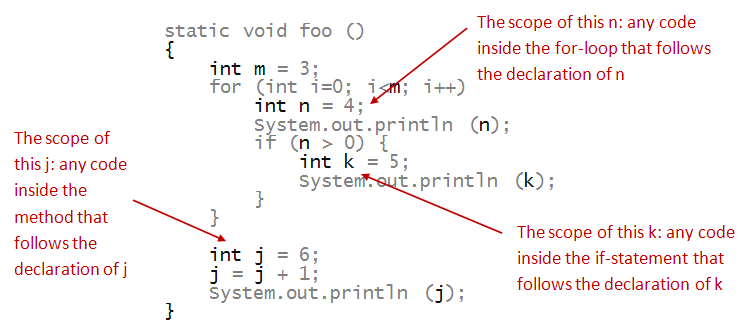
Now consider this example:
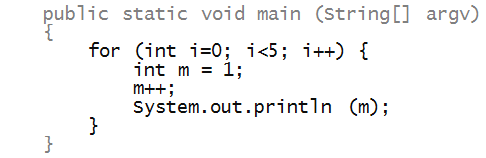
In-Class Exercise 4:
What gets printed out? The value 1 repeatedly
or increasing values of m?
In-Class Exercise 5: Write a class, a
method, and a number of conditionals and loops.
- Use variables within each of the scopes and experiment with
which ones are shadowed.
- Use another method, with arguments that shadow global
variables, and with local variables that also shadow other
variables.
- When does shadowing work as expected, and when does it yield a
compiler error?
Packaging and encapsulation
Consider the following program:
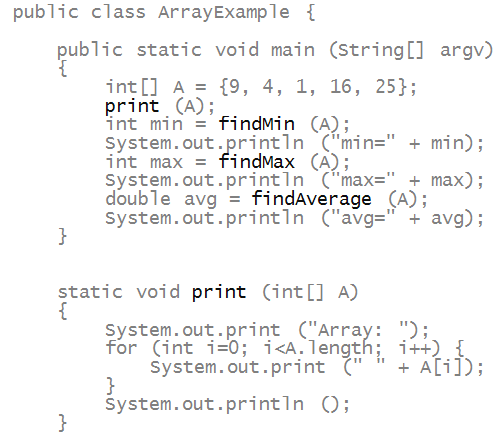
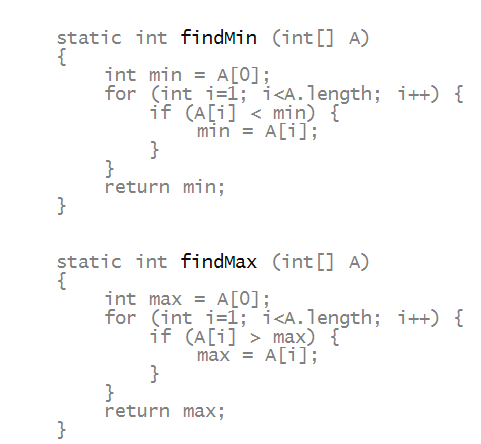
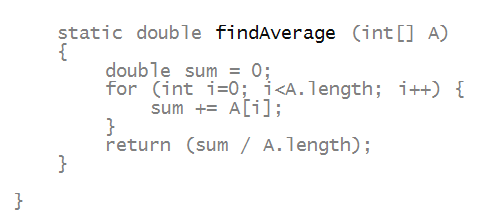
- Here, we see that there is an array and a bunch
of methods that "do things" with the array.
- Now, the methods appear to be of general use to
a variety of arrays, and could be useful in the future.
- Suppose we were to "package" these useful methods
into a class called ArrayTool as follows:
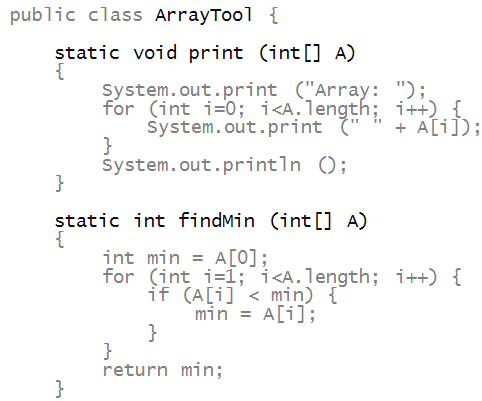
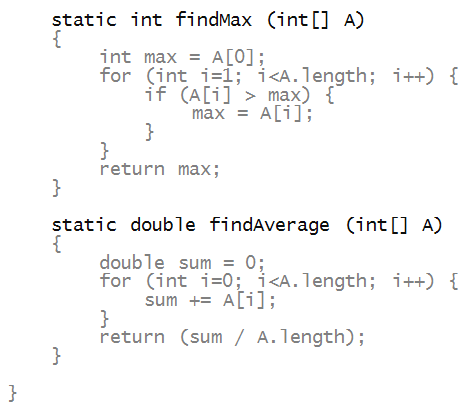
- Then, these could be used in the future by
any program in the same directory as ArrayTool, e.g.,
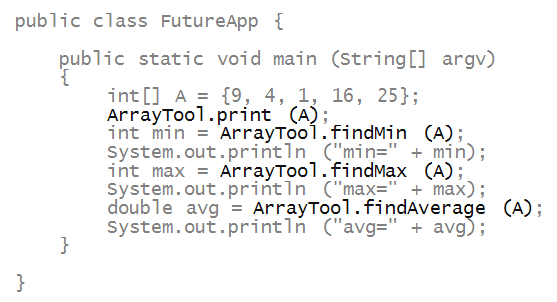
- This type of packaging is called encapsulation.
- Related code can be together.
- Other programs need not know the details, they can
just use (i.e., call) the methods in the class.
- Such encapsulation and re-use is a key part of
how efficiencies are realized in software development.
In-Class Exercise 6:
Add a new useful method to ArrayTool above
and use it in a new application.
Visibility and Encapsulation
Consider the following program that is split into two files. Make
sure both files are in the same folder/directory. First,
VisibilityTest.java:
public class VisibilityTest {
public static int a = 3;
static int b = 4;
private static int c = 5;
static void helper() {
return 1;
}
public static void helper2() {
return helper(n) + 1;
}
}
Second, VisibilityMain.java:
public class VisibilityMain {
public static void main(String[] args) {
System.out.println(VisibilityTest.helper());
System.out.println(VisibilityTest.helper2());
System.out.println(VisibilityTest.a);
System.out.println(VisibilityTest.b);
System.out.println(VisibilityTest.c);
}
}
It is quite common to break the main method into a separate class.
This is how we grade your homeworks!
In-Class Exercise 7: Fix the compiler
errors from the given example. Why are there errors? What are the
keywords in the program that are causing the compiler errors?
Programmers can control the visibility of each of the methods
and global variables within their classes.
- Visibility controls if a method outside of the class can
access the given method/variable.
Visibility is controlled by
the public
and private keywords
public means that the
method/variable is accessible outside of the class.
private means the opposite. The
symbol is private to the class.