Module 2: Simple Drawing
An example with drawing geometric shapes
Consider the following program:
import java.awt.*;
import java.applet.*;
public class CircleSquare extends Applet {
public void paint (Graphics g)
{
// Draw a red, filled circle:
g.setColor (Color.red);
g.fillOval (50,50,70,70);
// Draw an unfilled blue square next to it:
g.setColor (Color.blue);
g.drawRect (140,50,80,70);
}
}
Note:
- The program draws two geometric objects: one is a circle that's
filled in and the other is a square.
- In the method fillOval, the
(50,50) specifies the topleft corner of the square (in
general, rectangle) containing the circle (in general, oval).
The first 70 is the width of the square and the second 70 is
the height of the square.
- Similarly, the top left corner coordinates (140, 50),
width (80) and height (70) are specified in the call
to drawRect.
- This is what the applet looks like:
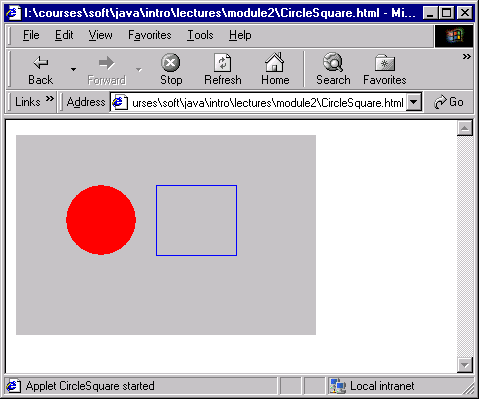
- Link to the applet
- The setColor method is used to set the paint color.
- Other available colors: black, blue, cyan, darkGray, gray,
green, lightGray, magenta, orange, pink, red, white, yellow.
- Colors can also be created by mixing, e.g.,
import java.awt.*;
import java.applet.*;
public class CircleSquare2 extends Applet {
public void paint (Graphics g)
{
// Mix red and blue: range is 0-255
g.setColor (new Color (255, 0, 255));
g.fillOval (50,50, 70,70);
// Mix some red, with mostly green and blue
g.setColor (new Color (100, 255, 255));
g.fillRect (140,50, 80,70);
}
}
Link to applet
In-Class Exercise:
Draw a square and then a circle around the square so that
the four corners of the square are on the circle.
A more interesting drawing example
Consider the following program:
import java.awt.*;
import java.applet.*;
public class Face extends Applet {
public void paint (Graphics g)
{
g.drawOval (40, 40, 120, 150); // Head.
g.drawOval (57, 75, 30, 20); // Left eye.
g.drawOval (110, 75, 30, 20); // Left eye.
g.fillOval (68, 81, 10, 10); // Left pupil.
g.fillOval (121, 81, 10, 10); // Right pupil.
g.drawOval (85, 100, 30, 30); // Nose.
g.fillArc (60, 125, 80, 40, 180, 180); // Mouth.
g.drawOval (25, 92, 15, 30); // Left ear.
g.drawOval (160, 92, 15, 30); // Right ear.
}
}
Note:
- This program draws a face the simple geometric commands.
- The only new one here is fillArc, to which is
given four rectangle parameters, a starting arc angle and the
overall angle of the arc.
- This applet looks like:
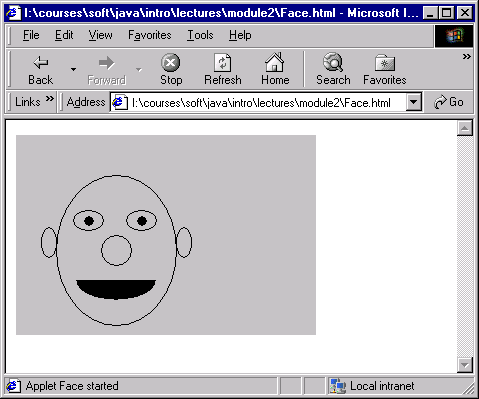
- Link to applet
In-Class Exercise:
Fill in some color to make the face more interesting. Add eyebrows.
In-Class Exercise:
Can you figure out how to set a background color (say, yellow) for
the whole applet?
Browsing the API
Are there more methods for drawing? How does one find out?
- Java provides documentation for all the classes in the Java
library, of which Graphics is the relevant one above.
- Initially, the API documentation is intimidating because
it's huge, like an encyclopaedia. But like an encyclopaedia
it's also navigable so you can get what you want.
- Start by looking at the main
documentation page at SUN. There are a lot of links. Look for
the English-version link to the JDK or SDK (or J2SE) documentation.
- For example, clicking on the 1.4.2 link takes you
here.
- In this page, look for the API documentation, which for 1.4.2
should take you here.
- The API documentation will look like this:
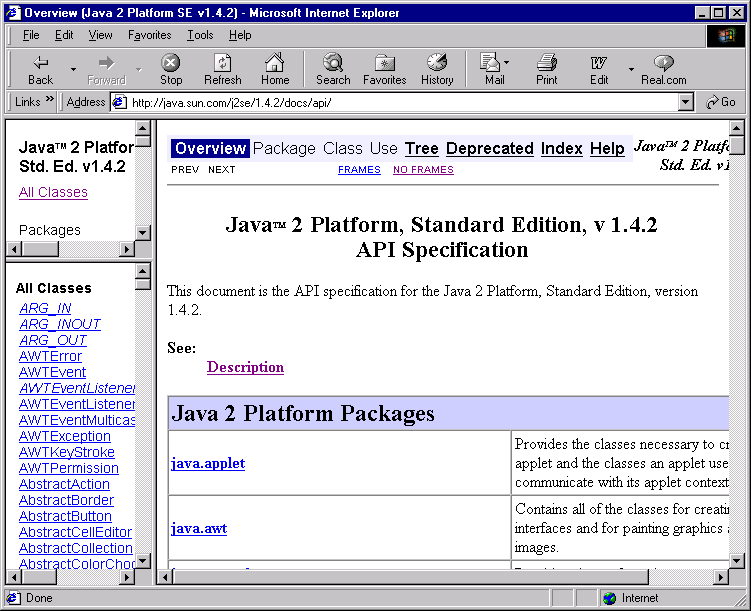
- Now scroll down the lower menu ("All classes") on the left to
look for Graphics and click on "Graphics". This is what you
should get:
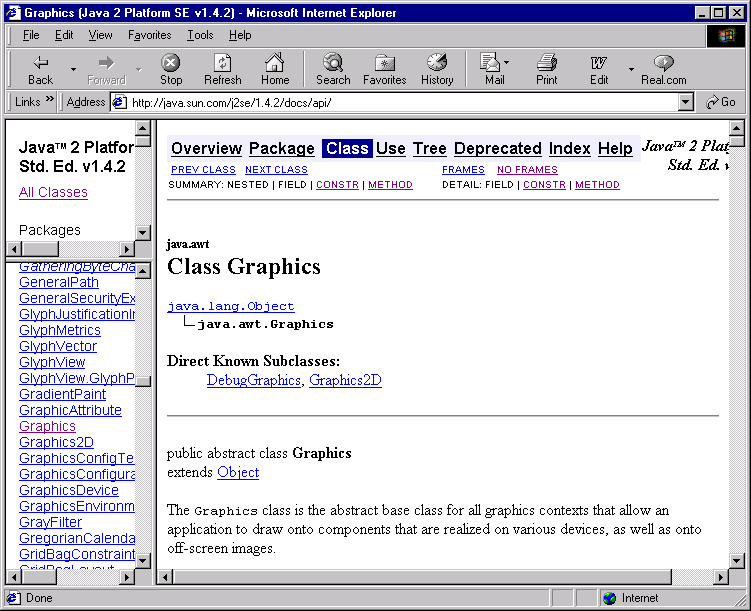
- Click once on the right sight and scroll down Graphics to where
the methods are described. For example, if we were to look up
the method drawOval:
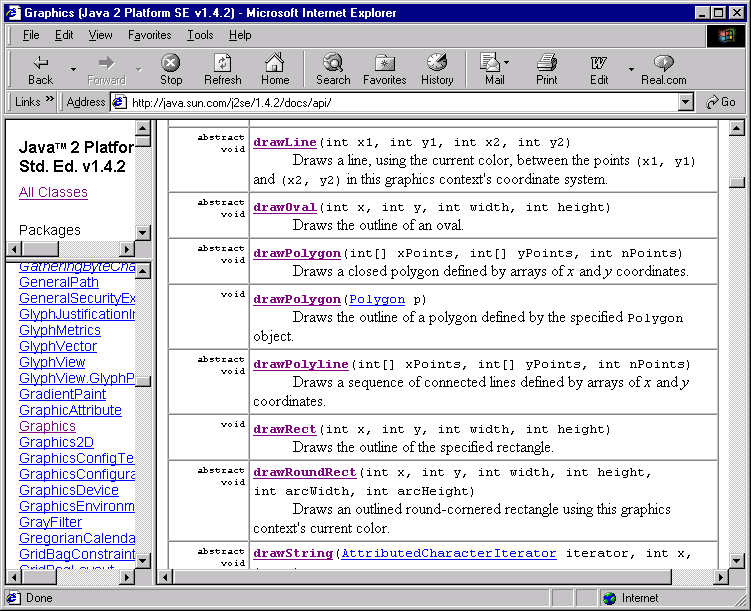
- Now you can click on the method name and get more information
on how to use it.
- How do you find out about functionality when you don't know
the name of the right class? That's harder. A book or tutorial is
the best place to start, such as
Java's tutorials homepage.
In-Class Exercise:
Modify the Face example to add an interesting polygonal object
to the picture.
Making the HTML more interesting
Applets can be made more interesting by using the
surrounding HTML to write stuff, include pictures and anything
you can do with HTML.
For example, let's make a tiny modification to both the
Face program and the Face HTML file:
- Modification to the Face program:
import java.awt.*;
import java.applet.*;
public class Face2 extends Applet {
public void paint (Graphics g)
{
g.drawOval (40, 40, 120, 150); // Head.
g.drawOval (57, 75, 30, 20); // Left eye.
g.drawOval (110, 75, 30, 20); // Left eye.
g.fillOval (68, 81, 10, 10); // Left pupil.
g.fillOval (121, 81, 10, 10); // Right pupil.
g.drawOval (85, 100, 30, 30); // Nose.
g.fillArc (60, 125, 80, 40, 180, 180); // Mouth.
g.drawOval (25, 92, 15, 30); // Left ear.
g.drawOval (160, 92, 15, 30); // Right ear.
g.drawString ("Burrp!", 180, 165); // Draw a string.
}
}
- Let's create Face2.html as follows
<HTML>
<title>Hello</title>
<BODY>
<applet code="Face2.class" width=300 height=200>
</applet>
<br>
Oh excuse me, I just finished lunch and er .... well, how are
you today?
</body>
</html>
- Link to applet
In-Class Exercise:
Modify your earlier example with the polygon to add
a string both in the applet and in the HTML file.
You can also place multiple applets on the same webpage:
- For example, let's put both Face and
Face2
on the same page:
<HTML>
<title>Hello</title>
<BODY bgcolor="#FFFFFF">
First applet:
<p>
<applet code="Face.class" width=300 height=200>
</applet>
<p>
Second applet:
<p>
<applet code="Face2.class" width=300 height=200>
</applet>
</body>
</html>
- Link to applets